filmov
tv
[Python Programming Basics to Advanced]:Positional Keyword Optional Variable-length Arguments|Lab 27
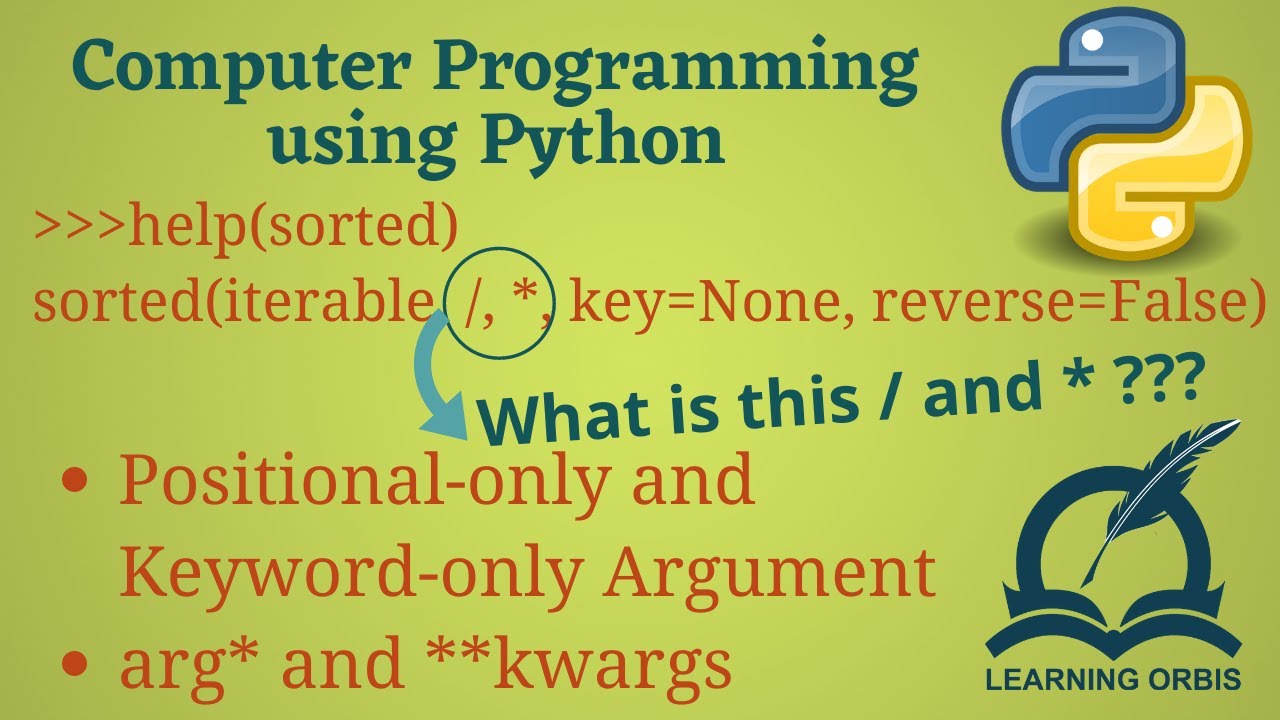
Показать описание
This Python programming playlist is designed to take beginners with zero programming experience to an expert level. The course covers installation, basic syntax, practical scenarios, and efficient logic building. The course material includes PDF handouts, review questions, and covers a wide range of topics, from data types to advanced functions like Lambda and Recursive functions, Generators, and JSON data parsing.
In this lesson we will study about different properties of the input arguments of the functions we can set while defining the function. These include:
- Default value of an Input Argument (Optional input argument)
- Positional-only and Keyword-only Input Arguments
- Variable length Input Arguments
The link for proper format of the Doc String:
Complete Playlist:
If you have the basic programming knowledge and interested to learn Object-Oriented Programming in Python, check out this playlist:
Lab Manual 27 can be downloaded from here:
Review Question:
1- Polygon is a planar closed shape with straight line segments. A polygon can have minimum 3 sides and there is no limit for the maximum sides. If a polygon has n sides, it will have n vertices. A vertex on XY plane can be represented as a Tuple with two values (x and y). As an example a polygon can have five vertices as:
v1=(2,4)
v2=(1,3)
v3=(5,-2)
v4=(3,1)
v5=(6,5)
Your task is to create a user-defined function polyParameter which could take any number of vertices as the input argument and will return the Perimeter of the Polygon. For above case, the function will be used like this:
polyParameter(v1,v2,v3,v4,v5)
To find the parameter you need to find the sum of length of all sides of the polygon. It will be better to create a function dist(v1,v2) which will take two vertices as input argument and returns the length of the line segment.
#PythonProgramming #python #pythontutorial
In this lesson we will study about different properties of the input arguments of the functions we can set while defining the function. These include:
- Default value of an Input Argument (Optional input argument)
- Positional-only and Keyword-only Input Arguments
- Variable length Input Arguments
The link for proper format of the Doc String:
Complete Playlist:
If you have the basic programming knowledge and interested to learn Object-Oriented Programming in Python, check out this playlist:
Lab Manual 27 can be downloaded from here:
Review Question:
1- Polygon is a planar closed shape with straight line segments. A polygon can have minimum 3 sides and there is no limit for the maximum sides. If a polygon has n sides, it will have n vertices. A vertex on XY plane can be represented as a Tuple with two values (x and y). As an example a polygon can have five vertices as:
v1=(2,4)
v2=(1,3)
v3=(5,-2)
v4=(3,1)
v5=(6,5)
Your task is to create a user-defined function polyParameter which could take any number of vertices as the input argument and will return the Perimeter of the Polygon. For above case, the function will be used like this:
polyParameter(v1,v2,v3,v4,v5)
To find the parameter you need to find the sum of length of all sides of the polygon. It will be better to create a function dist(v1,v2) which will take two vertices as input argument and returns the length of the line segment.
#PythonProgramming #python #pythontutorial
Комментарии