filmov
tv
Coding Interview Tutorial 136 - Design Circular Queue [LeetCode]
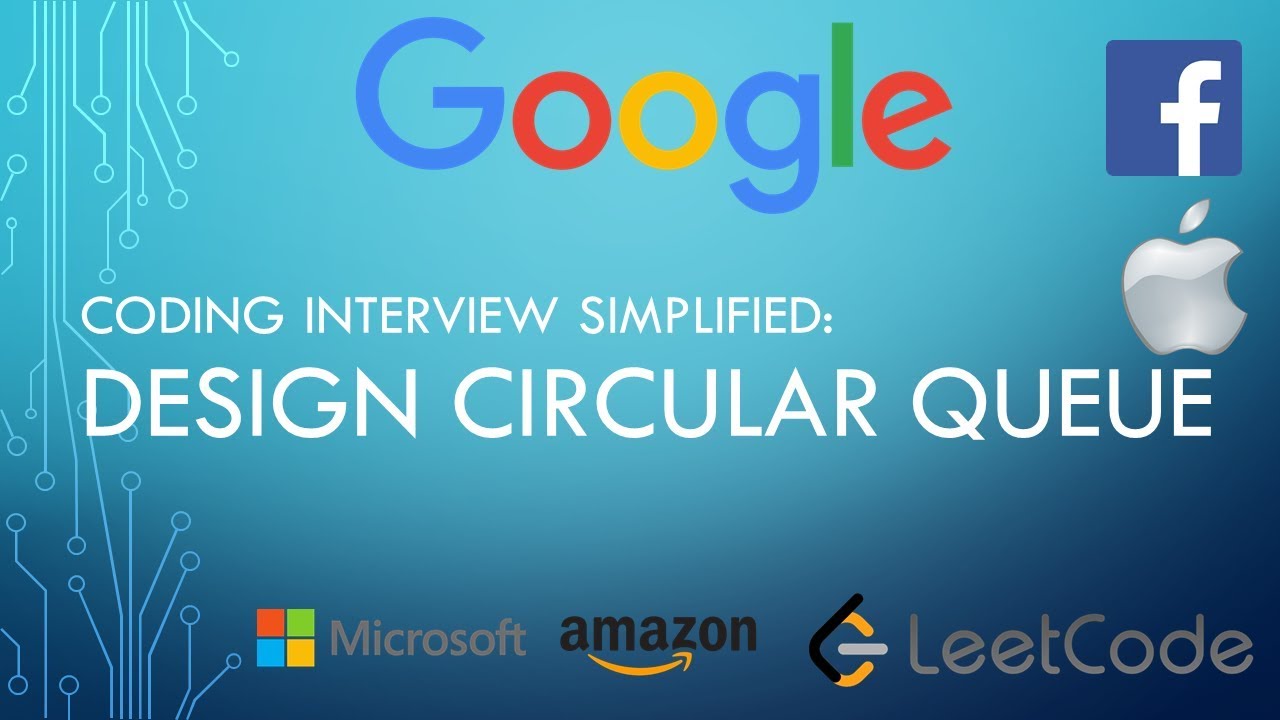
Показать описание
Learn how to design a circular queue easily and efficiently!
Algorithms, data structures, and coding interviews simplified!
This is an important programming interview problem, and we use the LeetCode platform to solve it.
Algorithms, data structures, and coding interviews simplified!
This is an important programming interview problem, and we use the LeetCode platform to solve it.
Coding Interview Tutorial 136 - Design Circular Queue [LeetCode]
Single Number | LeetCode 136 | Amazon Coding Interview Tutorial
Single Number | LeetCode 136 | Coding Interview Question
BRUTE FORCE DEVELOPER vs Optimal Toronto Engineer on Single Number, Leetcode 136
What is a defensive copy? - Cracking the Java Coding Interview
Single Number 1 - HashMap Solution || Leetcode 136 || Interview Questions - Amazon, Facebook , Apple
Applying bitwise XOR, LC #136 Single Number[Tech Interview Live Coding]
LeetCode 136: Single Number (SOLVED) | Facebook Coding Interview Question
Single Number - Leetcode 136 - Python
Coding Interview Tutorial 147 - Triangle [LeetCode]
LeetCode 136: Single Number - Interview Prep Ep 35
136. Single Number Leetcode Problem | Solution with Explanation | DSA Problem Series
Single Number | LeetCode#136 | Java | Technical Interview
Single Number 1-Bit Manipulation (Solution-2) | Leetcode -136 || Interview Questions-Amazon,FB,Apple
Solving LeetCode 136 in JavaScript (Single Number)
Question 136 | Linux Interview Questions
Single Number: 136 - google interview question
136/200. JavaScript interview questions #softwareengineer #coding #javascript #developer #code
LeetCode 704. Binary Search - Interview Prep Ep 136
LeetCode 136 Single Number | JavaScript Solution | Top Interview Questions Easy
136. Single Number | Interview Preparation | English | code io
That got a little 🥵👀 #shorts
Microsoft Loves This Coding Question! | Reverse Words in a String - Leetcode 151
Single Number - LeetCode 136 - Blind 75 - Bit Manipulation
Комментарии