filmov
tv
Building A JSON API In Golang - JWT Authentication part 4
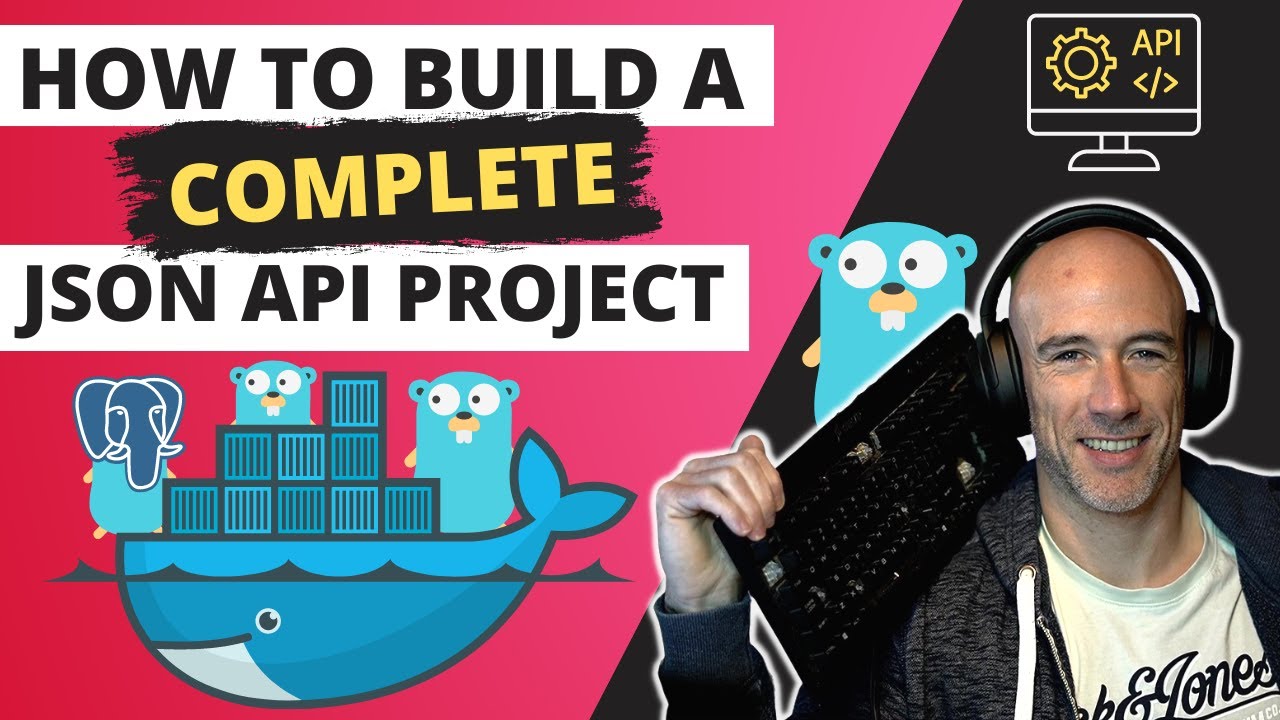
Показать описание
In this Golang programming tutorial series, I will teach you how to build a complete industry-ready JSON API project in Golang with JWT authentication, Postgresql, and Docker. We are going to build a bank API and build everything from scratch with only the mandatory packages we actually need. We are also going to write tests for each of our HTTP handlers. If you want to learn Golang or Rust consider subscribing to my channel so you can become a high-value engineer.
#golang
JSON API: Explained in 4 minutes (+ EXAMPLES)
RESTful APIs in 100 Seconds // Build an API from Scratch with Node.js Express
Creating A Json Api With Spring Boot
Golang API Basics: Building a JSON endpoint 📜💻
HOW TO BUILD A JSON API IN PYTHON
Building A JSON API In Golang - JWT Authentication part 4
Learn JSON in 10 Minutes
How To Build A Complete JSON API In Golang (JWT, Postgres, and Docker) Part 1
Convert Text to JSON: Easy Steps You Must Try
How To Build And Structure A JSON API Project In Golang!?
Creating a JSON CRUD API in Go (Gin/GORM)
How To Visualize JSON Files
6. JSON API specification
Build a JSON database in 5 mins with JavaScript
Building A JSON API In Golang Part 5 - Signing In Users
How to Host API on GitHub || How to Host JSON API on Server 🔥
Build a Mock REST API in Seconds with JSON Server
Create a Fake REST API with JSON-Server
Build a JSON REST API in Node.js- Learn backend development by building a Javascript web service!
How To Build A Complete JSON API In Golang (JWT, Postgres, and Docker) Part 2
🤩😱 Read a Local JSON File in JavaScript using Fetch API #shorts #javascript #programming #api
Post Data with ReactJS & JSON Server in Seconds | Simple API Tutorial #JSON #reactjs #shorts
The BEST way to visualize JSON👩💻 #programming #technology #software #code #data #tech
How To Create a Json Rest Api with SAP Abap
Комментарии