filmov
tv
How to Find the First Element of an Array Matching a Boolean Condition in JavaScript
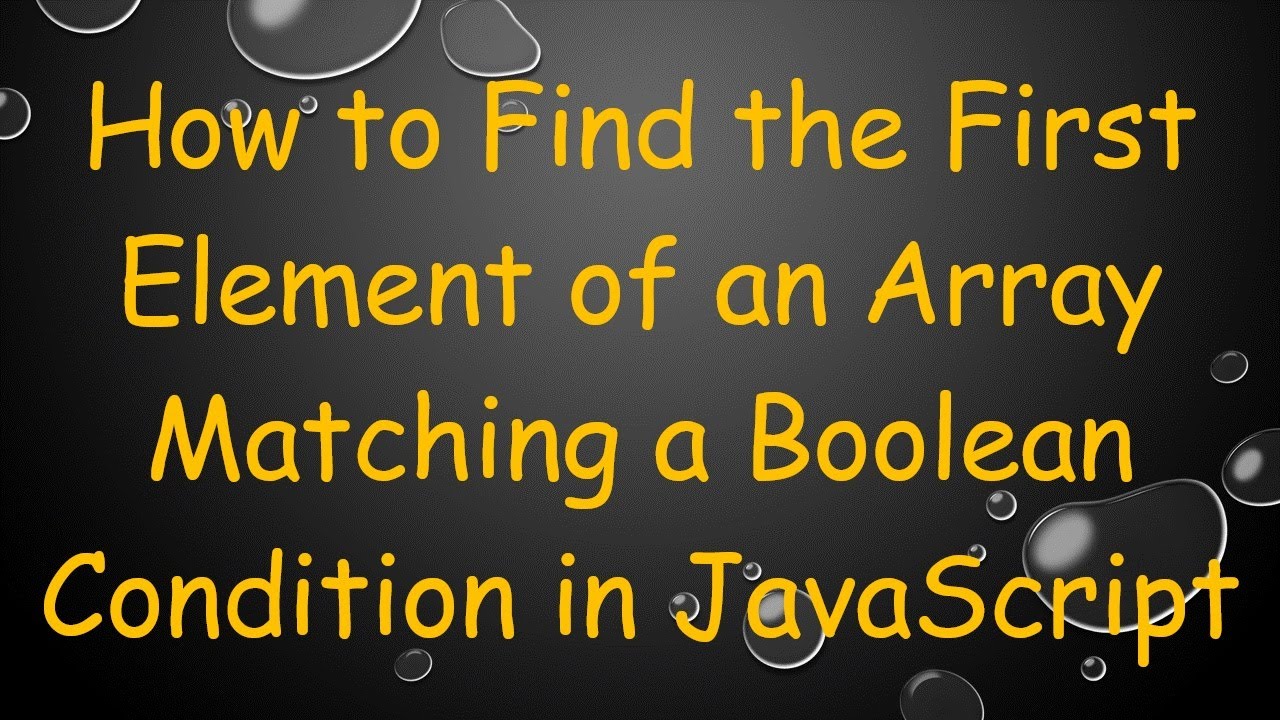
Показать описание
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Summary: Learn how to efficiently find the first element of an array that meets a specific boolean condition in JavaScript using various methods including the `find` method.
---
In JavaScript, finding the first element in an array that matches a specific boolean condition is a common task. This can be accomplished using various methods, each suited for different situations. Here, we'll explore the most effective and straightforward approaches to achieve this.
Using the find Method
The find method is one of the most intuitive and readable ways to find the first element that satisfies a given condition. It takes a callback function that tests each element of the array, returning the first element that matches the condition.
[[See Video to Reveal this Text or Code Snippet]]
In this example, isEven is a function that returns true if the element is even. The find method goes through the array and returns the first even number.
Using a for Loop
For situations where you need more control or need to perform additional operations while finding the element, a for loop is a good alternative.
[[See Video to Reveal this Text or Code Snippet]]
This loop iterates through the array and breaks out as soon as it finds the first even number, assigning it to firstEven.
Using the filter Method (Not Recommended for Performance)
Another method is to use the filter method combined with accessing the first element of the filtered array. This is generally less efficient because it processes the entire array even if the first match is found early.
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Choosing the right method depends on your specific needs and the context of your code. The find method is typically the most concise and readable for most use cases. A for loop provides more control and can be more efficient for certain scenarios. The filter method, while useful in some cases, is generally less efficient for finding a single element.
By understanding and applying these methods, you can efficiently find the first element in an array that matches a boolean condition in JavaScript, enhancing both the readability and performance of your code.
---
Summary: Learn how to efficiently find the first element of an array that meets a specific boolean condition in JavaScript using various methods including the `find` method.
---
In JavaScript, finding the first element in an array that matches a specific boolean condition is a common task. This can be accomplished using various methods, each suited for different situations. Here, we'll explore the most effective and straightforward approaches to achieve this.
Using the find Method
The find method is one of the most intuitive and readable ways to find the first element that satisfies a given condition. It takes a callback function that tests each element of the array, returning the first element that matches the condition.
[[See Video to Reveal this Text or Code Snippet]]
In this example, isEven is a function that returns true if the element is even. The find method goes through the array and returns the first even number.
Using a for Loop
For situations where you need more control or need to perform additional operations while finding the element, a for loop is a good alternative.
[[See Video to Reveal this Text or Code Snippet]]
This loop iterates through the array and breaks out as soon as it finds the first even number, assigning it to firstEven.
Using the filter Method (Not Recommended for Performance)
Another method is to use the filter method combined with accessing the first element of the filtered array. This is generally less efficient because it processes the entire array even if the first match is found early.
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Choosing the right method depends on your specific needs and the context of your code. The find method is typically the most concise and readable for most use cases. A for loop provides more control and can be more efficient for certain scenarios. The filter method, while useful in some cases, is generally less efficient for finding a single element.
By understanding and applying these methods, you can efficiently find the first element in an array that matches a boolean condition in JavaScript, enhancing both the readability and performance of your code.