filmov
tv
Dealing with JSONDecodeError: Expecting value in Python
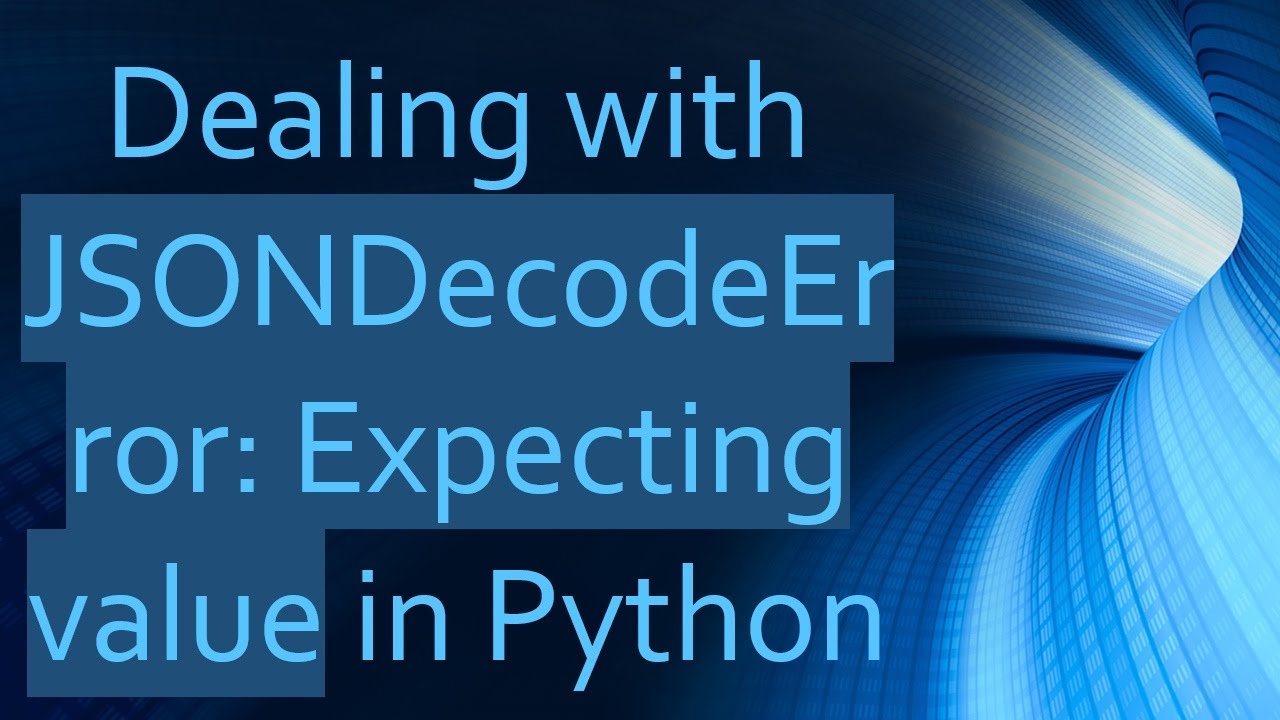
Показать описание
Learn how to handle the common `JSONDecodeError: Expecting value` in Python, understand the root causes, and explore ways to troubleshoot and fix this error.
---
Dealing with JSONDecodeError: Expecting value in Python
When working with Python to process JSON data, it's not uncommon to encounter errors, one of the most frequent being JSONDecodeError: Expecting value. This error typically arises when trying to decode a JSON object from a response or a string. Let’s dive into why this error occurs and how you can address it.
What is JSONDecodeError?
A JSONDecodeError is thrown by Python's json module when it cannot parse the input string as valid JSON. The specific error message Expecting value indicates that the parser expected a value but found none. This often means that the input string is either empty, not properly formatted JSON or the request didn't return the expected JSON response.
Common Causes
Empty Response:
Malformed JSON:
If the response is not a proper JSON format, such as missing quotes, commas, or curly braces, the JSON decoder will not be able to parse it correctly.
Hidden Characters:
There could be unintended characters that aren’t visible in a text editor but are part of the string, causing the decoding to fail.
How to Troubleshoot and Fix
Check Response Content
First, ensure that the response you are processing actually contains JSON data. You can do this by printing or logging the response content before attempting to decode it:
[[See Video to Reveal this Text or Code Snippet]]
Validate JSON Format
If the response is not empty, the next step is to check its format. You can use online JSON validators or tools to confirm if the data structure is correct. For debugging within Python, you can use:
[[See Video to Reveal this Text or Code Snippet]]
Check for Hidden Characters
Ensure there are no hidden or special characters in the string that are causing issues. You can use encoding to filter out irregular characters:
[[See Video to Reveal this Text or Code Snippet]]
Use .json() Method Correctly
If you are working with requests library, it's safer to use the built-in .json() method which simplifies this process but also properly handles content types:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
The JSONDecodeError: Expecting value is a common but fixable issue when dealing with JSON in Python. By ensuring that the response contains proper JSON format and by implementing thorough error handling, you can troubleshoot and resolve these decoding issues effectively.
Understanding the root causes and knowing how to apply the right checks can save significant debugging time and make your Python code more robust. Happy coding!
---
Dealing with JSONDecodeError: Expecting value in Python
When working with Python to process JSON data, it's not uncommon to encounter errors, one of the most frequent being JSONDecodeError: Expecting value. This error typically arises when trying to decode a JSON object from a response or a string. Let’s dive into why this error occurs and how you can address it.
What is JSONDecodeError?
A JSONDecodeError is thrown by Python's json module when it cannot parse the input string as valid JSON. The specific error message Expecting value indicates that the parser expected a value but found none. This often means that the input string is either empty, not properly formatted JSON or the request didn't return the expected JSON response.
Common Causes
Empty Response:
Malformed JSON:
If the response is not a proper JSON format, such as missing quotes, commas, or curly braces, the JSON decoder will not be able to parse it correctly.
Hidden Characters:
There could be unintended characters that aren’t visible in a text editor but are part of the string, causing the decoding to fail.
How to Troubleshoot and Fix
Check Response Content
First, ensure that the response you are processing actually contains JSON data. You can do this by printing or logging the response content before attempting to decode it:
[[See Video to Reveal this Text or Code Snippet]]
Validate JSON Format
If the response is not empty, the next step is to check its format. You can use online JSON validators or tools to confirm if the data structure is correct. For debugging within Python, you can use:
[[See Video to Reveal this Text or Code Snippet]]
Check for Hidden Characters
Ensure there are no hidden or special characters in the string that are causing issues. You can use encoding to filter out irregular characters:
[[See Video to Reveal this Text or Code Snippet]]
Use .json() Method Correctly
If you are working with requests library, it's safer to use the built-in .json() method which simplifies this process but also properly handles content types:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
The JSONDecodeError: Expecting value is a common but fixable issue when dealing with JSON in Python. By ensuring that the response contains proper JSON format and by implementing thorough error handling, you can troubleshoot and resolve these decoding issues effectively.
Understanding the root causes and knowing how to apply the right checks can save significant debugging time and make your Python code more robust. Happy coding!