filmov
tv
Crust of Rust: Send, Sync, and their implementors
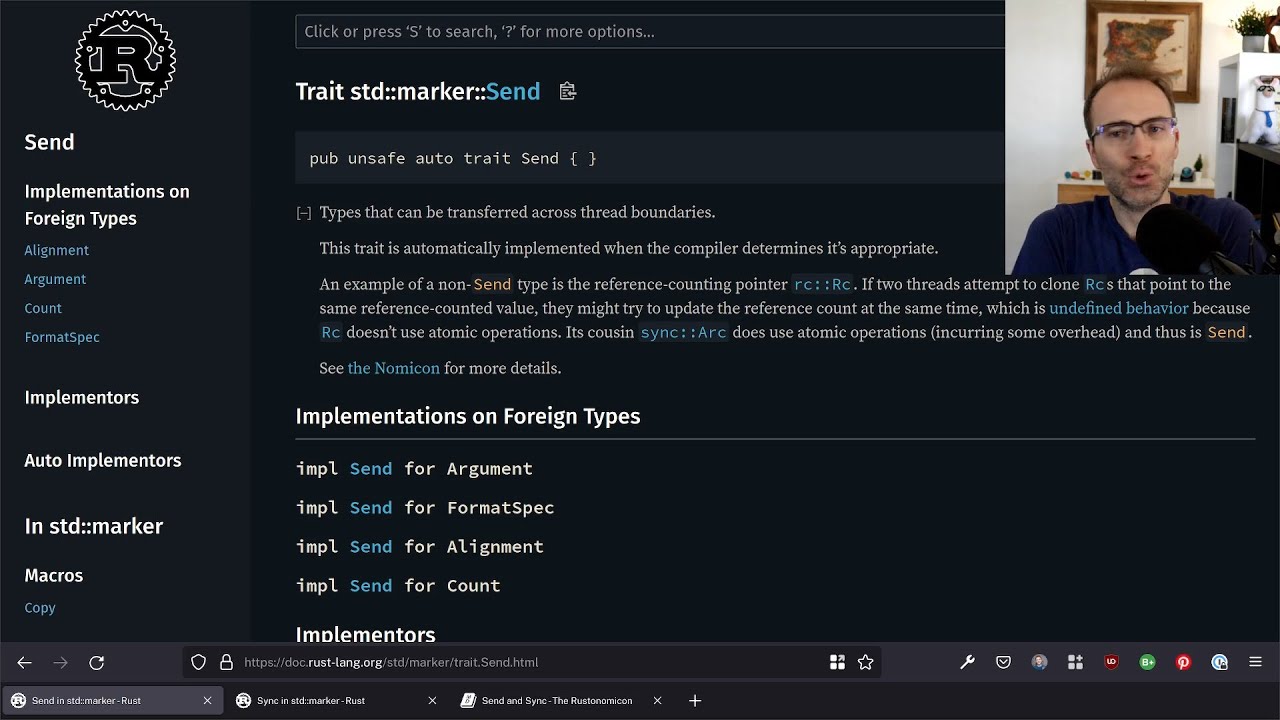
Показать описание
0:00:00 Why Send and Sync?
0:01:31 What are Send and Sync?
0:02:51 Marker traits
0:03:50 Auto traits
0:05:45 The Send trait
0:06:49 Types that aren't Send
0:09:40 Digging into Rc
0:23:52 The Sync trait
0:26:21 Send + !Sync
0:32:41 Negative implementations
0:35:17 Sending mutable references
0:37:00 Raw pointers
0:41:54 std::sync::mpsc and !Sync
0:42:30 Placement of T: Send/Sync bounds
0:46:04 Per-OS impl Send for guards
0:47:06 more std::sync::mpsc and !Sync
0:48:40 Is Send/Sync auto-implemented?
0:50:40 The nomicon Send/Sync entry
0:52:57 There's no magic!
1:02:46 Negative impls on stable
Crust of Rust: Send, Sync, and their implementors
Crust of Rust: async/await
Crust of Rust: Functions, Closures, and Their Traits
Rust: Send and Sync Traits
Crust of Rust: Channels
Crust of Rust: Subtyping and Variance
Crust of Rust: Atomics and Memory Ordering
Rust: Send Data between Threads
Crust of Rust: The Drop Check
Send is not about ownership by Alice Ryhl @ Copenhagen Rust Community
Concurrency in Rust - Sharing State
Easy Rust 122: Introduction to Arc, Send and Sync
Crust of Rust: Smart Pointers and Interior Mutability
Crust of Rust: Dispatch and Fat Pointers
Decrusting the tokio crate
Post-Crust of Rust Q&A
Rust 'did you know': derive isn't perfect
Think Twice Before Using Async Rust | Prime Reacts
Crust of Rust: Lifetime Annotations
[ITA] Rust: Send, Sync and Arc
Rust. Multithreading. Send & Sync
Crust of Rust: Build Scripts and Foreign-Function Interfaces (FFI)
Crust of Rust: Sorting Algorithms
Decrusting the axum crate
Комментарии