filmov
tv
Mastering Array Initialization and Declaration in C
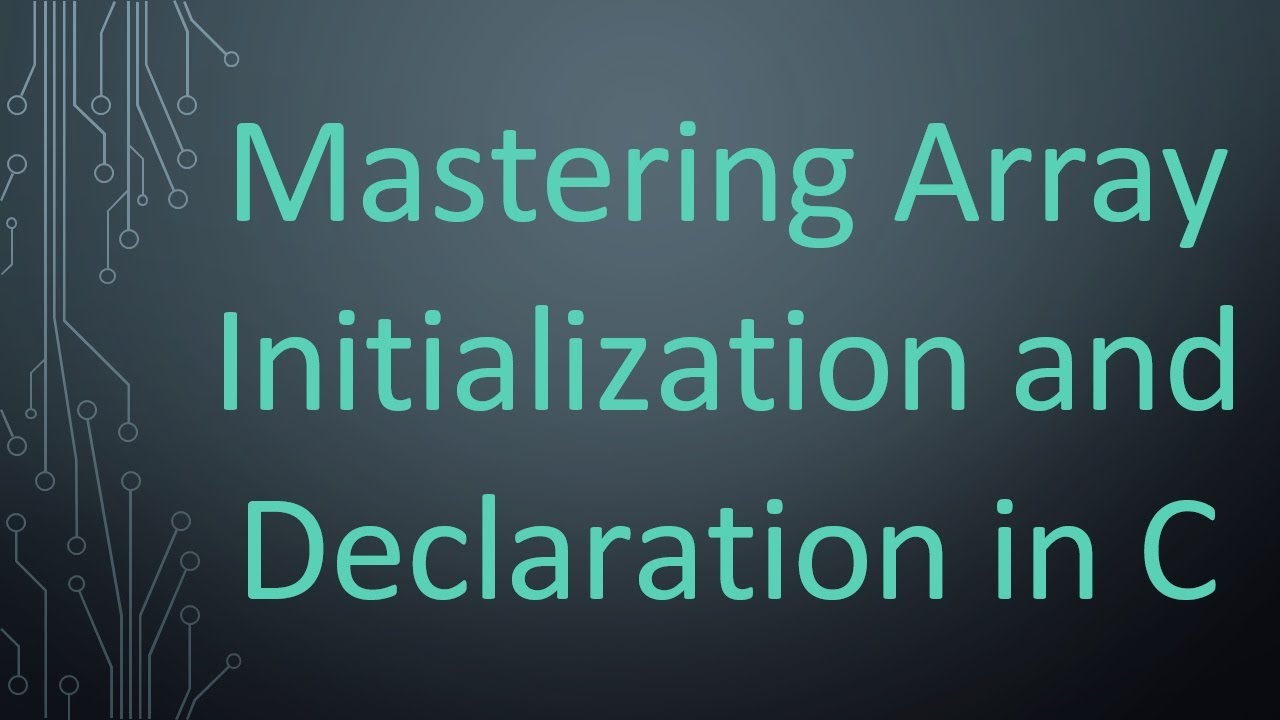
Показать описание
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Summary: Discover how to declare and initialize arrays in C, including 1D, 2D, and multidimensional arrays, with practical examples. Learn the advantages of initializing arrays at the time of declaration.
---
Mastering Array Initialization and Declaration in C
In C programming, arrays are crucial for handling collections of data. Understanding how to declare and initialize arrays effectively can help you write more efficient and readable code. Let's delve into the different techniques for dealing with arrays in C, focusing on practical examples and the advantages of early initialization.
Declaring and Initializing 1D Arrays
A one-dimensional (1D) array in C is a simple list of elements of the same type. Here's how you can declare and initialize a 1D array:
Declaration
[[See Video to Reveal this Text or Code Snippet]]
This example declares an array named numbers that can hold five integers.
Initialization at Declaration
[[See Video to Reveal this Text or Code Snippet]]
Here, the array numbers is initialized with five integers at the time of declaration. This is a concise way to set initial values and can prevent potential errors.
