filmov
tv
Creating a Generic Function with Textfield Inputs in Swift
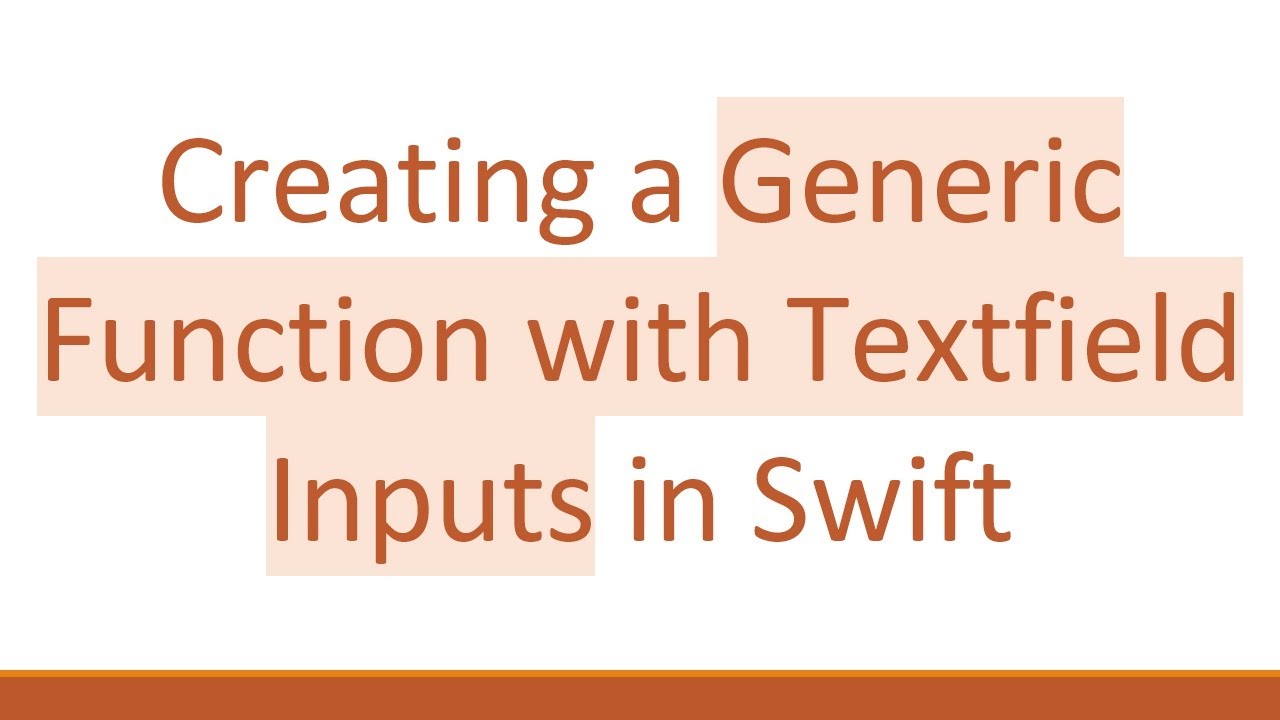
Показать описание
Learn how to build a reusable generic function using textfield inputs in Swift that allows you to compare values against multiple types of data structures.
---
Visit these links for original content and any more details, such as alternate solutions, comments, revision history etc. For example, the original title of the Question was: How to create a generic function with textfield inputs in Swift
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Create a Generic Function with Textfield Inputs in Swift
In the world of Swift programming, developers often come across challenges that require efficient solutions. One such challenge is creating a button linked to a generic function that can work with diverse data types and inputs. In this guide, we will explore how to create a button that generates random elements from an array and verifies if the textfield input matches the correct values linked to those elements.
Understanding the Problem
Imagine you want to have an interactive SwiftUI interface that takes user input and checks it against a pre-defined array of strings or even integers. This may be straightforward if you're only using one data type, but it becomes complicated when you want a generic solution that can handle various data types like strings and integers.
