filmov
tv
Troubleshooting Common TypeError Issues in Python Programming
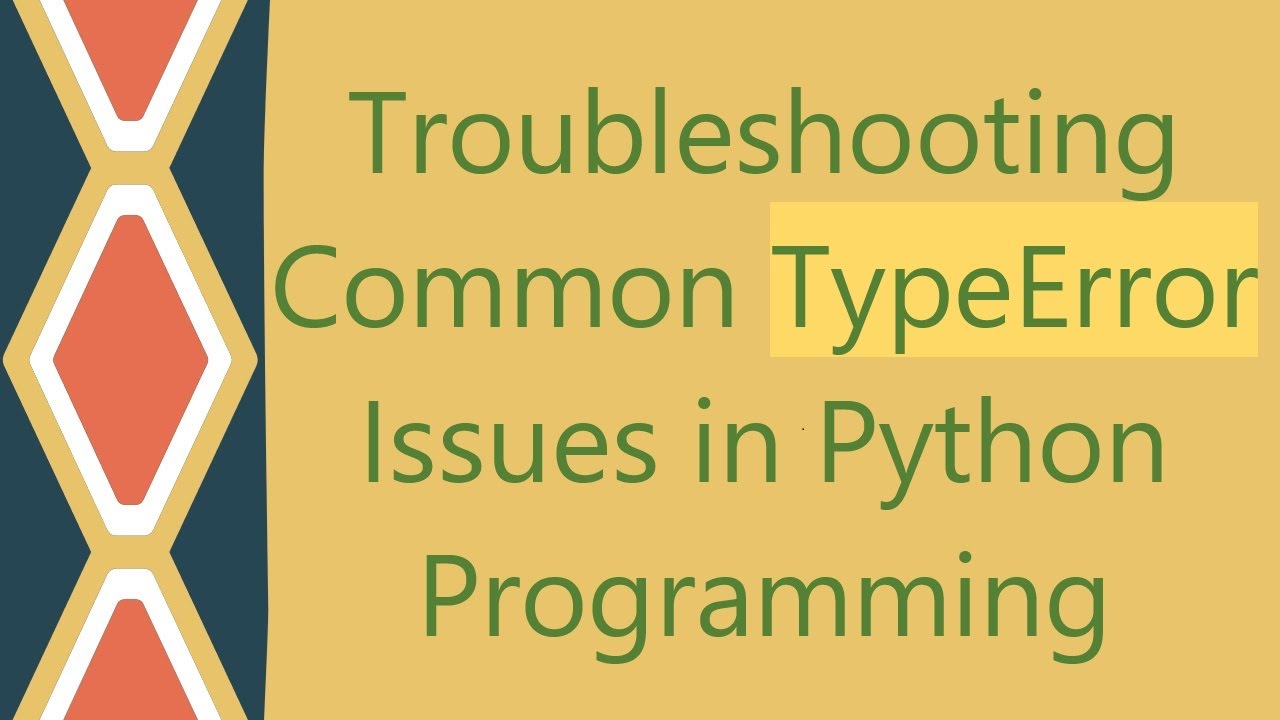
Показать описание
Summary: Discover solutions to common `TypeError` issues in Python, such as "there are no type variables left in list," "`type` object is not subscriptable," and "list object is not callable."
---
Troubleshooting Common TypeError Issues in Python Programming
In Python programming, TypeError is a frequently encountered exception that can often puzzle both novice and experienced programmers. This guide aims to demystify some of the most common TypeError issues and provide practical solutions to tackle them effectively.
TypeError: "There Are No Type Variables Left in List"
One of the less intuitive TypeError messages you might encounter is:
[[See Video to Reveal this Text or Code Snippet]]
This usually occurs when you're dealing with type hints and generics but misuse them. For example:
[[See Video to Reveal this Text or Code Snippet]]
This code throws an error because it lacks a concrete type for the List. Python requires a specific type:
[[See Video to Reveal this Text or Code Snippet]]
Here, we specify that items is a list of integers, resolving the TypeError.
TypeError: "'type' Object Is Not Subscriptable"
Another common error is:
[[See Video to Reveal this Text or Code Snippet]]
This issue surfaces when mistakenly trying to subscript a type object. Consider the code snippet:
[[See Video to Reveal this Text or Code Snippet]]
This throws a TypeError because list as a type object cannot be subscripted. To fix this:
[[See Video to Reveal this Text or Code Snippet]]
This simple modification ensures you're creating an instance of a list, not attempting to subscript a type object.
TypeError: "List Object Is Not Callable"
Ever encountered this error?
[[See Video to Reveal this Text or Code Snippet]]
This often occurs when you mistakenly attempt to call a list as if it were a function. For instance:
[[See Video to Reveal this Text or Code Snippet]]
To fix this, ensure you're using the proper methods intended for list operations:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
TypeError issues can be tricky, but by understanding the context and making specific corrections, you can resolve these common errors in your Python code. Whenever you encounter these errors, revisit the type annotations, ensure proper usage of type objects, and double-check that your lists and other data structures are being used correctly.
Happy coding!
---
Troubleshooting Common TypeError Issues in Python Programming
In Python programming, TypeError is a frequently encountered exception that can often puzzle both novice and experienced programmers. This guide aims to demystify some of the most common TypeError issues and provide practical solutions to tackle them effectively.
TypeError: "There Are No Type Variables Left in List"
One of the less intuitive TypeError messages you might encounter is:
[[See Video to Reveal this Text or Code Snippet]]
This usually occurs when you're dealing with type hints and generics but misuse them. For example:
[[See Video to Reveal this Text or Code Snippet]]
This code throws an error because it lacks a concrete type for the List. Python requires a specific type:
[[See Video to Reveal this Text or Code Snippet]]
Here, we specify that items is a list of integers, resolving the TypeError.
TypeError: "'type' Object Is Not Subscriptable"
Another common error is:
[[See Video to Reveal this Text or Code Snippet]]
This issue surfaces when mistakenly trying to subscript a type object. Consider the code snippet:
[[See Video to Reveal this Text or Code Snippet]]
This throws a TypeError because list as a type object cannot be subscripted. To fix this:
[[See Video to Reveal this Text or Code Snippet]]
This simple modification ensures you're creating an instance of a list, not attempting to subscript a type object.
TypeError: "List Object Is Not Callable"
Ever encountered this error?
[[See Video to Reveal this Text or Code Snippet]]
This often occurs when you mistakenly attempt to call a list as if it were a function. For instance:
[[See Video to Reveal this Text or Code Snippet]]
To fix this, ensure you're using the proper methods intended for list operations:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
TypeError issues can be tricky, but by understanding the context and making specific corrections, you can resolve these common errors in your Python code. Whenever you encounter these errors, revisit the type annotations, ensure proper usage of type objects, and double-check that your lists and other data structures are being used correctly.
Happy coding!