filmov
tv
Longest Common Prefix - Leetcode 14 - Python
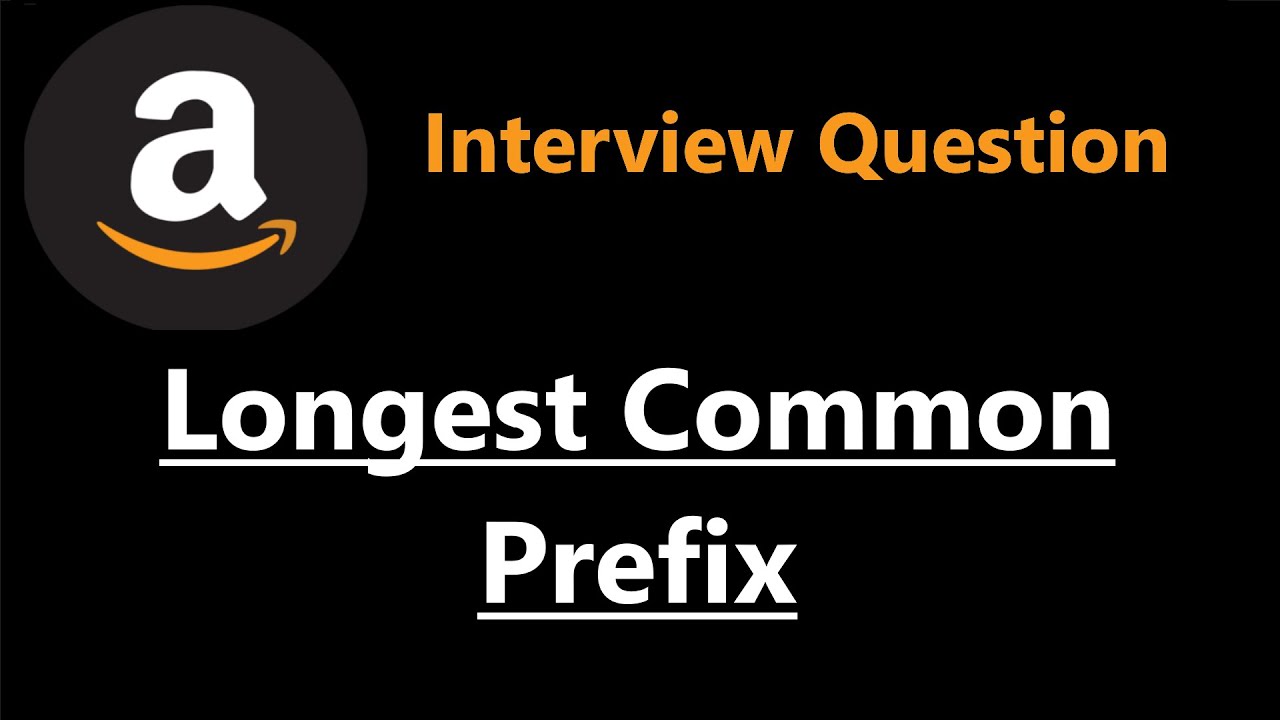
Показать описание
0:00 - Read the problem
1:40 - Drawing Explanation
3:02 - Coding Explanation
leetcode 14
#prefix #interview #python
Disclosure: Some of the links above may be affiliate links, from which I may earn a small commission.
Longest Common Prefix - Leetcode 14 - Python
LeetCode 14. Longest Common Prefix Solution Explained - Java
Longest Common Prefix - Leetcode 14 - Arrays & Strings (Python)
Longest Common Prefix | LeetCode problem 14
Most Asked FAANG Coding Question! | Longest Common Prefix - Leetcode 14
Python Programming Practice: LeetCode #14 -- Longest Common Prefix
Longest Common Prefix (LeetCode 14) | Full solution with animations and examples | Study Algorithms
LeetCode 14 LeetCode Longest Common Prefix in javascript
LeetCode 14 Longest Common Prefix | JavaScript Solution | Top Interview Questions Easy
Longest common prefix
LeetCode 14 | LONGEST COMMON PREFIX | STRINGS | C++ [ Approach and Code Explanation]
How To Solve Algorithms - Longest Common Prefix
LeetCode #14: Longest Common Prefix | Vertical Scanning
05. Longest Common Prefix - leetcode | Java DSA LeetCode Solutions
Leetcode Longest Common Prefix | Python
Lecture 80: Longest Common Prefix Problem || Tries || C++ Placement Series
LeetCode #14 - Longest Common Prefix
Find Longest Common Prefix Solution | LeetCode 14 | Algorithm Explained
Longest Common Prefix
Leetcode 14: Longest Common Prefix | Java Solution
Longest Common Prefix - LeetCode 14 - Coding Interview Questions
14. Longest Common Prefix || Java || Leetcode || Hindi
14. Longest Common Prefix | LeetCode Easy | Python Solution | String, Array
DSA Phir se with Sumeet | Leetcode 14 | Longest Common Prefix
Комментарии