filmov
tv
Find nth Fibonacci number Algorithm in Java with a PRACTICAL EXAMPLE using while loop
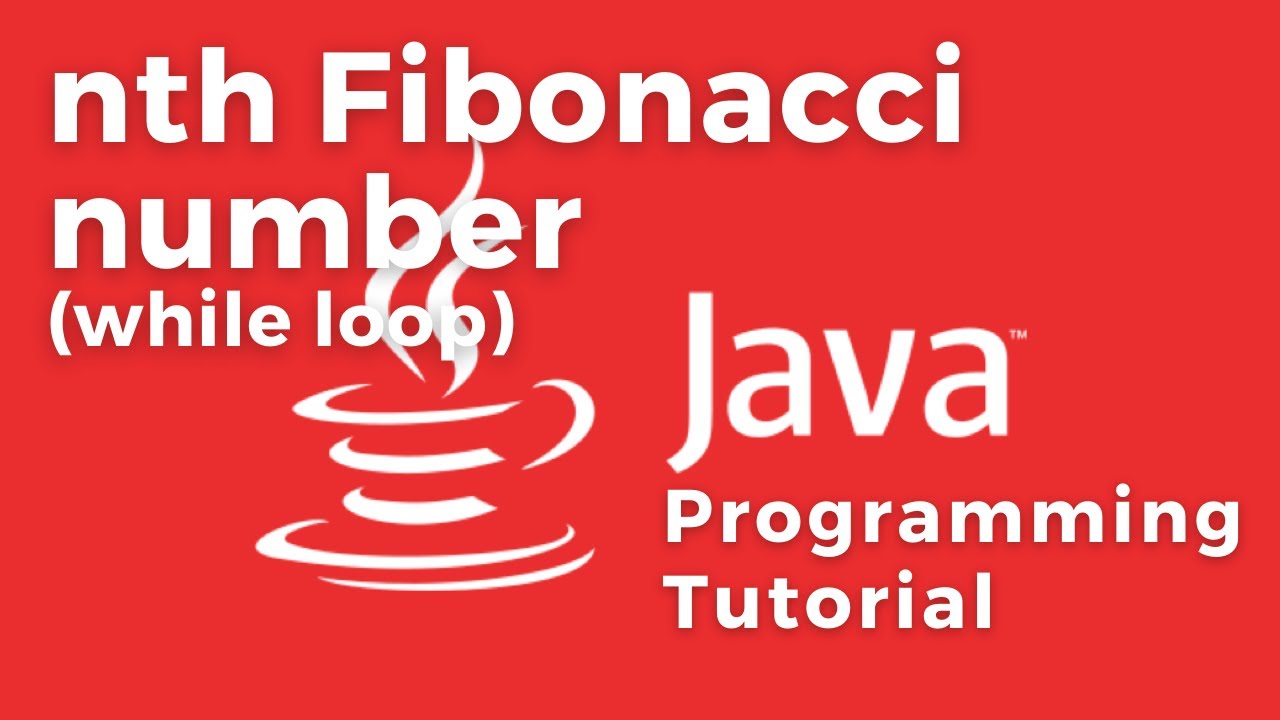
Показать описание
Find nth Fibonacci number Algorithm in Java with a PRACTICAL EXAMPLE using while loop
In this video, we are going to write a program that uses a while loop to find a Fibonacci number by specifying its position in the Fibonacci sequence
In Mathematics, Fibonacci numbers form a sequence of numbers called the Fibonacci sequence
By definition, the Fibonacci sequence is a series of numbers where each number is the addition of the last two preceding numbers starting with 0 and 1
Here on the screen is an example of that sequence
0, 1, 1, 2, 3, 5, 8, 13, 21, 34, ....
