filmov
tv
Leetcode - Design Circular Queue (Python)
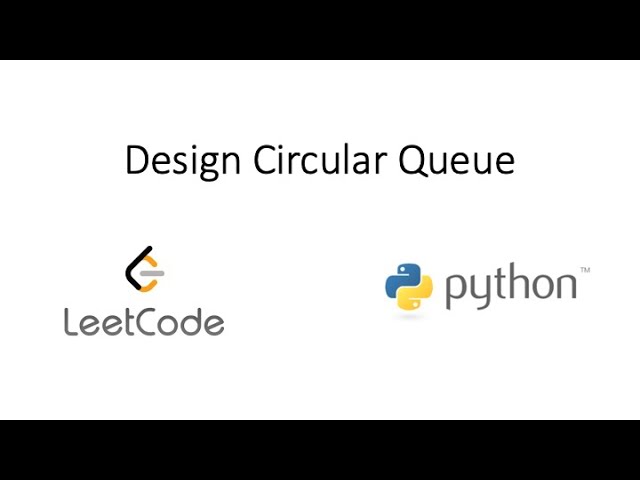
Показать описание
April 2021 Leetcode Challenge
Leetcode - Design Circular Queue #622
Difficulty: Medium
Leetcode - Design Circular Queue #622
Difficulty: Medium
Design Circular Queue | Live Coding with Explanation | Leetcode - 622
Leetcode - Design Circular Queue (Python)
Design Circular Queue - Leetcode 622 - Python
622. Design Circular Queue
Leetcode 622. Design Circular Queue - Python
Leetcode 622 (Design Circular Queue)
622. Design Circular Queue - Day 25/30 Leetcode September Challenge
Design Circular Queue | Leetcode 622 | LC Medium | Live coding session | April 4 | LEETCODE EXPLORE
LeetCode - Design Circular Queue
Leetcode Design Circular Queue | April Leetcode Challenge 2021
Design Circular Queue - Leetcode 622 - Linked List - Python
Amazon Coding Interview Question | Leetcode 622 | Design Circular Queue
Coding Interview Tutorial 136 - Design Circular Queue [LeetCode]
622. Design Circular Queue - Day 4/30 Leetcode April Challenge
Daily LeetCode Challenge [28]: 622. Design Circular Queue. (#queue #array)
LeetCode.622. Design Circular Queue - Java - Day 21
622 Design Circular Queue
LeetCode 622 - Design Circular Queue | Решение с объяснением - Swift
Leetcode 622 Design Circular Queue
LeetCode 622 Design Circular Queue
Design Circular Queue | LeetCode 622 | Circular Queue Implementation | @LearnOverflow
Design Circular Queue | April Leetcode Challenge
Design Circular Deque | Leetcode 641
622 Design Circular Queue | Leetcode | Java | Hindi Using Array
Комментарии