filmov
tv
MAX AREA OF AN ISLAND | PYTHON | LEETCODE # 695
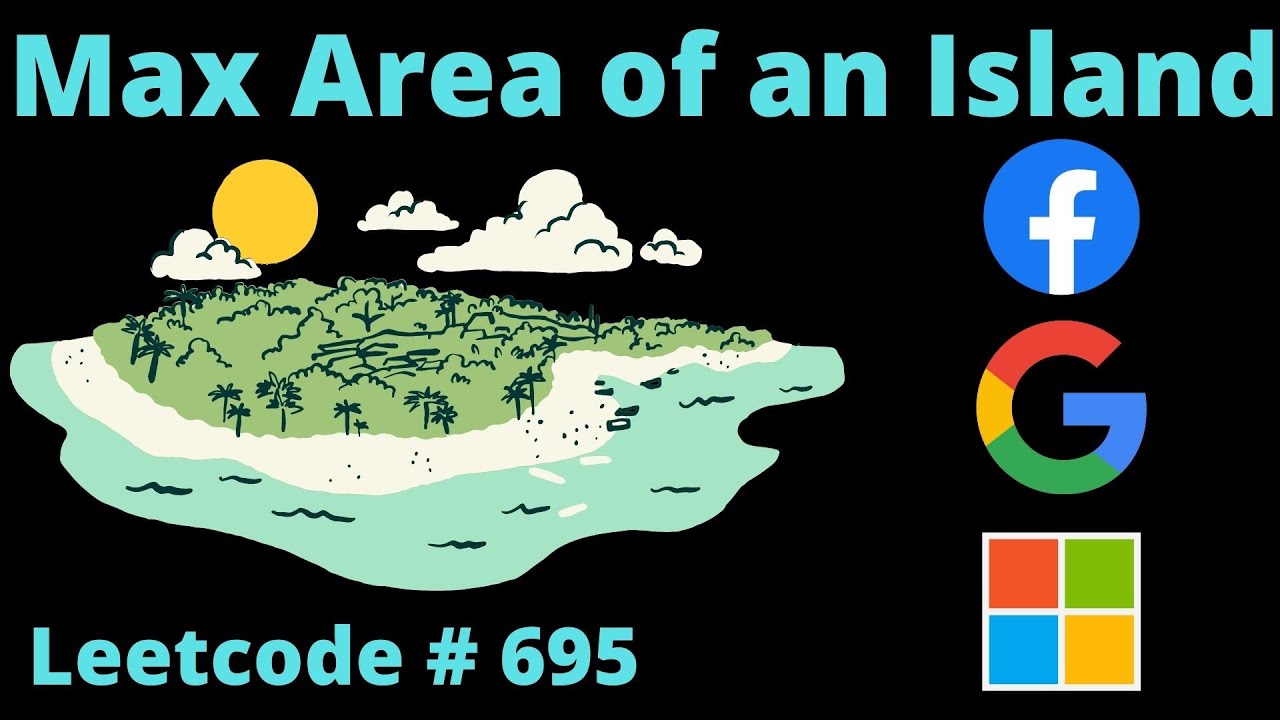
Показать описание
Today's we're going to be solving Leetcode Problem # 695 - Max Area of an Island. This is a relatively straightforward problem but the approach serves as a foundation for a lot of similar problems on Leetcode.
We'll be solving this with a Depth First Search approach and the coding pattern used here is very common so pay attention to the implementation details as you'll encounter many problems similar to this!
We'll be solving this with a Depth First Search approach and the coding pattern used here is very common so pay attention to the implementation details as you'll encounter many problems similar to this!
Max Area of Island - Leetcode 695 - Python
LeetCode Max Area of Island Solution Explained - Java
Leetcode - Max Area of Island (Python)
Max Area of Island - Leetcode 695 - Graphs (Python)
MAX AREA OF AN ISLAND | PYTHON | LEETCODE # 695
Max Area of Island
Max Area of Island - LeetCode 695 Python
695. Leetcode Max Area of Island|| Code + Explanation + Example Walkthrough || June1 Daily Challenge
Leetcode - 695 Max Area of Island
Max Area of Island | LeetCode 695 | C++, Python
Leetcode 695 - Max Area of Island (JAVA Solution Explained!)
Technical Interview Question - Max Area of Island [LeetCode]
Max Area of Island | Leetcode 695 | Matrix Graph BFS DFS | Google Facebook Amazon
Max Area Of Island
Max Area of Island | Leetcode 695 | Live Coding session
Leetcode problem 695 Max area of island solution | Finding the islands using recursion | python
LeetCode in JavaScript - Max Area of Island
LeetCode 695 Max Area of Island - Google Coding Interview Question. DFS Java Solution Explained
GOOGLE Coding Interview Question - Max Area of Island | LeetCode
Max Area of Island | 695 | Python
Coding Interview Tutorial 106 - BFS Max Area of Island [LeetCode]
Leetcode 695 Max Area of Island - Facebook, Google Coding Interview Question
695. Max Area of Island
Find the maximum area of an island | Max area of island | leetcode problem | Depth first search
Комментарии