filmov
tv
LeetCode Daily: Build a Matrix With Conditions Solution in Java | July 21, 2024 #leetcodechallenge
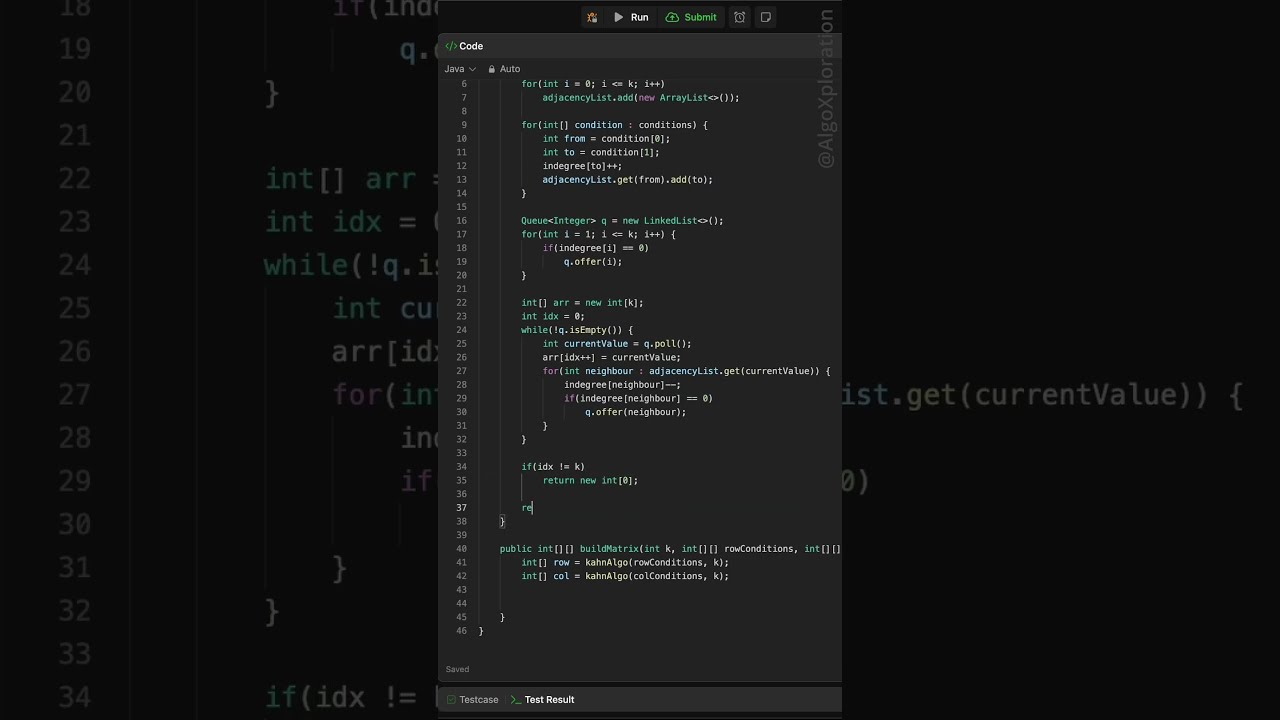
Показать описание
🔍 **LeetCode Problem of the Day**: Build a Matrix With Conditions
Welcome to today's LeetCode challenge! In this video, I will show you how to solve the problem "Build a Matrix With Conditions" in Java. This problem involves constructing a matrix based on given conditions using graph theory and topological sorting.
👉 **Solution**: Pinned on the comments
### 🌟 Problem Description:
The problem "Build a Matrix With Conditions" requires you to construct a matrix that satisfies certain conditions using graph theory principles and topological sorting. This problem is great for practicing advanced algorithm techniques in Java.
### 🔑 Key Points:
1. Understand the problem requirements and constraints.
2. Use graph theory and topological sort to construct the matrix.
3. Implement the solution in Java.
### 📅 **Daily Solutions**:
I will be posting solutions to the LeetCode daily problems every day. Make sure to subscribe and hit the bell icon to stay updated!
### 👥 **Join the Community**:
- Share your solutions in the comments.
- Connect with fellow coders and enhance your problem-solving skills.
If you enjoyed this video, don't forget to like, share, and subscribe for more daily LeetCode solutions!
#LeetCode #Coding #Programming #TechInterview #GraphTheory #TopologicalSort #Algorithm #DailyChallenge #Java #YouTubeShorts
Welcome to today's LeetCode challenge! In this video, I will show you how to solve the problem "Build a Matrix With Conditions" in Java. This problem involves constructing a matrix based on given conditions using graph theory and topological sorting.
👉 **Solution**: Pinned on the comments
### 🌟 Problem Description:
The problem "Build a Matrix With Conditions" requires you to construct a matrix that satisfies certain conditions using graph theory principles and topological sorting. This problem is great for practicing advanced algorithm techniques in Java.
### 🔑 Key Points:
1. Understand the problem requirements and constraints.
2. Use graph theory and topological sort to construct the matrix.
3. Implement the solution in Java.
### 📅 **Daily Solutions**:
I will be posting solutions to the LeetCode daily problems every day. Make sure to subscribe and hit the bell icon to stay updated!
### 👥 **Join the Community**:
- Share your solutions in the comments.
- Connect with fellow coders and enhance your problem-solving skills.
If you enjoyed this video, don't forget to like, share, and subscribe for more daily LeetCode solutions!
#LeetCode #Coding #Programming #TechInterview #GraphTheory #TopologicalSort #Algorithm #DailyChallenge #Java #YouTubeShorts
Комментарии