filmov
tv
How to Group a Nested Array in TypeScript
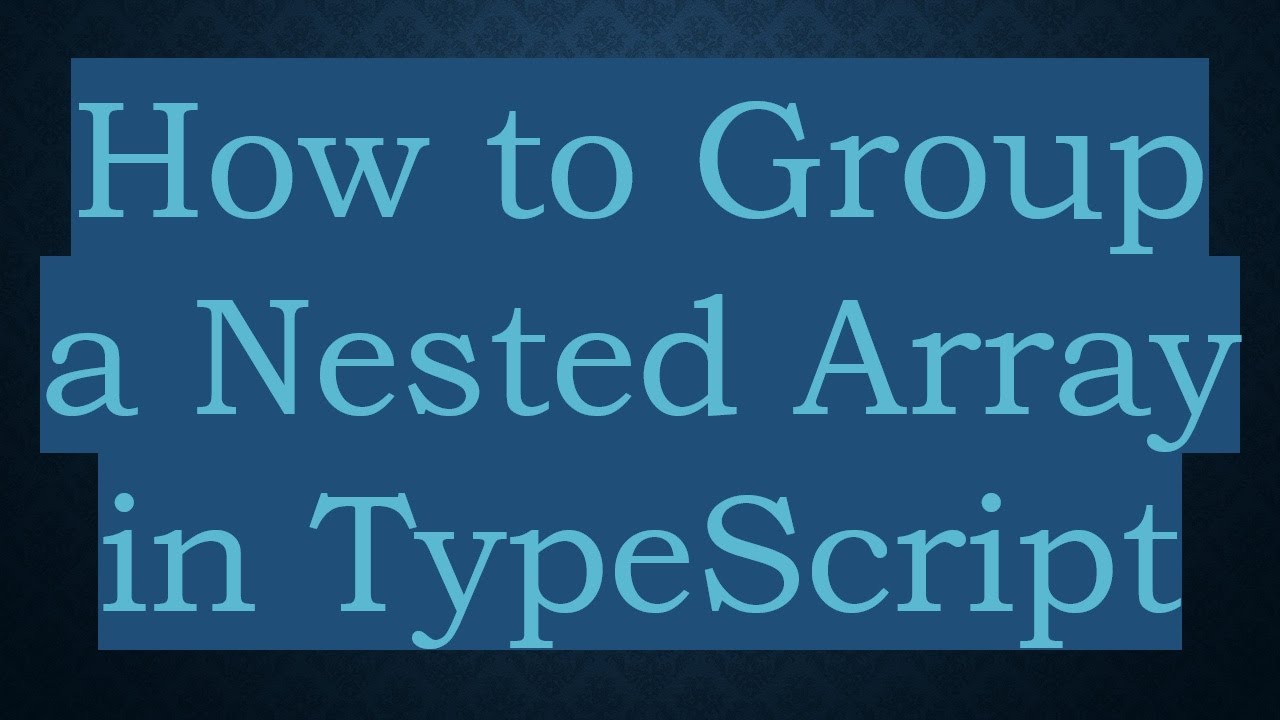
Показать описание
Learn how to effectively group nested arrays by properties in TypeScript with a practical example for quizzes.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Typescript - How to group a nested array
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Group a Nested Array in TypeScript
Working with nested arrays can often lead to challenges, especially when you want to organize or group data for easier access. If you’re dealing with data from an API that returns user scores associated with quizzes, grouping these scores by a specific property, such as quiz_title, can be an essential step in managing the data effectively.
In this guide, we’ll dive into the problem of grouping a nested array in TypeScript and provide a clear solution with examples. Let’s break it down!
Understanding the Problem
You might encounter a scenario where you receive data in a structured format like the following. Here’s an example structure of the UserScore array that includes user data and their associated quiz information:
[[See Video to Reveal this Text or Code Snippet]]
You want to group the entries by their quiz_title so that all scores for each quiz are organized together.
Approach to Solution
Using Array Reduce Method
In TypeScript, one way to group entries in an array is by using the reduce method. This method allows you to iterate over the array and accumulate results based on the condition you define.
Here’s how to implement it:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Code
Input Array: Start with the UserScore array which contains user scores with associated quiz information.
Reduce Method:
The reduce method takes a callback function and an initial accumulator object ({}).
For each current entry (curr), it checks:
If an array for the quiz_title already exists. If it does, it spreads the existing array and adds the current item.
If not, it initializes a new array containing the current score entry.
Result: You will get an object where each key corresponds to the quiz_title, and the value is an array of scores for that quiz.
Conclusion
By using the above technique, you can efficiently group nested arrays in TypeScript, making your data much easier to handle and visualize. This skill not only helps you organize data but also enhances your ability to manipulate it swiftly in TypeScript applications.
Feel free to experiment with this method on similar structures, and let your creativity flow in data management!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Typescript - How to group a nested array
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Group a Nested Array in TypeScript
Working with nested arrays can often lead to challenges, especially when you want to organize or group data for easier access. If you’re dealing with data from an API that returns user scores associated with quizzes, grouping these scores by a specific property, such as quiz_title, can be an essential step in managing the data effectively.
In this guide, we’ll dive into the problem of grouping a nested array in TypeScript and provide a clear solution with examples. Let’s break it down!
Understanding the Problem
You might encounter a scenario where you receive data in a structured format like the following. Here’s an example structure of the UserScore array that includes user data and their associated quiz information:
[[See Video to Reveal this Text or Code Snippet]]
You want to group the entries by their quiz_title so that all scores for each quiz are organized together.
Approach to Solution
Using Array Reduce Method
In TypeScript, one way to group entries in an array is by using the reduce method. This method allows you to iterate over the array and accumulate results based on the condition you define.
Here’s how to implement it:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Code
Input Array: Start with the UserScore array which contains user scores with associated quiz information.
Reduce Method:
The reduce method takes a callback function and an initial accumulator object ({}).
For each current entry (curr), it checks:
If an array for the quiz_title already exists. If it does, it spreads the existing array and adds the current item.
If not, it initializes a new array containing the current score entry.
Result: You will get an object where each key corresponds to the quiz_title, and the value is an array of scores for that quiz.
Conclusion
By using the above technique, you can efficiently group nested arrays in TypeScript, making your data much easier to handle and visualize. This skill not only helps you organize data but also enhances your ability to manipulate it swiftly in TypeScript applications.
Feel free to experiment with this method on similar structures, and let your creativity flow in data management!