filmov
tv
8.6 Subarrays - Challenges | Questions asked by Top MNC's | C++ Placement Course
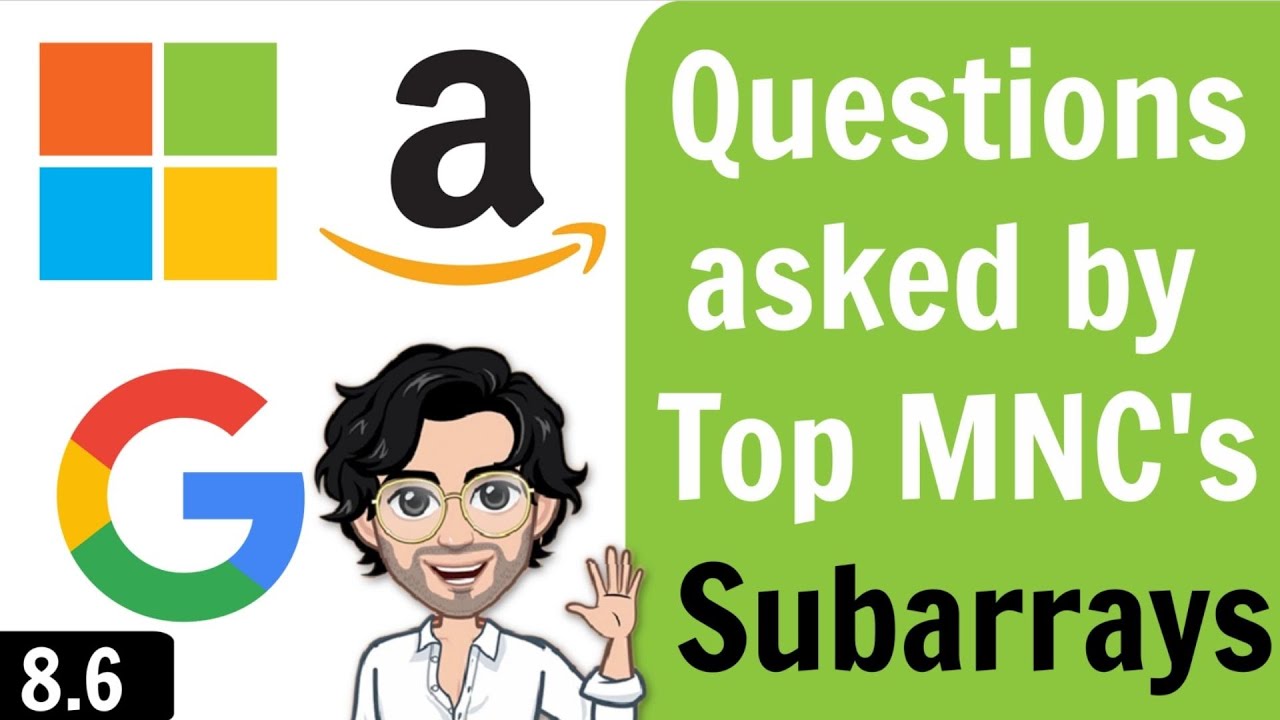
Показать описание
8.6 Subarrays - Challenges | Questions asked by Top MNC's | C++ Placement Course
Subarray with given sum
Maximum Subarray - Amazon Coding Interview Question - Leetcode 53 - Python
8 patterns to solve 80% Leetcode problems
Find Subarray with the given sum k 🔥| Hashmap in Java | DSA-One Course #28
Finding Maximum Sum SubArray using Divide and Conquer Approach.
Kadane's Algorithm | Largest Sum Contiguous Subarray | Java and C++ | Anuj Bhaiya ✅DSAOne Cours...
Kadanes algorithm | Longest sum contiguous subarray
Prefix Sum Array Explained
Maximum of all subarrays of size K | Leetcode #239
Kadane's Algo in 16 minutes || Algorithms for Placements
LeetCode Minimum Size Subarray Sum Solution Explained - Java
Subarray Divisibility (AtCoder)
Minimum Size Subarray Sum - Leetcode 209 - Python
I gave 127 interviews. Top 5 Algorithms they asked me.
6.2 Sum Of Subsets Problem - Backtracking
Sliding Window Maximum - Monotonic Queue - Leetcode 239
Maximum Subarray Sum | Leetcode | Kadane's Algorithm | Brute-Better-Optimal | CPP/Java
Maximum Product Subarray - Dynamic Programming - Leetcode 152
Sliding Window Technique - Algorithmic Mental Models
25. Subarray Sum Equals K | Leetcode 560 | Array | Prefix Sum
2 Sum Problem | 2 types of the same problem for Interviews | Brute-Better-Optimal
Number of Subarrays with xor K | Brute - Better - Optimal
Subarray with Given Sum | Non Negative Numbers | Programming Tutorials
Комментарии