filmov
tv
Sorting a HashMap by Values in Java
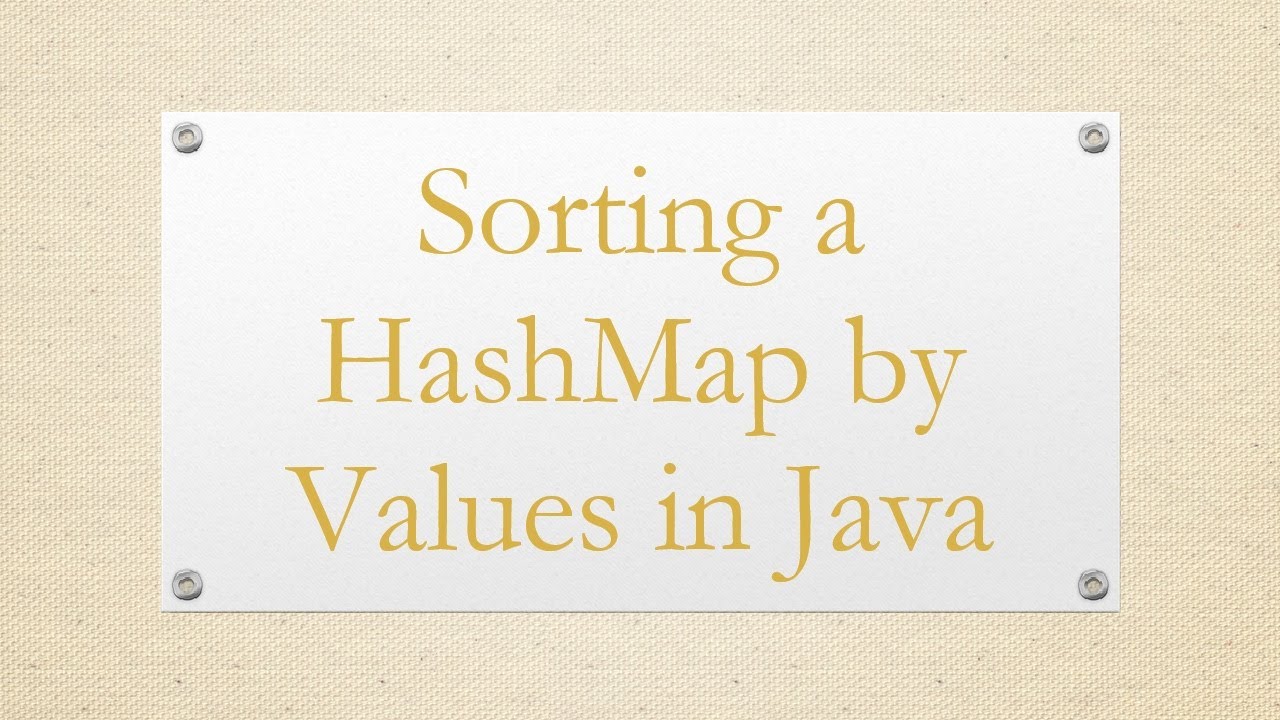
Показать описание
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Summary: Learn how to sort a HashMap by values in Java while maintaining key-value association with effective techniques and code examples.
---
In Java, HashMap is a common data structure that allows you to store key-value pairs. However, one of its limitations is that it does not maintain any specific order of elements. When you need to sort a HashMap by its values while ensuring that the key-value associations remain intact, there are specific methods you can implement in your Java programs. This guide will delve into how to achieve this sorting mechanism, providing code snippets and explanations along the way.
Understanding HashMap
Before diving into sorting methods, it is essential to understand what a HashMap is. It is part of the Java Collections Framework and implements the Map interface. Being a hash table-based implementation, it provides constant-time performance for basic operations such as adding, removing, and accessing elements.
Why Sort a HashMap by Values?
Sorting a HashMap can be useful in various scenarios, such as when you want to display data in a specific order or analyze value-related metrics. While Java's HashMap does not offer built-in support for sorting, you can achieve the desired results by using other data structures such as List or Stream.
How to Sort a HashMap by Values
Method 1: Using a List
Convert the entrySet of the HashMap to a List.
Sort the List using a Comparator that compares the values.
Create a LinkedHashMap to maintain the order.
Here’s how that looks in code:
[[See Video to Reveal this Text or Code Snippet]]
This code initializes a HashMap with keys and values, converts it to a List, sorts the list based on values, and finally maintains the sorted order in a LinkedHashMap.
Method 2: Using Streams (Java 8 and above)
Java 8 introduced the Streams API, which provides a more concise way to sort HashMap entries. You can achieve sorting using the following steps:
Stream the entrySet of the HashMap.
Sort the entries by value.
Collect the results into a LinkedHashMap.
Here’s an example of this approach:
[[See Video to Reveal this Text or Code Snippet]]
This method is compact and takes advantage of the capabilities of the Streams API, making the code cleaner and easier to read.
Conclusion
Sorting a HashMap by values while maintaining key-value associations is achievable in Java using either conventional methods with Lists or the more modern Streams API. By converting entries to a List or using streams, you can effectively organize your data according to the values, which can enhance the readability or usability of your collections in Java.
Understanding these techniques aids in the manipulation of data structures within Java, ensuring that developers can manage key-value pairs flexibly and efficiently.
---
Summary: Learn how to sort a HashMap by values in Java while maintaining key-value association with effective techniques and code examples.
---
In Java, HashMap is a common data structure that allows you to store key-value pairs. However, one of its limitations is that it does not maintain any specific order of elements. When you need to sort a HashMap by its values while ensuring that the key-value associations remain intact, there are specific methods you can implement in your Java programs. This guide will delve into how to achieve this sorting mechanism, providing code snippets and explanations along the way.
Understanding HashMap
Before diving into sorting methods, it is essential to understand what a HashMap is. It is part of the Java Collections Framework and implements the Map interface. Being a hash table-based implementation, it provides constant-time performance for basic operations such as adding, removing, and accessing elements.
Why Sort a HashMap by Values?
Sorting a HashMap can be useful in various scenarios, such as when you want to display data in a specific order or analyze value-related metrics. While Java's HashMap does not offer built-in support for sorting, you can achieve the desired results by using other data structures such as List or Stream.
How to Sort a HashMap by Values
Method 1: Using a List
Convert the entrySet of the HashMap to a List.
Sort the List using a Comparator that compares the values.
Create a LinkedHashMap to maintain the order.
Here’s how that looks in code:
[[See Video to Reveal this Text or Code Snippet]]
This code initializes a HashMap with keys and values, converts it to a List, sorts the list based on values, and finally maintains the sorted order in a LinkedHashMap.
Method 2: Using Streams (Java 8 and above)
Java 8 introduced the Streams API, which provides a more concise way to sort HashMap entries. You can achieve sorting using the following steps:
Stream the entrySet of the HashMap.
Sort the entries by value.
Collect the results into a LinkedHashMap.
Here’s an example of this approach:
[[See Video to Reveal this Text or Code Snippet]]
This method is compact and takes advantage of the capabilities of the Streams API, making the code cleaner and easier to read.
Conclusion
Sorting a HashMap by values while maintaining key-value associations is achievable in Java using either conventional methods with Lists or the more modern Streams API. By converting entries to a List or using streams, you can effectively organize your data according to the values, which can enhance the readability or usability of your collections in Java.
Understanding these techniques aids in the manipulation of data structures within Java, ensuring that developers can manage key-value pairs flexibly and efficiently.