filmov
tv
Single Linked List (Deleting the Node at a Particular Position)
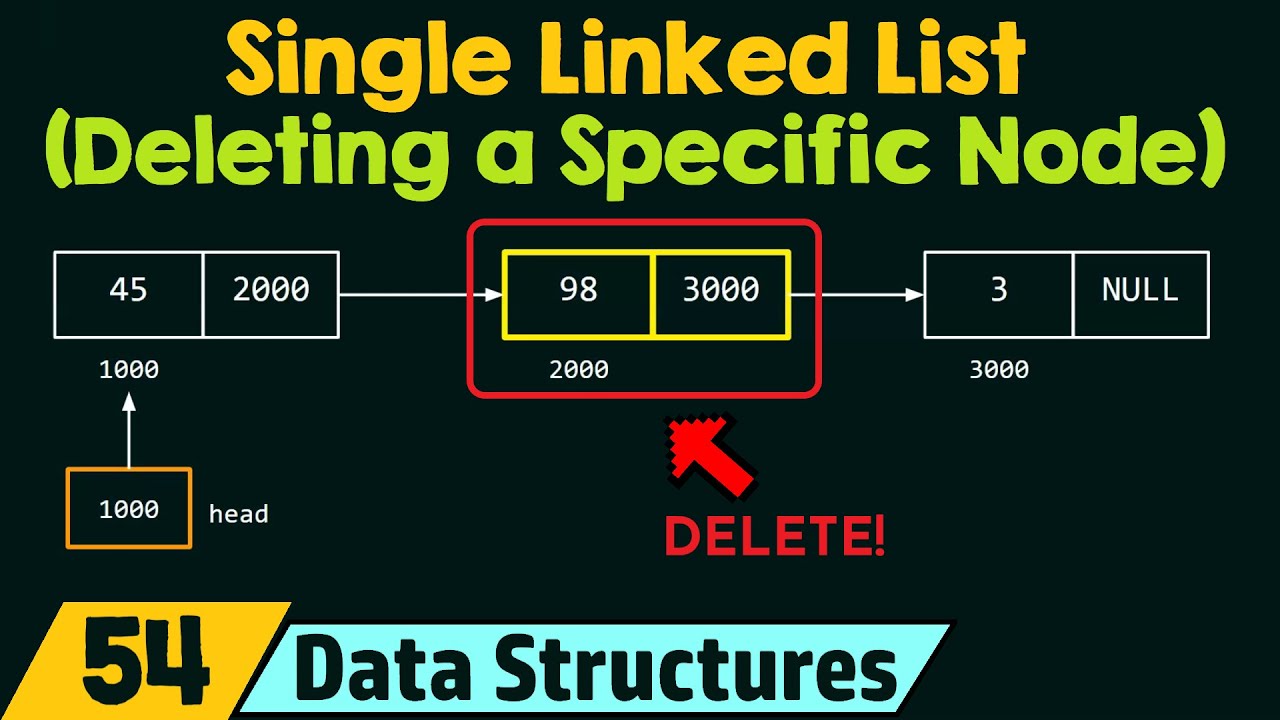
Показать описание
Data Structures: Deleting the Node of a Singly Linked List at a Specified Position.
Topics discussed:
1) C program to delete the node of a singly linked list present at a particular position.
Music:
Axol x Alex Skrindo - You [NCS Release]
#DataStructuresByNeso #DataStructures #LinkedList #SingleLinkedList
Topics discussed:
1) C program to delete the node of a singly linked list present at a particular position.
Music:
Axol x Alex Skrindo - You [NCS Release]
#DataStructuresByNeso #DataStructures #LinkedList #SingleLinkedList
Single Linked List (Deleting the First Node)
Single Linked List (Deleting the Node at a Particular Position)
Single Linked List (Deleting the Last Node)
Deleting the Entire Single Linked List
2.6 Deletion of a node from Linked List (from beginning, end, specified position) | DSA Tutorials
Single Linked List (Deleting the Last Node using Single Pointer)
Circular Singly Linked List (Deleting the First Node)
Circular Singly Linked List (Deleting the Last Node)
Queue using Linked List in C | Enqueue Operation of Queue | Implement Queue in C
Circular Singly Linked List (Deleting the Intermediate Node)
Delete first node from Singly Linked List | Algorithm | Data Structure
Doubly Linked List (Deleting the First Node)
Delete a linked list (all nodes)
Singly Linked List | Insert, Delete, Complexity Analysis
LINKED LIST (DELETION FROM BEGINNING,ENDING AND SPECIFIED POSITION) - DATA STRUCTURES
Singly-Linked Lists - CS50 Shorts
Doubly Linked List (Deleting the Last Node)
Delete Node in a Linked List | Can you solve it ?
Delete Any Node By Value in Linked List | Delete At the End | Python Program
Deleting a node in Linked list | Data Structures Tutorial
Delete a node from single linked list( head node/ middle / end node)
Delete The First Node in Linked List | Delete At the Beginning | Python Program
Deletion in a Linked List | Deleting a node from Linked List Data Structure
Doubly Linked List (Deleting the Intermediate Node)
Комментарии