filmov
tv
1 Problem, 4 More Programming Languages (Python vs Kotlin vs F# vs Wolfram)
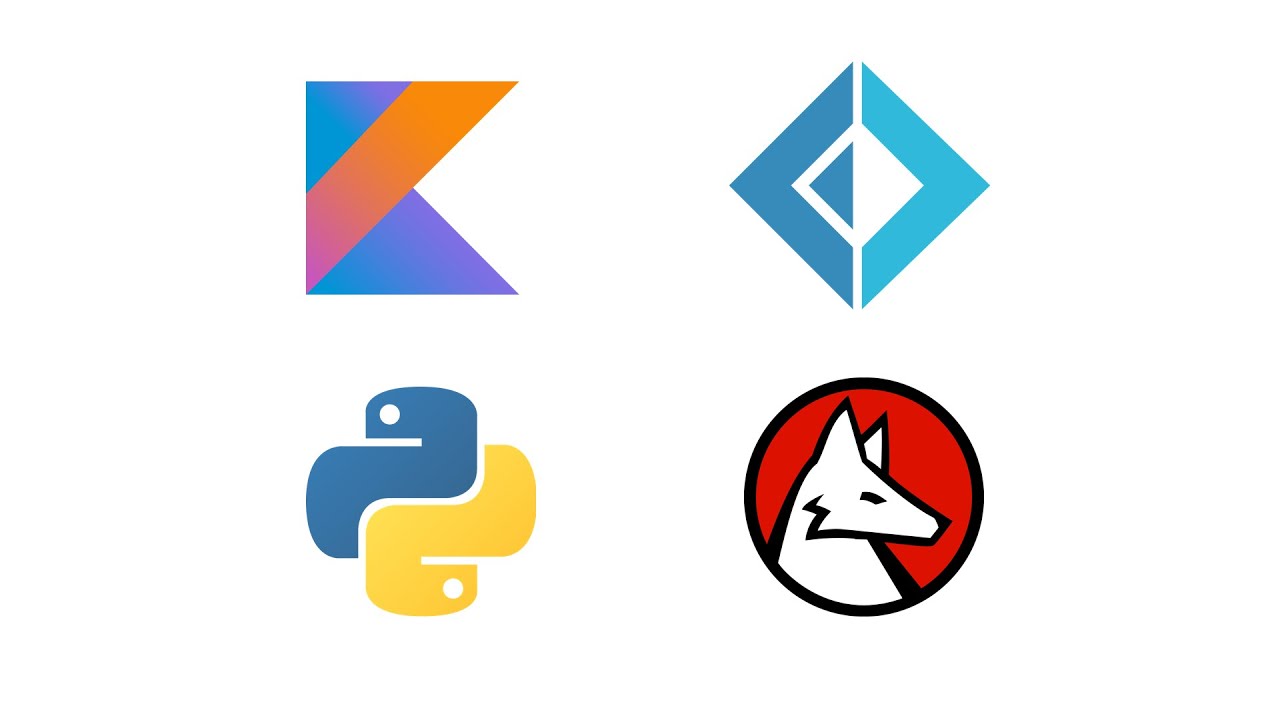
Показать описание
A video taking a look at 4 more programming language solutions (Python, Kotlin, F# & Wolfram Language) to one problem.
Github Links:
Github Links:
1 Problem, 4 More Programming Languages (Python vs Kotlin vs F# vs Wolfram)
1 Problem, 24 Programming Languages
1 Problem, 5 Programming Languages
Maple Programming Test 1: Problem 4
Programming Problem #4 - A Set of Beginner Coding Problems & Solutions
Google Medium Dynamic Programming Problem - Leetcode 64 - Minimum Path Sum
1 Problem, 6 Programming Languages (C++ vs Rust vs Haskell vs APL vs Clojure vs Scala)
How to solve (almost) any binary tree coding problem
PYTHON PROBLEM SOLVING SERIES [PROBLEM-1] |#programming #python #pythonprogramming #pythontutorial
algorithm & flowchart problem #shorts #c programming
1 Problem, 16 Programming Languages (C++ vs Rust vs Haskell vs Python vs APL...)
Problem Solving In Programming | Problem Solving Skills For Programming | Simplilearn
Social Security Fairness Act passes in the Senate
Solving linear programming problems with Excel Solver
Single Push button ON/OFF PLC Program - Example Problems for Practice
Mastering Dynamic Programming - How to solve any interview problem (Part 1)
Do THIS To Become A Better Problem Solver #coding #progamming #computerscience
Python Quiz #59 Fix the Error in Python: A Coding Challenge | Python for Beginners | MCQs
LG TV Not Program Problem solve।TV Main not program solve kaise kare।#tv #youtubeshorts
0/1 Knapsack problem | Dynamic Programming
0/1 Knapsack Problem TABLE FILLING APPROACH with Coding | Easy Intuitive Method | DP Deep Dive
5 Simple Steps for Solving Dynamic Programming Problems
Mastering Dynamic Programming - A Real-Life Problem (Part 2)
Daily Coding Problem - Problem 4 (First missing positive number)
Комментарии