filmov
tv
Find the Missing and Repeating Number | 4 Approaches 🔥
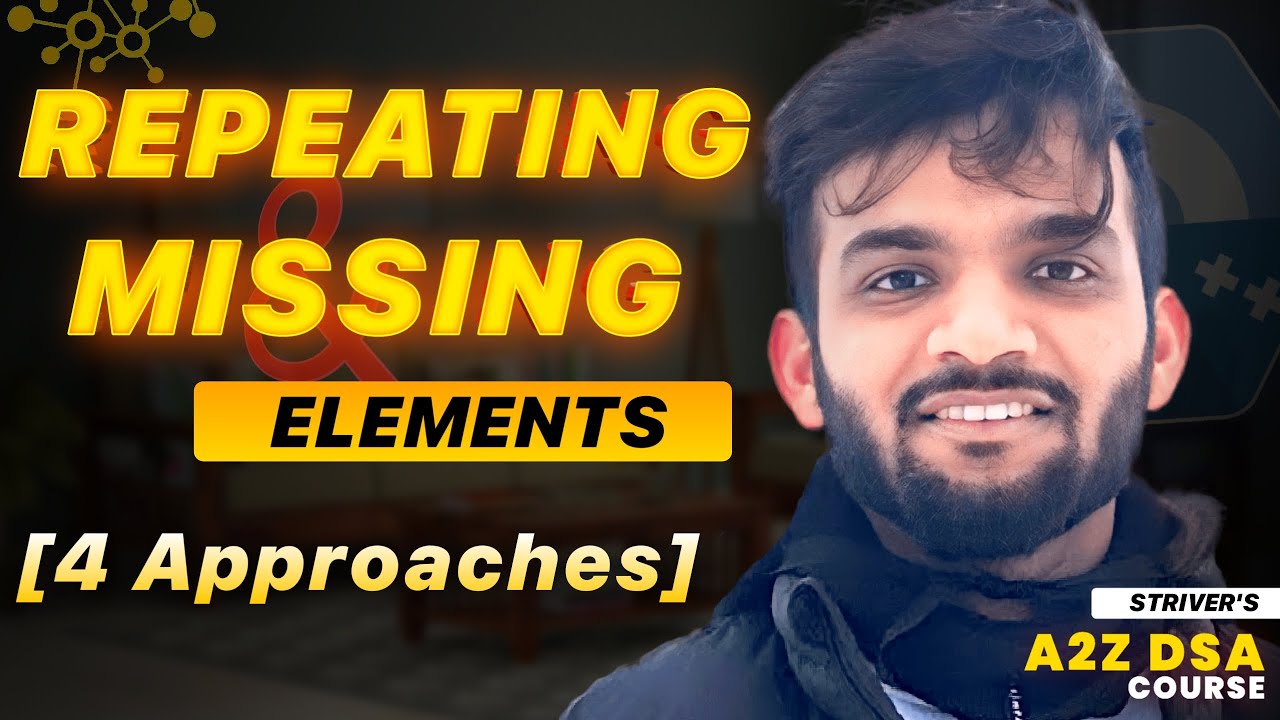
Показать описание
We have solved the problem, and we have gone from brute force and ended with the most optimal solution. Every approach's code has been written in the video itself. Also, we have covered the algorithm with intuition.
You can follow me across social media, all my handles are below:
0:00 Introduction of Course
00:20 Problem Statement
01:14 Brute Force approach
02:08 Pseudocode
03:18 Complexity
03:47 Better approach (Hashing)
05:41 Code
06:58 Complexity
07:28 Optimal approach (Maths + XOR)
08:01 Solution-01 (Maths)
08:08 Intuition + Approach
14:54 Code
19:17 Complexity
19:55 Solution-02 (XOR)
20:15 Intuition + Approach
31:11 Code
41:24 Complexity
Find missing and repeating number
Find the Missing and Repeating Number | 4 Approaches 🔥
Find Missing and Repeating Number | 5 Approaches with Full Code in Comments | Bit Manipulation Array
Find the Missing and Repeating Number | GFG | Python | Optimal
Find the Repeating and the Missing Element | Love Babbar DSA Sheet | Leetcode | Amazon 🔥| Placement...
Find repeating and missing number
Find Duplicate Number and Missing Number from 1 to N | One Duplicate One Missing | Bit Manipulation
Find Missing And Repeating (GeeksforGeeks): Problem of the Day 14/20/2022 | Time: O(N) | Space: O(1)
Find Missing And Repeating | Problem of the Day-14/02/2022 | Yash Dwivedi
Find Missing and Duplicate Number in an Array | Swap Sort Need
Find Missing and Repeating Element in Unsorted Array | GFG | Problem Solving | Hindi | Shashwat
Find the Missing and Repeating Number | GFG | C++ and Java | Brute-Better-Optimal-Optimal
Find Missing and Repeating | geeks for geeks Array problem And it's solution | Difficulty- Med...
Lecture 35: Majority Elements || Count Frequency of element | Find Missing and Repeating elements
Find missing and repeating number
Find Missing and Repeating number in an array - Amz, Samsung, DE-Shaw, Goldman Sachs
Find Missing And Repeating
#9 Find Missing and Repeating Number in an Array | Bit Manipulation | GeeksforGeeks | V. Imp 🔥
Find the Missing and Repeating Number | GFG✅ | DSA
Find a missing and repeating element in an unsorted array
Find Missing And Repeating in array | Searching and Sorting | Love Babbar DSA Sheet | Amazon🔥
L07 : Missing & Repeating Number | Bit Manipulation | CodeNCode
Find the missing and repeating number from 1 to N | GFG | DSA | Placement course
20. Find the missing and repeating number | Array | Problem Solving
Комментарии