filmov
tv
Why JavaScript sort() Method Isn't Sorting Numbers as Expected
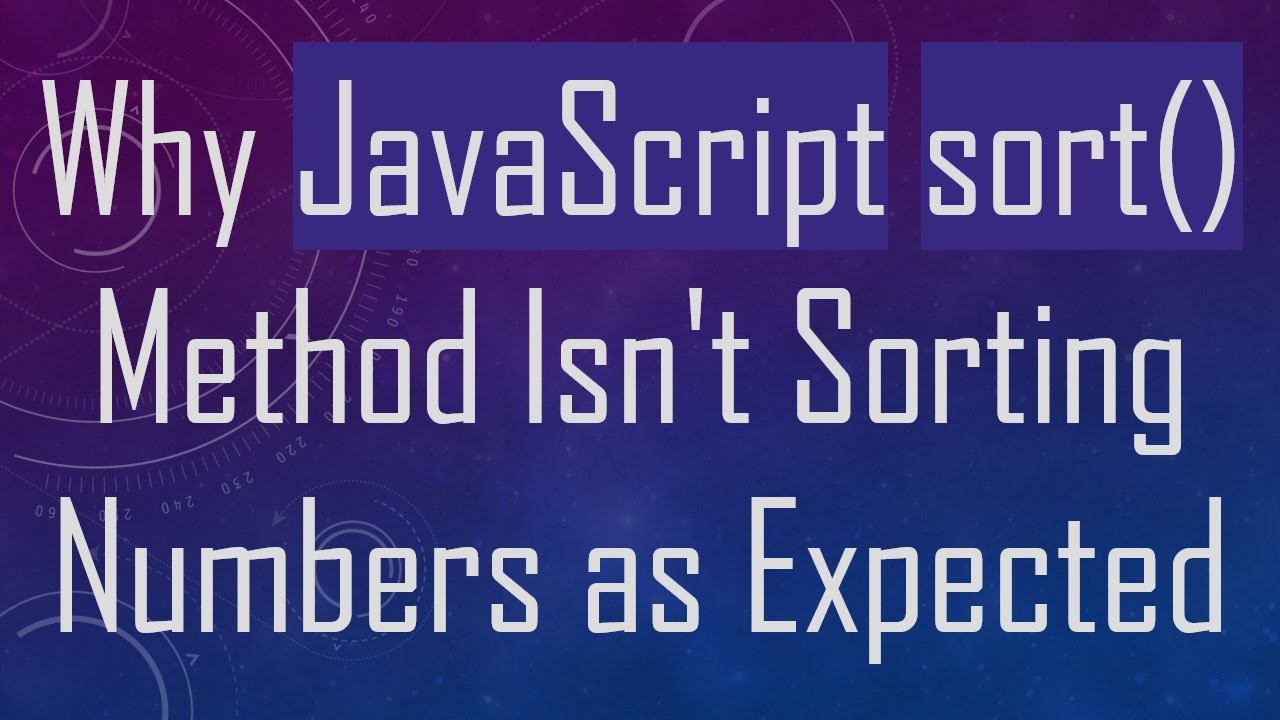
Показать описание
Discover why the JavaScript `sort()` method may not sort numbers as expected and learn how to properly sort numeric arrays.
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
When developing in JavaScript, a common issue developers face is the unexpected behavior of the array sort() method when dealing with numbers. This is a common obstacle for both new and experienced JavaScript enthusiasts. Let's delve into why this happens and how to handle it correctly.
Understanding the Default Behavior
The sort() method in JavaScript, by default, sorts the elements of an array as strings. This can pose a problem when you're trying to sort numbers. For example, if you run the sort method on an array of numbers without any additional arguments, you may notice the order is not what you anticipated. The array [10, 1, 30, 3, 2] if sorted using the default sort() method would return [1, 10, 2, 3, 30].
Why Does This Happen?
The root of this behavior lies in how JavaScript's default sort() method treats the elements as strings for comparison purposes. When numbers are converted to strings, lexicographical ordering takes precedence, leading to results that may seem incorrect if you're expecting numerical order. In our example, JavaScript treats "10" as being less than "2" because "1" is the leading character in "10," which comes lexicographically before "2."
How to Sort Numbers Correctly
To sort numbers correctly, you need to provide a comparison function to the sort() method. This function determines the order of the elements based on numerical comparison rather than lexicographical string order. Here's how you can do it:
[[See Video to Reveal this Text or Code Snippet]]
The comparison function (a, b) => a - b ensures that the numbers are compared numerically. If a is less than b, it returns a negative value, which means a should appear before b. Conversely, if a is greater than b, a positive value is returned, ensuring b comes first. If they are equal, zero is returned, indicating their positions remain unchanged.
Conclusion
Understanding the default sorting behavior of the sort() method is crucial. While it sorts array elements as strings by default, providing a comparison function allows for sorting numbers numerically. Whether you are sorting simple arrays or dealing with more complex data structures, keeping this in mind can save you from unexpected sorting results and make your code more reliable.
Remember, when confronted with unexpected behavior in your code, it's always helpful to refer back to the underlying documentation or seek clarification on the specifics of the language feature you are utilizing.
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
When developing in JavaScript, a common issue developers face is the unexpected behavior of the array sort() method when dealing with numbers. This is a common obstacle for both new and experienced JavaScript enthusiasts. Let's delve into why this happens and how to handle it correctly.
Understanding the Default Behavior
The sort() method in JavaScript, by default, sorts the elements of an array as strings. This can pose a problem when you're trying to sort numbers. For example, if you run the sort method on an array of numbers without any additional arguments, you may notice the order is not what you anticipated. The array [10, 1, 30, 3, 2] if sorted using the default sort() method would return [1, 10, 2, 3, 30].
Why Does This Happen?
The root of this behavior lies in how JavaScript's default sort() method treats the elements as strings for comparison purposes. When numbers are converted to strings, lexicographical ordering takes precedence, leading to results that may seem incorrect if you're expecting numerical order. In our example, JavaScript treats "10" as being less than "2" because "1" is the leading character in "10," which comes lexicographically before "2."
How to Sort Numbers Correctly
To sort numbers correctly, you need to provide a comparison function to the sort() method. This function determines the order of the elements based on numerical comparison rather than lexicographical string order. Here's how you can do it:
[[See Video to Reveal this Text or Code Snippet]]
The comparison function (a, b) => a - b ensures that the numbers are compared numerically. If a is less than b, it returns a negative value, which means a should appear before b. Conversely, if a is greater than b, a positive value is returned, ensuring b comes first. If they are equal, zero is returned, indicating their positions remain unchanged.
Conclusion
Understanding the default sorting behavior of the sort() method is crucial. While it sorts array elements as strings by default, providing a comparison function allows for sorting numbers numerically. Whether you are sorting simple arrays or dealing with more complex data structures, keeping this in mind can save you from unexpected sorting results and make your code more reliable.
Remember, when confronted with unexpected behavior in your code, it's always helpful to refer back to the underlying documentation or seek clarification on the specifics of the language feature you are utilizing.