A user came forward with a specific requirement: they need a button that will allow them to create additional "cards" in the view and check if the input matches certain values defined in different arrays.
Example Scenario
Let's say we have the following requirements:
The app should have a user interface with a textfield and button.
The button should compare the text entered in the textfield against a list of correct answers.
The functionality should be generic enough to allow adding different sets of instructions or values beyond strings.
Offering a Solution
Here, we’ll break down how to implement this using Swift and SwiftUI.
Step 1: Create a ViewModel
We will start by creating a ViewModel that is generic. This allows you to pass in different types of values and a logic closure that defines how to check for matches.
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Implement the Matching Logic
You can easily create instances of this ViewModel for different types of data as shown below:
String Example
[[See Video to Reveal this Text or Code Snippet]]
Integer Example
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Utilizing State in SwiftUI
In your SwiftUI view, it's beneficial to use @State properties which can be dynamically updated from user inputs rather than relying solely on the ViewModel. Here’s how you can set it up:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of Code
Generic ViewModel: The VariablesViewModel class allows you to create a generic function that can handle any data type.
Matcher Closure: The closure passed during initialization defines how a match is determined.
State Properties: The @State properties hold the input from the textfield which can be easily accessed for comparison.
Conclusion
Building a generic function with textfield inputs in Swift offers great flexibility for handling multiple data types. Whether you're working with strings or integers, the approach highlighted above provides a structured solution.
Implementing these ideas will enhance your SwiftUIP development skills and allow you to create versatile user interfaces easily. Keep experimenting with different types, and your applications will become even more dynamic and user-friendly!
---
Visit these links for original content and any more details, such as alternate solutions, comments, revision history etc. For example, the original title of the Question was: How to create a generic function with textfield inputs in Swift
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Create a Generic Function with Textfield Inputs in Swift
In the world of Swift programming, developers often come across challenges that require efficient solutions. One such challenge is creating a button linked to a generic function that can work with diverse data types and inputs. In this guide, we will explore how to create a button that generates random elements from an array and verifies if the textfield input matches the correct values linked to those elements.
Understanding the Problem
Imagine you want to have an interactive SwiftUI interface that takes user input and checks it against a pre-defined array of strings or even integers. This may be straightforward if you're only using one data type, but it becomes complicated when you want a generic solution that can handle various data types like strings and integers.
A user came forward with a specific requirement: they need a button that will allow them to create additional "cards" in the view and check if the input matches certain values defined in different arrays.
Example Scenario
Let's say we have the following requirements:
The app should have a user interface with a textfield and button.
The button should compare the text entered in the textfield against a list of correct answers.
The functionality should be generic enough to allow adding different sets of instructions or values beyond strings.
Offering a Solution
Here, we’ll break down how to implement this using Swift and SwiftUI.
Step 1: Create a ViewModel
We will start by creating a ViewModel that is generic. This allows you to pass in different types of values and a logic closure that defines how to check for matches.
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Implement the Matching Logic
You can easily create instances of this ViewModel for different types of data as shown below:
String Example
[[See Video to Reveal this Text or Code Snippet]]
Integer Example
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Utilizing State in SwiftUI
In your SwiftUI view, it's beneficial to use @State properties which can be dynamically updated from user inputs rather than relying solely on the ViewModel. Here’s how you can set it up:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of Code
Generic ViewModel: The VariablesViewModel class allows you to create a generic function that can handle any data type.
Matcher Closure: The closure passed during initialization defines how a match is determined.
State Properties: The @State properties hold the input from the textfield which can be easily accessed for comparison.
Conclusion
Building a generic function with textfield inputs in Swift offers great flexibility for handling multiple data types. Whether you're working with strings or integers, the approach highlighted above provides a structured solution.
Implementing these ideas will enhance your SwiftUIP development skills and allow you to create versatile user interfaces easily. Keep experimenting with different types, and your applications will become even more dynamic and user-friendly!