In this sequence, for example, you can notice that 34 is the result of the addition between the preceding numbers which are 21 and 13
Or 21 is the result of the addition between 13 and 8
13 is the result of the addition between 5 and 8
8 is the result of the addition between 3 and 5
From the sequence on the screen
You can determine the next Fibonacci number by applying the same logic
Which is to add up 21 and 34 in order to get 55
As I said at the beginning, in this video we are going to develop a program that will allow us to find the Fibonacci number that occupies a specific position in the sequence
The program must allow the user to input the position of the desired Fibonacci number in the Fibonacci sequence
That simply means that we need to get the first two Fibonacci numbers, the position of the desired number in the Fibonacci sequence
Then calculate the next Fibonacci number by adding the previous two elements of the Fibonacci sequence
And repeat this step until the desired Fibonacci number is found
Then print out that Fibonacci number
Here is how the code will look like
First, let’s declare the input object like this
Second, let’s declare the various variables used in the program
The first variables will be the ones to store the first two numbers of the Fibonacci sequence
int number1 = 0
Int number2 = 1;
The next variable will be the one that stores the desired Fibonacci number
int fibonacci_number;
I will need another variable that will store the position of the Fibonacci number we are looking for
int number_position;
Then, the other variable will be the loop control variable
int counter;
Now, let’s write the output statements
The first output statement will tell the user about the first and second Fibonacci number of the sequence
The second statement will prompt the user to enter the position of the desired Fibonacci number
Right after that, let’s add an input statement to allow the user to enter the position
We have now reached the main part of our program
Which is to determine how the program will find the desired Fibonacci number
Let’s start by writing an if … else selection control structure
In which the Fibonacci number position entered by the user will be used in the condition
if (number_position == 1) {
fibonacci_number = number1;
}
else if (number_position == 2) {
fibonacci_number = number2;
}
else {
Else if the position entered by the user is actually greater than 2 then
We will initialize the counter variable and set it to 3
counter = 3;
After we need a while loop
And in the condition of the while loop we will test and compare the variable counter and the variable number_position
while ( counter less than or equal number_position ) {
In the body of the loop here are the action statements that need to execute
fibonacci_number = number 2 + number1;
This is because the Fibonacci number is the addition between the last two preceding numbers of the sequence
Also, in order to make sure that the Fibonacci number sequence will continue, we need to add these other statements
number1 = number2;
number2 = fibonacci_number;
counter++;
}
}
Outside of the while loop and the if statement
Let’s write the output statement that will print out the desired Fibonacci number
}
}
Once everything is set up correctly
You can run the program and see what happens
Thanks for viewing, I hope this video was informative.
Please do not forget to like and SUBSCRIBE to this channel.
Let’s meet in the next video.
#codingriver
#fibonaccialgorithm
#learnjava
In this video, we are going to write a program that uses a while loop to find a Fibonacci number by specifying its position in the Fibonacci sequence
In Mathematics, Fibonacci numbers form a sequence of numbers called the Fibonacci sequence
By definition, the Fibonacci sequence is a series of numbers where each number is the addition of the last two preceding numbers starting with 0 and 1
Here on the screen is an example of that sequence
0, 1, 1, 2, 3, 5, 8, 13, 21, 34, ....
In this sequence, for example, you can notice that 34 is the result of the addition between the preceding numbers which are 21 and 13
Or 21 is the result of the addition between 13 and 8
13 is the result of the addition between 5 and 8
8 is the result of the addition between 3 and 5
From the sequence on the screen
You can determine the next Fibonacci number by applying the same logic
Which is to add up 21 and 34 in order to get 55
As I said at the beginning, in this video we are going to develop a program that will allow us to find the Fibonacci number that occupies a specific position in the sequence
The program must allow the user to input the position of the desired Fibonacci number in the Fibonacci sequence
That simply means that we need to get the first two Fibonacci numbers, the position of the desired number in the Fibonacci sequence
Then calculate the next Fibonacci number by adding the previous two elements of the Fibonacci sequence
And repeat this step until the desired Fibonacci number is found
Then print out that Fibonacci number
Here is how the code will look like
First, let’s declare the input object like this
Second, let’s declare the various variables used in the program
The first variables will be the ones to store the first two numbers of the Fibonacci sequence
int number1 = 0
Int number2 = 1;
The next variable will be the one that stores the desired Fibonacci number
int fibonacci_number;
I will need another variable that will store the position of the Fibonacci number we are looking for
int number_position;
Then, the other variable will be the loop control variable
int counter;
Now, let’s write the output statements
The first output statement will tell the user about the first and second Fibonacci number of the sequence
The second statement will prompt the user to enter the position of the desired Fibonacci number
Right after that, let’s add an input statement to allow the user to enter the position
We have now reached the main part of our program
Which is to determine how the program will find the desired Fibonacci number
Let’s start by writing an if … else selection control structure
In which the Fibonacci number position entered by the user will be used in the condition
if (number_position == 1) {
fibonacci_number = number1;
}
else if (number_position == 2) {
fibonacci_number = number2;
}
else {
Else if the position entered by the user is actually greater than 2 then
We will initialize the counter variable and set it to 3
counter = 3;
After we need a while loop
And in the condition of the while loop we will test and compare the variable counter and the variable number_position
while ( counter less than or equal number_position ) {
In the body of the loop here are the action statements that need to execute
fibonacci_number = number 2 + number1;
This is because the Fibonacci number is the addition between the last two preceding numbers of the sequence
Also, in order to make sure that the Fibonacci number sequence will continue, we need to add these other statements
number1 = number2;
number2 = fibonacci_number;
counter++;
}
}
Outside of the while loop and the if statement
Let’s write the output statement that will print out the desired Fibonacci number
}
}
Once everything is set up correctly
You can run the program and see what happens
Thanks for viewing, I hope this video was informative.
Please do not forget to like and SUBSCRIBE to this channel.
Let’s meet in the next video.
#codingriver
#fibonaccialgorithm
#learnjava