filmov
tv
java program 22 print diamond shape star pattern in java
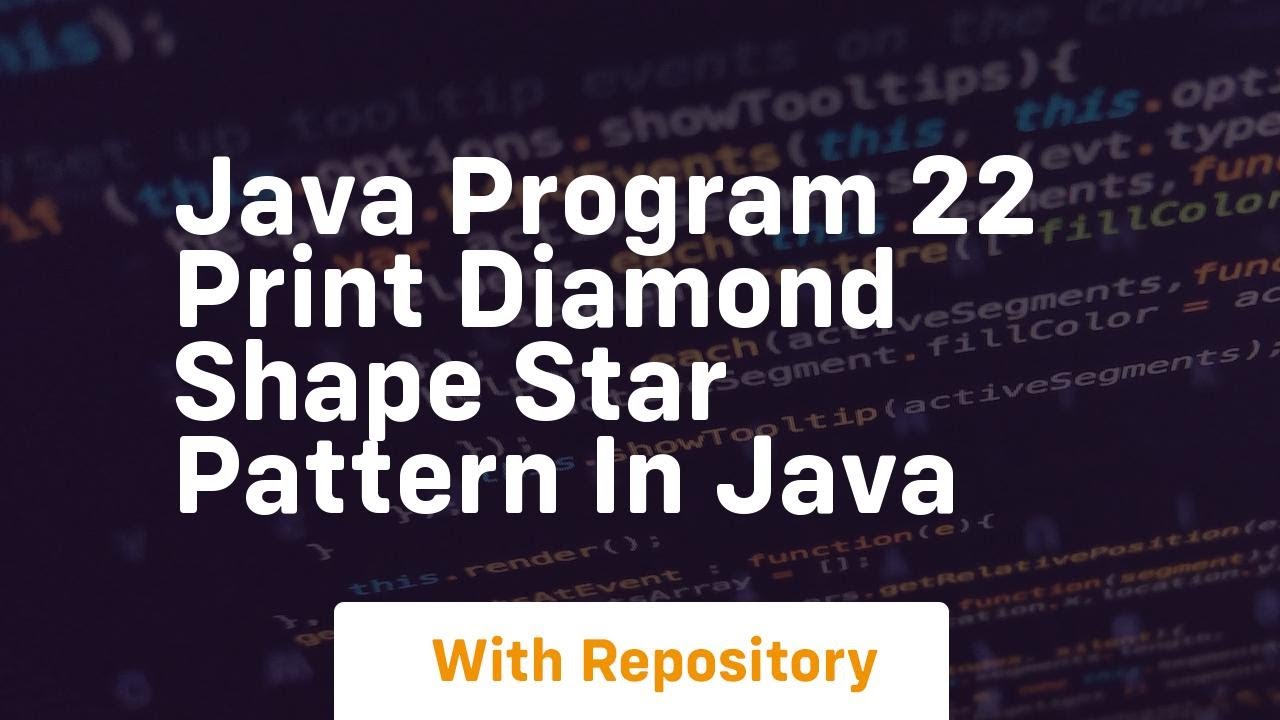
Показать описание
sure! in this tutorial, we'll create a java program that prints a diamond shape star pattern. this is a common exercise that helps you understand loops and nested structures in java.
overview of diamond shape star pattern
a diamond shape star pattern consists of two parts:
1. the upper part (triangle) of the diamond.
2. the lower part (inverted triangle) of the diamond.
for example, given an input of `n = 5`, the output will look like this:
steps to create the diamond pattern
1. **determine the height of the diamond**: the height can be specified as an odd number to make the pattern symmetrical.
2. **print the upper part of the diamond**: use nested loops to print spaces and stars.
3. **print the lower part of the diamond**: similar to the upper part but in reverse order.
java code example
here's a complete java program that prints a diamond shape star pattern:
breakdown of the code
1. **input handling**: we use a `scanner` to get the height of the diamond from the user. we also validate that the number is odd.
2. **upper part of the diamond**:
- we use an outer loop that runs with a step of 2 to print the odd rows (1, 3, 5,...).
- for each row, we first print the required leading spaces and then the stars.
- the number of stars increases by 2 for each subsequent row, while the number of leading spaces decreases.
3. **lower part of the diamond**:
- similar to the upper part, but we start from `n - 2` and decrease by 2 each time.
- the number of leading spaces increases as we go down.
4. **output**: the program prints the diamond shape based on the user's input.
running the program
to run this program:
3. run the compiled program using `java diamondpattern`.
you will be prompted to enter the height of the diamond, and the program will display the diamond pattern accordingly.
conclusion
this exercise demonstrates ho ...
#JavaProgramming #StarPattern #binaryemulation
Java
diamond shape
star pattern
print pattern
nested loops
console output
programming
algorithm
shapes in Java
coding exercises
pattern generation
Java programming
loops in Java
graphical patterns
coding challenges
overview of diamond shape star pattern
a diamond shape star pattern consists of two parts:
1. the upper part (triangle) of the diamond.
2. the lower part (inverted triangle) of the diamond.
for example, given an input of `n = 5`, the output will look like this:
steps to create the diamond pattern
1. **determine the height of the diamond**: the height can be specified as an odd number to make the pattern symmetrical.
2. **print the upper part of the diamond**: use nested loops to print spaces and stars.
3. **print the lower part of the diamond**: similar to the upper part but in reverse order.
java code example
here's a complete java program that prints a diamond shape star pattern:
breakdown of the code
1. **input handling**: we use a `scanner` to get the height of the diamond from the user. we also validate that the number is odd.
2. **upper part of the diamond**:
- we use an outer loop that runs with a step of 2 to print the odd rows (1, 3, 5,...).
- for each row, we first print the required leading spaces and then the stars.
- the number of stars increases by 2 for each subsequent row, while the number of leading spaces decreases.
3. **lower part of the diamond**:
- similar to the upper part, but we start from `n - 2` and decrease by 2 each time.
- the number of leading spaces increases as we go down.
4. **output**: the program prints the diamond shape based on the user's input.
running the program
to run this program:
3. run the compiled program using `java diamondpattern`.
you will be prompted to enter the height of the diamond, and the program will display the diamond pattern accordingly.
conclusion
this exercise demonstrates ho ...
#JavaProgramming #StarPattern #binaryemulation
Java
diamond shape
star pattern
print pattern
nested loops
console output
programming
algorithm
shapes in Java
coding exercises
pattern generation
Java programming
loops in Java
graphical patterns
coding challenges