filmov
tv
Very Common FAANG Interview Question! | Index of the First Occurrence in a String - Leetcode 28
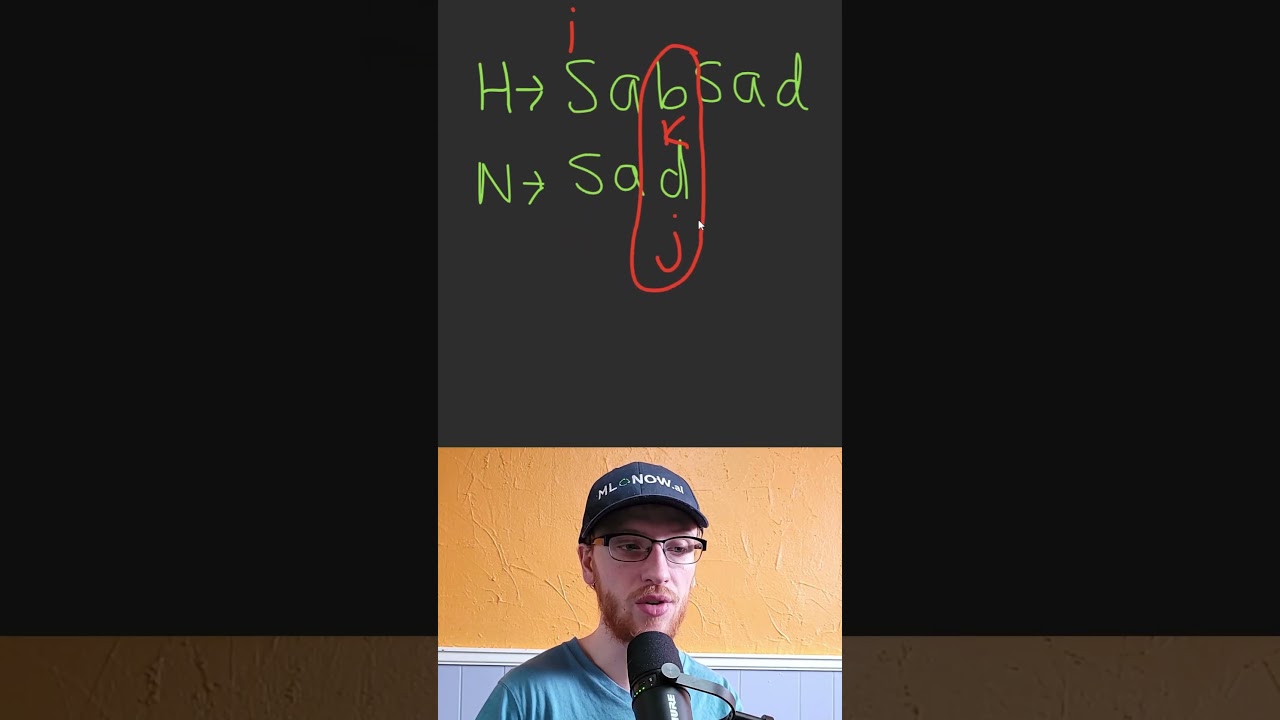
Показать описание
dynamic programming, leetcode, coding interview question, data structures, data structures and algorithms, faang
This is the Most Asked FAANG Interview Question! - Two Sum - Leetcode 1
Very Common FAANG Interview Question! | Index of the First Occurrence in a String - Leetcode 28
VERY COMMON FAANG INTERVIEW QUESTION | Leetcode 27 - Remove Element
I HATE This Coding Question, but FAANG Loves it! | Majority Element - Leetcode 169
How To Pass Coding Interviews Like the Top 1%
FAANG Behavioral Interview Guide | Most Common Question Types | Right Way & Wrong Way to Answer!
How To Pass Technical Interviews When You Suck At LeetCode
How to NOT Fail a Technical Interview
Google LOVES This Coding Interview Question! | Happy Number - Leetcode 202
Top 6 Coding Interview Concepts (Data Structures & Algorithms)
BEST Way To Approach Technical Interviews
Dissecting FAANG Interview Questions
Meta Coding Interview Question - Top K Frequent Elements - Leetcode 347
Most Asked Coding Interview Question (Don't Skip !!😮) #shorts
Cracking the Behavioral Interview for Software Developers
Solving JavaScript's Most NOTORIOUS Interview Question
Most Common Concepts for Coding Interviews
I gave 127 interviews. Top 5 Algorithms they asked me.
Code With Me: 24 FAANG Interview Questions
Top 5 Data Structures they asked me in 127 interviews
Can You Solve This Google Interview Question? | Puzzles for Software Engineers Part-6🔍
Top 10 Behavioral Software Engineering Interview Questions
How I Interviewed Candidates at Google
Can you Solve this Google Interview Question? | Puzzle for Software Developers
Комментарии