filmov
tv
String Processing in Python: Check Permutation
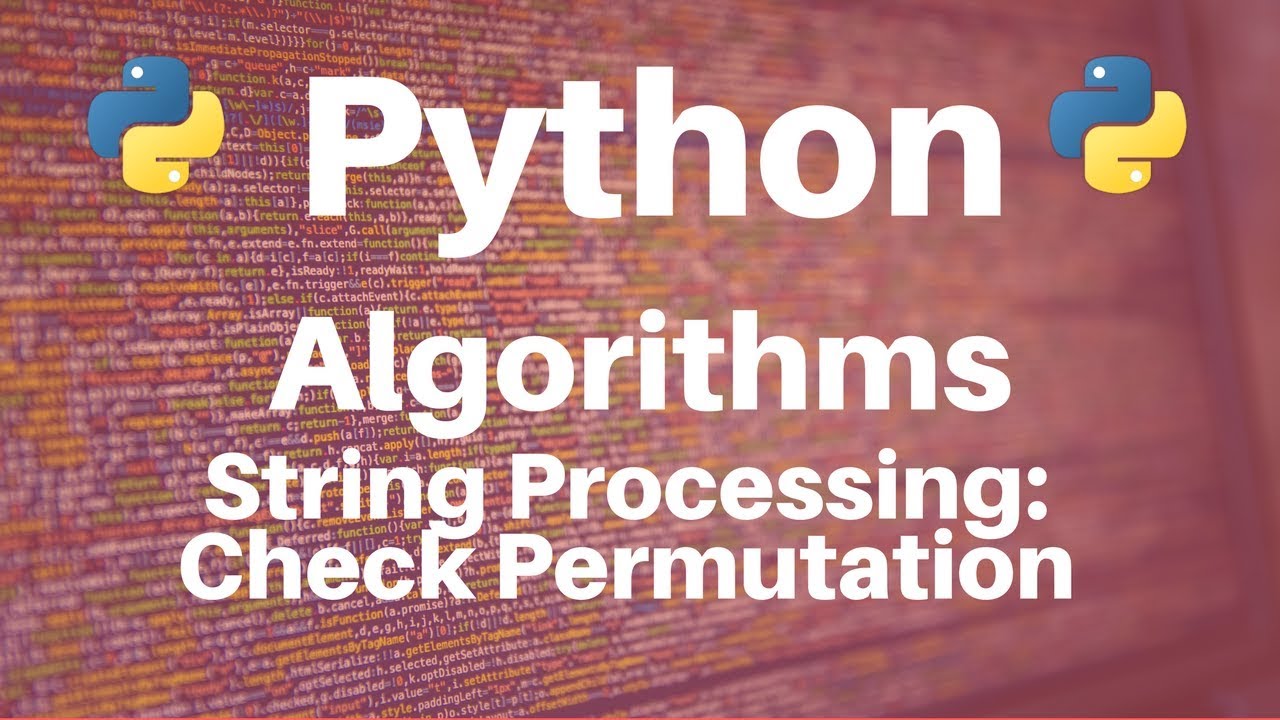
Показать описание
In this video, we will be considering how to determine if a given string is a permutation of another string.
Specifically, we want to solve the problem:
Given two strings, write a function to determine if one is a permutation of the other.
We solve this problem in Python and analyze the time and space complexity of our approach.
This video is part of a series on string processing and, specifically, on how these problems tend to show up in the context of a technical interview:
This video is also part of an "Algorithm" series. For more algorithm tutorials:
The software written in this video is available at:
Do you like the development environment I'm using in this video? It's a customized version of vim that's enhanced for Python development. If you want to see how I set up my vim, I have a series on this here:
If you've found this video helpful and want to stay up-to-date with the latest videos posted on this channel, please subscribe:
Specifically, we want to solve the problem:
Given two strings, write a function to determine if one is a permutation of the other.
We solve this problem in Python and analyze the time and space complexity of our approach.
This video is part of a series on string processing and, specifically, on how these problems tend to show up in the context of a technical interview:
This video is also part of an "Algorithm" series. For more algorithm tutorials:
The software written in this video is available at:
Do you like the development environment I'm using in this video? It's a customized version of vim that's enhanced for Python development. If you want to see how I set up my vim, I have a series on this here:
If you've found this video helpful and want to stay up-to-date with the latest videos posted on this channel, please subscribe:
String Processing in Python: Check Permutation
ALL 47 STRING METHODS IN PYTHON EXPLAINED
To check given string is palindrome or not in Python ( python for beginners )
3. String Manipulation, Guess and Check, Approximations, Bisection
String Processing in Python: Is Unique
I Learned Something New About Boolean Checks In Python
String Processing in Python: Is Palindrome
How to Find Reverse a String in Python ? #shorts
Check variable type in Python
The Python find() String method
Find First And Last Substring Occurrences Using find() rfind() index() rindex() | Python Tutorial
String Processing in Python: Is Palindrome Permutation
Accessing String Characters in Python
Python Program To Check Substring is Present in a Given String | Substring | Python Strings
Frequently Asked Python Program 25:Check for URL in a String
String Processing in Python: Is Anagram
Fuzzy String Matching in Python
MUCH Better Way Of Adding Variables To Strings In Python! #python #coding #programming
Functions in Python are easy 📞
Advanced Python Programming - String Manipulation and Functions
Longest Common Prefix - Leetcode 14 - Python
Python program to check whether a string is palindrome or not #shorts #datascience
Check If A String Is A Palindrome | Python Example
Python Tutorial: String operations
Комментарии