filmov
tv
Delete the Middle Node of a Linked List | Leetcode 2095 | Python | Explained | Simple | 2 Solution
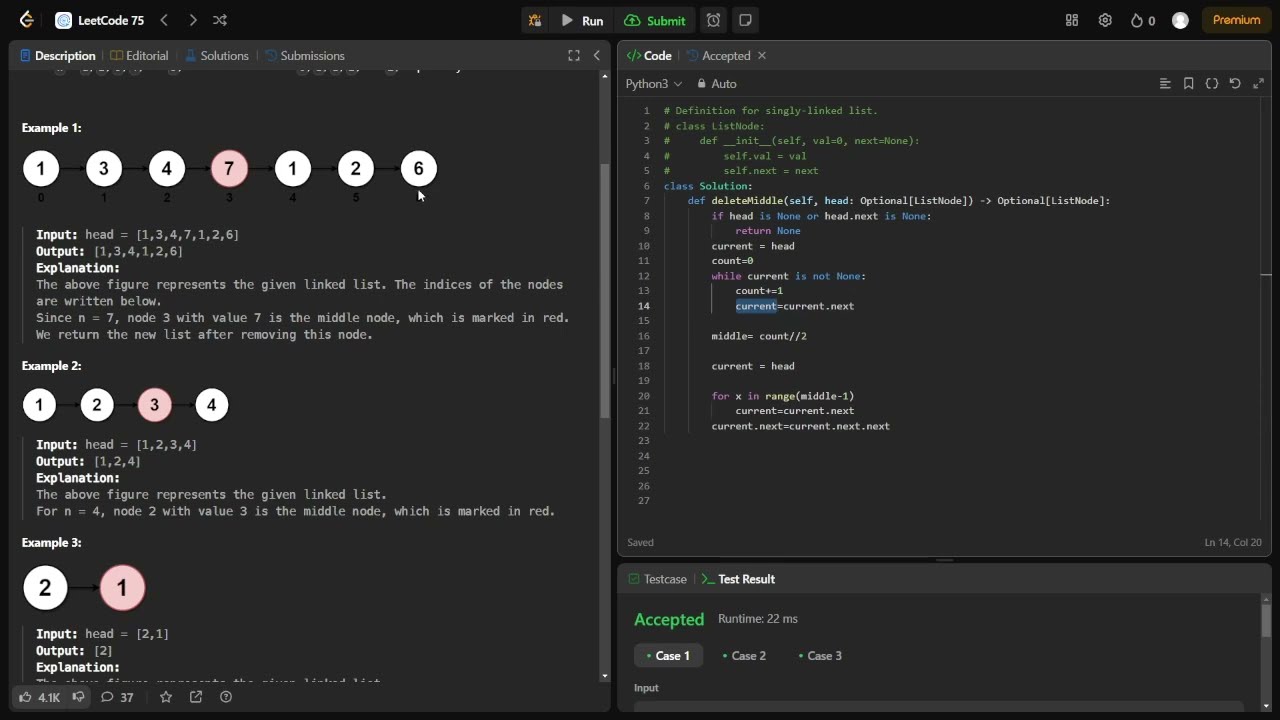
Показать описание
Welcome back, everyone! In today's video, we are going to tackle the "Delete the Middle Node of a Linked List" problem from Leetcode (Problem 2095). This is a classic problem that tests your understanding of linked list operations. We'll walk through the problem step-by-step and explore two efficient solutions to help you master this concept.
📝 Problem Description:
You are given the head of a singly linked list. You need to delete the middle node of the linked list and return the head of the modified linked list. If the linked list has an even number of nodes, delete the second middle node.
🔍 Example:
Input:
head = [1,3,4,7,1,2,6]
Output:
[1,3,4,1,2,6]
🚀 Solution Overview:
We'll cover two approaches to solve this problem:
Two-Pointer Approach: This is an efficient method using slow and fast pointers to find the middle node in one pass.
Counting Nodes Approach: This involves counting the total number of nodes and then deleting the middle node in a second pass.
🧩 Approach 1: Two-Pointer Approach
This is a popular technique for linked list problems. The idea is to use two pointers, slow and fast. The fast pointer moves twice as fast as the slow pointer. By the time the fast pointer reaches the end of the list, the slow pointer will be at the middle node. Here's a step-by-step explanation and implementation in Python.
🧩 Approach 2: Counting Nodes Approach
In this method, we first traverse the linked list to count the total number of nodes. Then, we traverse the list again to find the middle node and delete it. This approach might take a bit longer but is straightforward and easy to understand. We'll also implement this approach in Python.
💡 Key Concepts:
Linked Lists
Two-pointer technique
Node deletion in linked lists
📚 What You'll Learn:
By the end of this video, you'll understand:
How to efficiently find the middle node of a linked list.
How to delete a node in a linked list.
Two different methods to solve the same problem, providing a deeper understanding of linked list operations.
🔧 Code Implementation:
We'll provide the full Python code for both approaches, and I'll walk you through each line to ensure you understand the logic and flow.
💬 Join the Discussion:
If you have any questions or need further clarifications, feel free to leave a comment below. I love interacting with you all and helping out where I can.
👍 Don't Forget to Like, Share, and Subscribe:
If you found this video helpful, please give it a thumbs up and share it with your friends. Also, don't forget to subscribe to my channel and hit the bell icon to get notified whenever I upload new content.
📝 Problem Description:
You are given the head of a singly linked list. You need to delete the middle node of the linked list and return the head of the modified linked list. If the linked list has an even number of nodes, delete the second middle node.
🔍 Example:
Input:
head = [1,3,4,7,1,2,6]
Output:
[1,3,4,1,2,6]
🚀 Solution Overview:
We'll cover two approaches to solve this problem:
Two-Pointer Approach: This is an efficient method using slow and fast pointers to find the middle node in one pass.
Counting Nodes Approach: This involves counting the total number of nodes and then deleting the middle node in a second pass.
🧩 Approach 1: Two-Pointer Approach
This is a popular technique for linked list problems. The idea is to use two pointers, slow and fast. The fast pointer moves twice as fast as the slow pointer. By the time the fast pointer reaches the end of the list, the slow pointer will be at the middle node. Here's a step-by-step explanation and implementation in Python.
🧩 Approach 2: Counting Nodes Approach
In this method, we first traverse the linked list to count the total number of nodes. Then, we traverse the list again to find the middle node and delete it. This approach might take a bit longer but is straightforward and easy to understand. We'll also implement this approach in Python.
💡 Key Concepts:
Linked Lists
Two-pointer technique
Node deletion in linked lists
📚 What You'll Learn:
By the end of this video, you'll understand:
How to efficiently find the middle node of a linked list.
How to delete a node in a linked list.
Two different methods to solve the same problem, providing a deeper understanding of linked list operations.
🔧 Code Implementation:
We'll provide the full Python code for both approaches, and I'll walk you through each line to ensure you understand the logic and flow.
💬 Join the Discussion:
If you have any questions or need further clarifications, feel free to leave a comment below. I love interacting with you all and helping out where I can.
👍 Don't Forget to Like, Share, and Subscribe:
If you found this video helpful, please give it a thumbs up and share it with your friends. Also, don't forget to subscribe to my channel and hit the bell icon to get notified whenever I upload new content.