filmov
tv
Array Implementation of Stacks (Part 4)
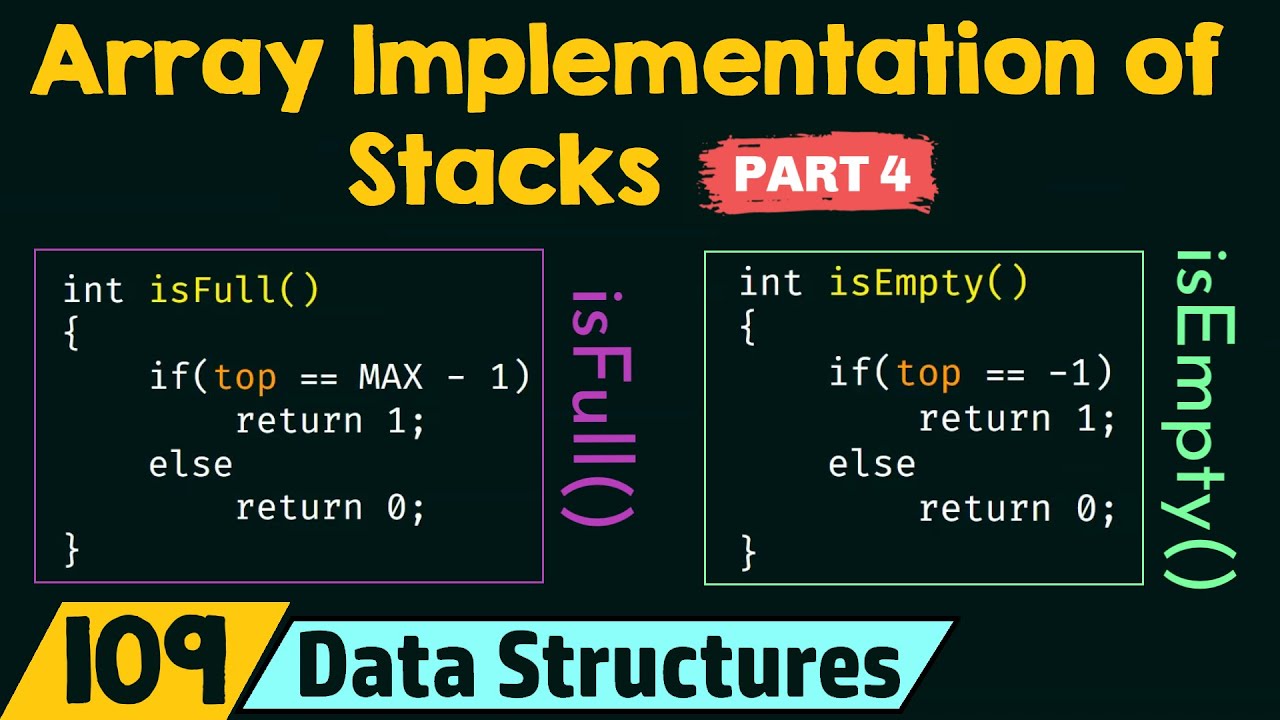
Показать описание
Data Structures: Array Implementation of Stacks (Part 4)
Topics discussed:
1) isFull() function to check if the stack is full or not.
2) isEmpty() function to check if the stack is empty or not.
3) Complete C program for the array implementation of stacks.
Music:
Axol x Alex Skrindo - You [NCS Release]
#DataStructuresByNeso #DataStructures #Stacks #ArrayImplementationOfStacks
Topics discussed:
1) isFull() function to check if the stack is full or not.
2) isEmpty() function to check if the stack is empty or not.
3) Complete C program for the array implementation of stacks.
Music:
Axol x Alex Skrindo - You [NCS Release]
#DataStructuresByNeso #DataStructures #Stacks #ArrayImplementationOfStacks
Array Implementation of Stacks (Part 1)
Array Implementation of Stacks (Part 2)
Array Implementation of Stacks (Part 3)
Array Implementation of Stacks (Part 4)
Data structures: Array implementation of stacks
3.2 Implementation of Stack using Array | Data Structure and Algorithm Tutorials
Implementing Stack Using Array in Data Structures
#10 Stack Implementation using Java Part 1 | Push Pop Peek Methods
Circular Linked List | Insertion at begining | DSA in C with implementation
Linked List Implementation of Stacks (Part 1)
Implementing 3 stacks in a single array
Stack Data Structure in C++ Programming (using arrays) | All Stack Operations | Part - 2
106 Array Implementation of Stacks Part 1
Stacks C++ : Stack Array Implementation
Implementing Four Stacks In One Array
Data Structures using C | Class 6: Stacks and Array Implementation of Stacks - Part 2
108 Array Implementation of Stacks Part 3
109 Array Implementation of Stacks Part 4
Coding Push(), Pop(), isEmpty() and isFull() Operations in Stack Using an Array| C Code For Stack
Implement two stacks in one array (Algorithm)
Array implementation of stacks
107 Array Implementation of Stacks Part 2
Implementing Two Stacks In One Array
Stacks (Program 1) – Part 1
Комментарии