filmov
tv
JavaScript Algorithms - 21 - Bubble Sort Solution
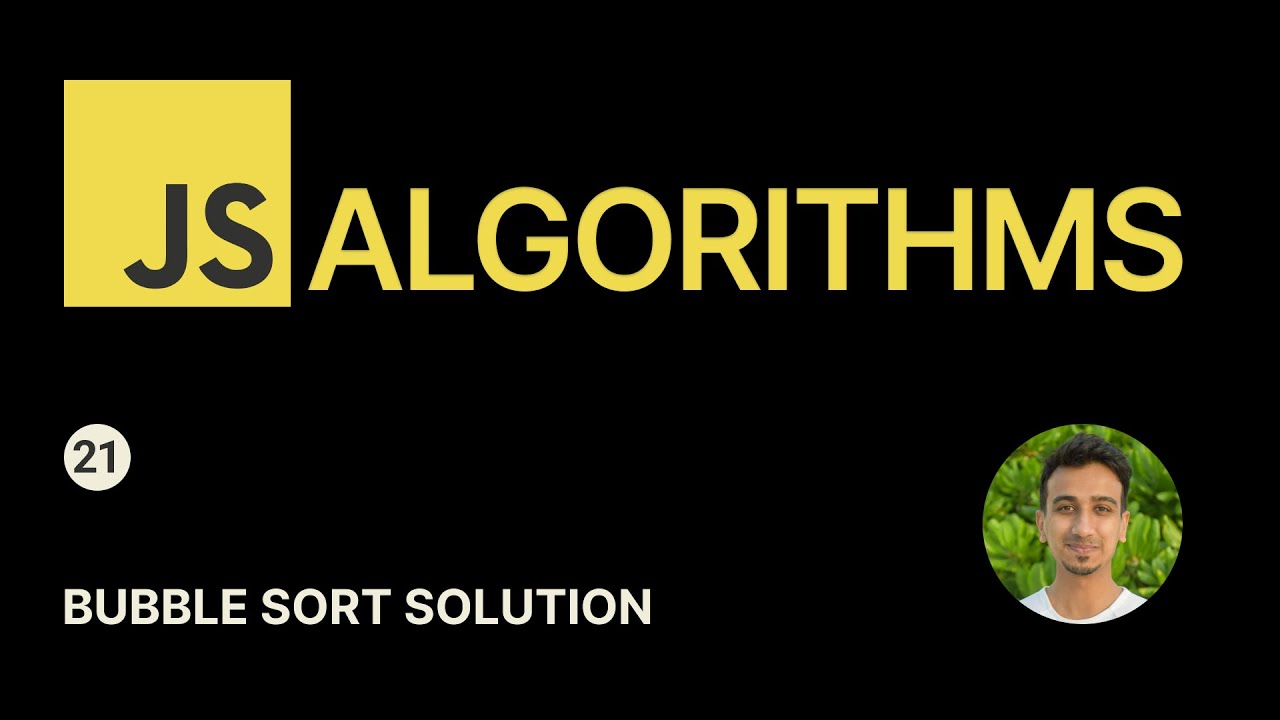
Показать описание
📱 Follow Codevolution
Bubble Sort Solution
JavaScript Algorithms
Algorithms with JavaScript
JavaScript Algorithms - 21 - Bubble Sort Solution
Javascript Freecodecamp Algorithm #21: Map the Debris
Top 10 Javascript Algorithms to Prepare for Coding Interviews
21 Match All Non-Numbers - RegEx - FreeCodeCamp EXPLAINED - JavaScript Algorithms Data FCC Guide
JavaScript Algorithms - 1 Introduction
JavaScript Algorithms - 22 - Insertion Sort
JavaScript Algorithms - 19 - Sorting Algorithms
JavaScript Algorithms - 24 - Quick Sort
How to create Event Emitter in JS | Asked in Meta, Microsoft | JS Coding | DSA in JavaScript
JavaScript Algorithms and Data Structures Masterclass (Part 1)
Data Structures and Algorithms in JavaScript - Full Course for Beginners
JavaScript Algorithms - 6 - Math Algorithms
JavaScript Basic 21: Augmented Addition | FreeCodeCamp |
JavaScript Algorithms - 23 - Insertion Sort Solution
Learn Form Validation by Building a Calorie Counter| STEP 21|JavaScript Algorithms & Data Struct...
Free Code Camp 2019 - Javascript Algorithms & DS Certification - Basic JavaScript Lesson 21
JavaScript Algorithms - 15 - Linear Search
JavaScript Algorithms and Data Structures | #21 - Intermediate Algorithm Scripting (Bagian 2/5)
JavaScript Algorithms - 20 - Bubble Sort
What are Algorithms? | JavaScript Algorithms
JavaScript: 7 String Methods
JavaScript Algorithms - 18 - Recursive Binary Search
Sorting Algorithms Explained Visually
Learn JavaScript Interactively in NEW freeCodeCamp.org Curriculum
Комментарии