Examples
Example of Declaring and Initializing a 1D Array
[[See Video to Reveal this Text or Code Snippet]]
Declaring and Initializing 2D Arrays
A two-dimensional (2D) array in C can be thought of as a table with rows and columns.
Declaration
[[See Video to Reveal this Text or Code Snippet]]
This declares a 3x3 array named matrix.
Initialization at Declaration
[[See Video to Reveal this Text or Code Snippet]]
This initializes the matrix array with predefined values.
Examples
Example of Declaring and Initializing a 2D Array
[[See Video to Reveal this Text or Code Snippet]]
Declaring and Initializing Multidimensional Arrays
For applications requiring more complexity, multidimensional arrays (arrays of arrays) can be used.
Declaration
[[See Video to Reveal this Text or Code Snippet]]
This declares a 3D array that can be visualized as two tables of 3x4 elements.
Initialization at Declaration
[[See Video to Reveal this Text or Code Snippet]]
This initializes a 3D array with specific values for each element.
Examples
Example of Declaring and Initializing a Multidimensional Array
[[See Video to Reveal this Text or Code Snippet]]
Advantages of Initializing Arrays at Declaration
Simplicity: Initializing arrays at the time of declaration saves lines of code and makes the program easier to read and maintain.
Avoids Garbage Values: Arrays in C are not automatically initialized; uninitialized elements can contain garbage values. Initialization at the time of declaration ensures that all elements have known values.
Improves Safety: Initial assignment prevents accidental usage of uninitialized variables, reducing the chance of bugs and errors.
Mastering the techniques of declaring and initializing arrays in C enables you to manage data collections more effectively, ensuring clearer, more reliable code.
---
Summary: Discover how to declare and initialize arrays in C, including 1D, 2D, and multidimensional arrays, with practical examples. Learn the advantages of initializing arrays at the time of declaration.
---
Mastering Array Initialization and Declaration in C
In C programming, arrays are crucial for handling collections of data. Understanding how to declare and initialize arrays effectively can help you write more efficient and readable code. Let's delve into the different techniques for dealing with arrays in C, focusing on practical examples and the advantages of early initialization.
Declaring and Initializing 1D Arrays
A one-dimensional (1D) array in C is a simple list of elements of the same type. Here's how you can declare and initialize a 1D array:
Declaration
[[See Video to Reveal this Text or Code Snippet]]
This example declares an array named numbers that can hold five integers.
Initialization at Declaration
[[See Video to Reveal this Text or Code Snippet]]
Here, the array numbers is initialized with five integers at the time of declaration. This is a concise way to set initial values and can prevent potential errors.
Examples
Example of Declaring and Initializing a 1D Array
[[See Video to Reveal this Text or Code Snippet]]
Declaring and Initializing 2D Arrays
A two-dimensional (2D) array in C can be thought of as a table with rows and columns.
Declaration
[[See Video to Reveal this Text or Code Snippet]]
This declares a 3x3 array named matrix.
Initialization at Declaration
[[See Video to Reveal this Text or Code Snippet]]
This initializes the matrix array with predefined values.
Examples
Example of Declaring and Initializing a 2D Array
[[See Video to Reveal this Text or Code Snippet]]
Declaring and Initializing Multidimensional Arrays
For applications requiring more complexity, multidimensional arrays (arrays of arrays) can be used.
Declaration
[[See Video to Reveal this Text or Code Snippet]]
This declares a 3D array that can be visualized as two tables of 3x4 elements.
Initialization at Declaration
[[See Video to Reveal this Text or Code Snippet]]
This initializes a 3D array with specific values for each element.
Examples
Example of Declaring and Initializing a Multidimensional Array
[[See Video to Reveal this Text or Code Snippet]]
Advantages of Initializing Arrays at Declaration
Simplicity: Initializing arrays at the time of declaration saves lines of code and makes the program easier to read and maintain.
Avoids Garbage Values: Arrays in C are not automatically initialized; uninitialized elements can contain garbage values. Initialization at the time of declaration ensures that all elements have known values.
Improves Safety: Initial assignment prevents accidental usage of uninitialized variables, reducing the chance of bugs and errors.
Mastering the techniques of declaring and initializing arrays in C enables you to manage data collections more effectively, ensuring clearer, more reliable code.