filmov
tv
JSON Maya Camera Exporter
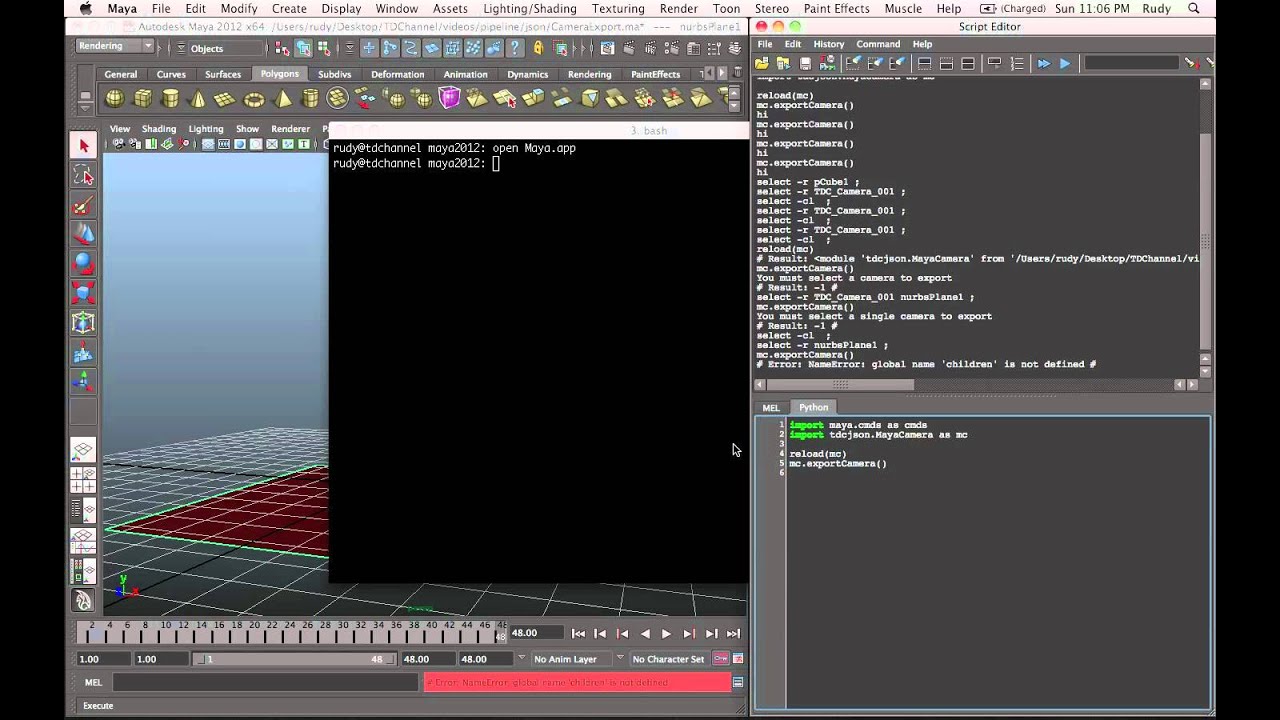
Показать описание
JSON is a very fast and lightweight inter exchange format that can be leveraged to export most data that consists of key value pairs.
This video covers the process of writing a simple camera exporter for Maya. We will cover the process of writing a Python module that can handle the export of the necessary camera data
This video covers the process of writing a simple camera exporter for Maya. We will cover the process of writing a Python module that can handle the export of the necessary camera data
JSON Maya Camera Exporter
Quick tip / How to export a camera from Maya to After effects
Creating an Accurate Camera in Maya with fSpy
Exporting three.js from Maya 2014
Json Export/Import for Character_Customize_Tool(in 3dsMax)
Import After Effects Tracking Data into Blender | No Plugin | Motion Tracking Tutorial
Import Not Appearing in the Viewport Quick Fix in Blender
Camera Align | Maya Script
Export 3d Maya Scene To Webgl
Fspy importer for Maya - Easy camera match
JSON - Blender Camera Importer
JSON Parser for Maya 2015 written in MEL Script
EveryDayMaya: 012: solving import / export wts
Learning THREE.js - 20 -- Blender Full Scene Importer Using JSON Exporter
Unreal Engine 3d track & matte painting
Maya Mel export to After effects. With or without timewarp.
python 8 json files
Your first product VFX In Adobe after effects and Blender
Your First Product VFX with blender and Adobe after effect!
AE Sign Text Test #4 (JSON Export - Locators/Null Objects)
Creating 3D space with correct scale from a single image - part 2
WebGL Exporter for Maya
jab_toAE
Best Way To Export Camera Track From After Effects To Blender | Export After Effects To Blender
Комментарии