filmov
tv
LeetCode Max Area of Island Solution Explained - Java
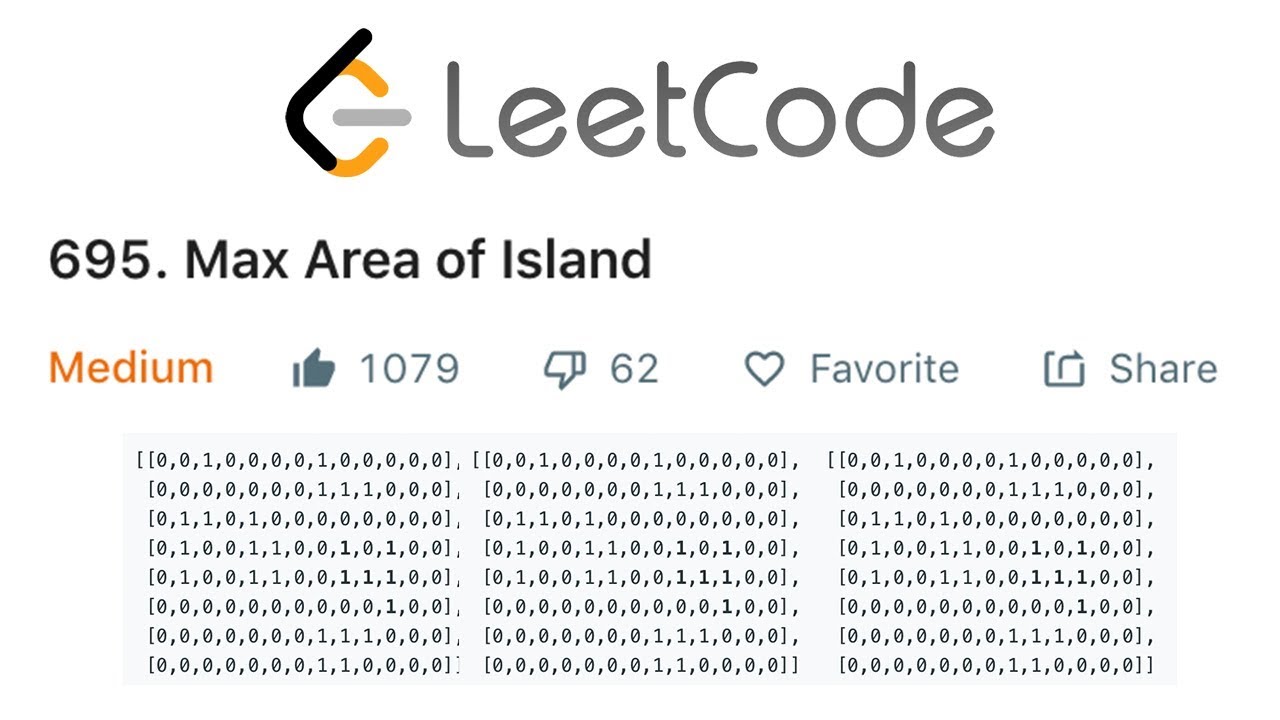
Показать описание
Preparing For Your Coding Interviews? Use These Resources
————————————————————
Other Social Media
----------------------------------------------
Show Support
------------------------------------------------------------------------------
#coding #programming #softwareengineering
Max Area of Island - Leetcode 695 - Python
LeetCode Max Area of Island Solution Explained - Java
Max Area of Island - Leetcode 695
Leetcode - Max Area of Island (Python)
Max Area of Island - Leetcode 695 - Graphs (Python)
Medium Facebook Graph Interview Question - Max Area of Island - Leetcode 695
Max Area of Island - Leetcode 695
Leetcode 695 - Max Area of Island (JAVA Solution Explained!)
NUMBER OF ISLANDS - Leetcode 200 - Python
695. Leetcode Max Area of Island|| Code + Explanation + Example Walkthrough || June1 Daily Challenge
LeetCode 695. Max Area of Island | Python TechZoo
LARGEST RECTANGLE IN HISTOGRAM - Leetcode 84 - Python
Number of Islands - Leetcode 200
Max Area of Island | LeetCode 695 | C++, Python
Leetcode 695: Max Area of Island | Java Solution
Leetcode problem 695 Max area of island solution | Finding the islands using recursion | python
Max Area of Island - LeetCode 695 Python
MAX AREA OF AN ISLAND | PYTHON | LEETCODE # 695
Max Area of Island - LeetCode #695 - C++
Max Area of Island Leet Code Question Explained | Recursive Graph DFS
LeetCode in JavaScript - Max Area of Island
Max Area of Island: LeetCode 695 | Very Common Big-Tech Interview Problem
Coding Interview Tutorial 105 - Max Area of Island [LeetCode]
LeetCode 695 Max Area of Island - Google Coding Interview Question. DFS Java Solution Explained
Комментарии