filmov
tv
Understanding and Fixing the list index out of range Error in Python Matrix Manipulation
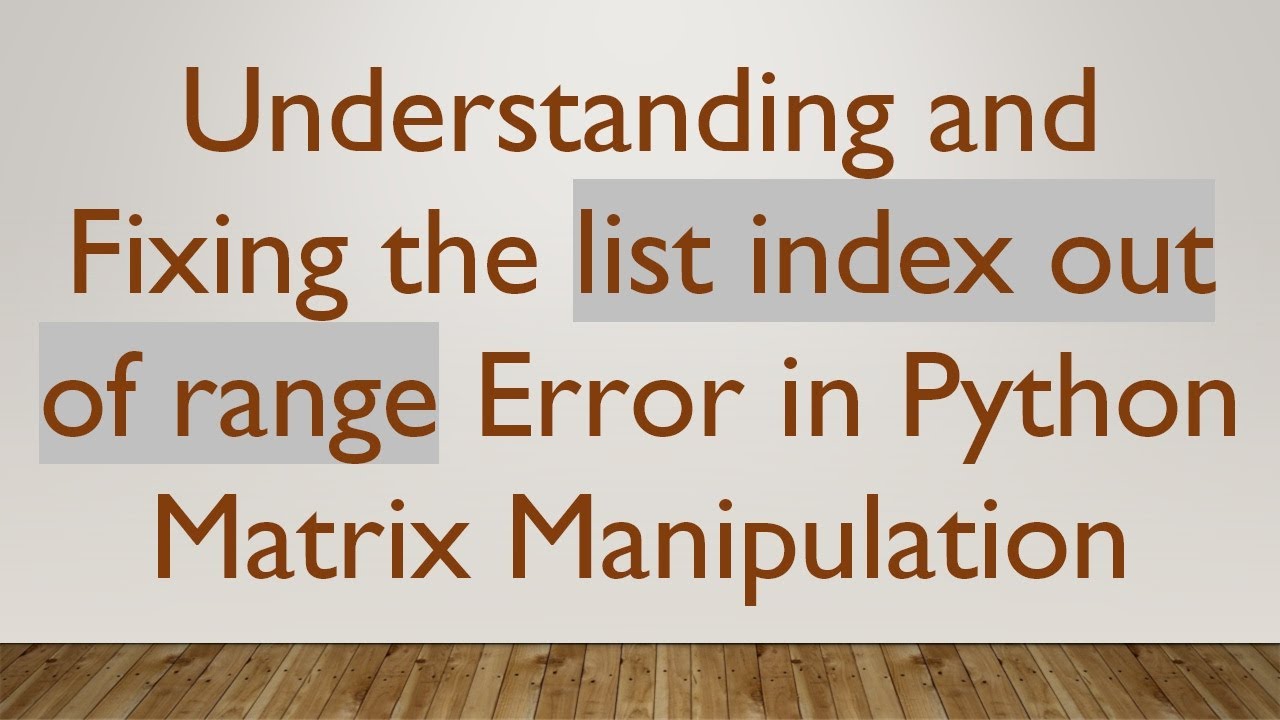
Показать описание
Learn how to resolve the `list index out of range` error in Python while working with a 10x10 matrix to modify cells based on the given condition.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: what is causing the list index out of range error here?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Fixing the list index out of range Error in Python Matrix Manipulation
Working with matrices in Python can be straightforward, but it often presents some challenges. One common issue is encountering the dreaded list index out of range error. If you’re dealing with a matrix where you need to modify entire rows and columns based on cell values, understanding the source of this error is crucial.
The Problem: List Index Out of Range
In our case, the goal is to modify a 10x10 matrix so that if a cell's value is 1, all cells in the same row and column should also be set to 1. Let’s take a look at the original code that’s intended to perform this task:
[[See Video to Reveal this Text or Code Snippet]]
What Went Wrong?
Matrix Initialization: The matrix is correctly initialized with user input, but there's a logical flow issue in navigating through the matrix.
Loops and Indexes: The nested loops go through i and j, representing the row and column indices. However, the inner while loop can inadvertently modify j and i beyond matrix bounds, especially when the conditions of j incrementing or resetting to 0 are not handled cautiously.
The IndexError
The IndexError occurs when the code attempts to access an index that doesn’t exist in the mat. In particular, if j climbs to 10 or if the outer loop increments i without proper safeguarding, trying to access mat[i][j] will result in this error.
Solution: Refactoring the Code
An effective way to avoid such errors and improve readability is by using a more structured approach. We can first identify all the positions that need to be modified and later apply the changes. Here is a rewritten version of the solution:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the New Approach
List Comprehension: We gather the positions of 1s in a clean and concise way using a list comprehension.
Modification Loop: A separate loop then efficiently modifies the targeted rows and columns based on these positions, which is less error-prone.
Readability: This method separates the identification of points from their modification, making debugging and future adjustments easier.
Conclusion
We’ve explored the common pitfalls that lead to the list index out of range error and provided an efficient way to address this issue by restructuring the code for clarity and robustness. By identifying the cells to modify first, we can prevent the pitfalls associated with index manipulations and improve the overall code quality.
Make sure to test your code with different matrix configurations to ensure that it handles all edge cases gracefully!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: what is causing the list index out of range error here?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Fixing the list index out of range Error in Python Matrix Manipulation
Working with matrices in Python can be straightforward, but it often presents some challenges. One common issue is encountering the dreaded list index out of range error. If you’re dealing with a matrix where you need to modify entire rows and columns based on cell values, understanding the source of this error is crucial.
The Problem: List Index Out of Range
In our case, the goal is to modify a 10x10 matrix so that if a cell's value is 1, all cells in the same row and column should also be set to 1. Let’s take a look at the original code that’s intended to perform this task:
[[See Video to Reveal this Text or Code Snippet]]
What Went Wrong?
Matrix Initialization: The matrix is correctly initialized with user input, but there's a logical flow issue in navigating through the matrix.
Loops and Indexes: The nested loops go through i and j, representing the row and column indices. However, the inner while loop can inadvertently modify j and i beyond matrix bounds, especially when the conditions of j incrementing or resetting to 0 are not handled cautiously.
The IndexError
The IndexError occurs when the code attempts to access an index that doesn’t exist in the mat. In particular, if j climbs to 10 or if the outer loop increments i without proper safeguarding, trying to access mat[i][j] will result in this error.
Solution: Refactoring the Code
An effective way to avoid such errors and improve readability is by using a more structured approach. We can first identify all the positions that need to be modified and later apply the changes. Here is a rewritten version of the solution:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the New Approach
List Comprehension: We gather the positions of 1s in a clean and concise way using a list comprehension.
Modification Loop: A separate loop then efficiently modifies the targeted rows and columns based on these positions, which is less error-prone.
Readability: This method separates the identification of points from their modification, making debugging and future adjustments easier.
Conclusion
We’ve explored the common pitfalls that lead to the list index out of range error and provided an efficient way to address this issue by restructuring the code for clarity and robustness. By identifying the cells to modify first, we can prevent the pitfalls associated with index manipulations and improve the overall code quality.
Make sure to test your code with different matrix configurations to ensure that it handles all edge cases gracefully!