filmov
tv
How to Set an Object Key by Variable in JavaScript
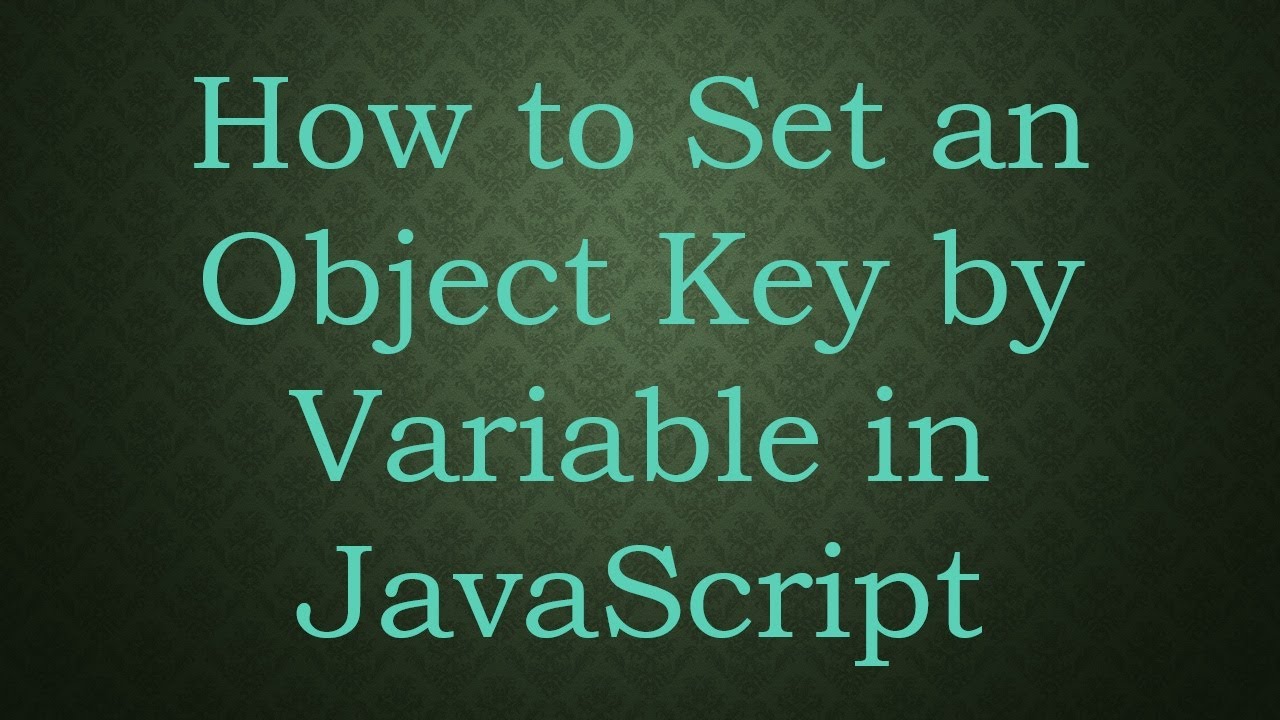
Показать описание
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Summary: Discover the process of setting an object key dynamically using variables in JavaScript with this comprehensive guide.
---
How to Set an Object Key by Variable in JavaScript
When working with JavaScript objects, there are scenarios where you might want to set a key dynamically using a variable. This task can be particularly useful when you're handling dynamic data structures or need to accommodate variable property names. Here's a detailed guide on how to achieve this.
The Traditional Way
Traditionally, when adding a key-value pair to a JavaScript object, you use dot notation or bracket notation. Here’s a recap:
[[See Video to Reveal this Text or Code Snippet]]
In both examples, the key names are hard-coded and fixed. But what if the key name is stored in a variable?
Using Variables as Keys
To dynamically set a key name using a variable, you would use bracket notation. Here’s how you can do it:
[[See Video to Reveal this Text or Code Snippet]]
In this instance, dynamicKey holds the key name as a string. By using the bracket notation, you can set variableKey in obj to have the value "value".
Dynamic Keys in Function Parameters
Consider a function that takes an object and a key-value pair, and assigns the value to the key dynamically. The function could look something like this:
[[See Video to Reveal this Text or Code Snippet]]
This function will add the key-value pair to the object obj based on the key provided.
Using ES6 Computed Property Names
With the advent of ES6, JavaScript introduced computed property names, which make the syntax even cleaner when dealing with dynamic keys. Here’s how you can use them:
[[See Video to Reveal this Text or Code Snippet]]
In this example, the value of dynamicKey is directly used to create a property in obj through the use of square brackets within the object literal.
Use Cases
Dynamic Form Handling: Consider a scenario where your application needs to handle form inputs dynamically. By assigning form input names as object keys, you can manage form states efficiently.
API Responses: When dealing with unpredictable API responses, certain responses might include fields that are not predefined in your application. Using variables as keys helps to dynamically accommodate these responses.
Localization: For applications supporting multiple languages, setting object keys based on locale identifiers can be streamlined using this approach.
Conclusion
Setting an object key by variable in JavaScript is essential for situations where you deal with dynamic or unpredictable property names. Whether you're using the classic bracket notation or leveraging ES6 computed property names, this technique will greatly enhance your ability to handle dynamic data structures efficiently. By understanding and implementing these methods, you can write more flexible and maintainable JavaScript code.
---
Summary: Discover the process of setting an object key dynamically using variables in JavaScript with this comprehensive guide.
---
How to Set an Object Key by Variable in JavaScript
When working with JavaScript objects, there are scenarios where you might want to set a key dynamically using a variable. This task can be particularly useful when you're handling dynamic data structures or need to accommodate variable property names. Here's a detailed guide on how to achieve this.
The Traditional Way
Traditionally, when adding a key-value pair to a JavaScript object, you use dot notation or bracket notation. Here’s a recap:
[[See Video to Reveal this Text or Code Snippet]]
In both examples, the key names are hard-coded and fixed. But what if the key name is stored in a variable?
Using Variables as Keys
To dynamically set a key name using a variable, you would use bracket notation. Here’s how you can do it:
[[See Video to Reveal this Text or Code Snippet]]
In this instance, dynamicKey holds the key name as a string. By using the bracket notation, you can set variableKey in obj to have the value "value".
Dynamic Keys in Function Parameters
Consider a function that takes an object and a key-value pair, and assigns the value to the key dynamically. The function could look something like this:
[[See Video to Reveal this Text or Code Snippet]]
This function will add the key-value pair to the object obj based on the key provided.
Using ES6 Computed Property Names
With the advent of ES6, JavaScript introduced computed property names, which make the syntax even cleaner when dealing with dynamic keys. Here’s how you can use them:
[[See Video to Reveal this Text or Code Snippet]]
In this example, the value of dynamicKey is directly used to create a property in obj through the use of square brackets within the object literal.
Use Cases
Dynamic Form Handling: Consider a scenario where your application needs to handle form inputs dynamically. By assigning form input names as object keys, you can manage form states efficiently.
API Responses: When dealing with unpredictable API responses, certain responses might include fields that are not predefined in your application. Using variables as keys helps to dynamically accommodate these responses.
Localization: For applications supporting multiple languages, setting object keys based on locale identifiers can be streamlined using this approach.
Conclusion
Setting an object key by variable in JavaScript is essential for situations where you deal with dynamic or unpredictable property names. Whether you're using the classic bracket notation or leveraging ES6 computed property names, this technique will greatly enhance your ability to handle dynamic data structures efficiently. By understanding and implementing these methods, you can write more flexible and maintainable JavaScript code.