filmov
tv
Optimizing Arduino Code: no setup(), no loop() ⛔
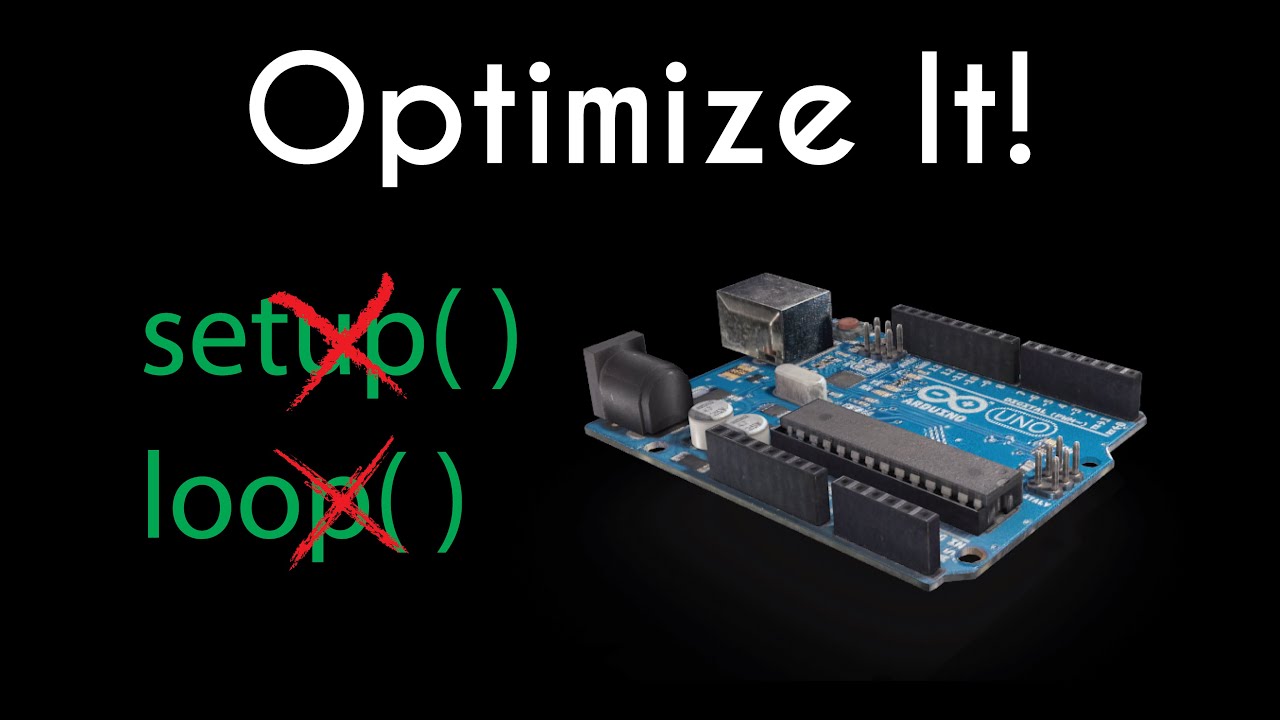
Показать описание
When you write Arduino code, you usually split your code into two parts: the setup() function that runs once, at the beginning of the program, and the loop() function that runs repeatedly. But did you know that it doesn't always have to be this way?
In this video you will learn how to write Arduino code without setup() and loop(), and see some of the benefits that come with it (such as reduced code size), as well as some solutions to common challenges with this method.
Arduino Port Registers Reference:
Arduino main() function source code:
The code from the video:
The Arduino Blink Playground:
In this video you will learn how to write Arduino code without setup() and loop(), and see some of the benefits that come with it (such as reduced code size), as well as some solutions to common challenges with this method.
Arduino Port Registers Reference:
Arduino main() function source code:
The code from the video:
The Arduino Blink Playground:
Optimizing Arduino Code: no setup(), no loop() ⛔
Optimize Your Arduino Code with Registers
Advanced Arduino Code Optimisation with Pin Registers
How to Use Arduino Compiler Optimizations to Produce Faster and Smaller Code
MEMORY OPTIMIZATION | ARDUINO | ERROR: Low memory available stability problems may occur
How to Organize Code
How Fast Does Your Arduino Code Run? ⏱
manually writing data to a HDD...kinda #shorts
How to do multiple tasks in Arduino | Beginners | millis() function
Senior Programmers vs Junior Developers #shorts
Getting Started with Baremetal Arduino C Programming | No IDE Required [Linux SDK]
Arduino in 100 Seconds
Debugging the Arduino Uno or Nano! (No extra hardware needed!)
The HARDEST part about programming 🤦♂️ #code #programming #technology #tech #software #developer...
Double your Arduino IDE Compiling Speed - ESP32, Arduino
#200 Feel the Need for Speed? GCC Compiler Optimisation for the Arduino
IMPROVE YOUR ARDUINO CODE WITH THESE TWO BASIC THINGS - Indentation and Comments
3D Printed Pin Support Challenge 😱
7 Arduino Tips for New Programmers
Arduino: Optimizing an Arduino code (2 Solutions!!)
Port Register Control | Increase speed of Read/Write - Arduino101
Nesting 'If Statements' Is Bad. Do This Instead.
AMD Sempron Cpu processor .Removing pins For Gold Recovery
Testing Stable Diffusion inpainting on video footage #shorts
Комментарии