filmov
tv
Section 4 (Javascript): Lesson 9 - Arrays
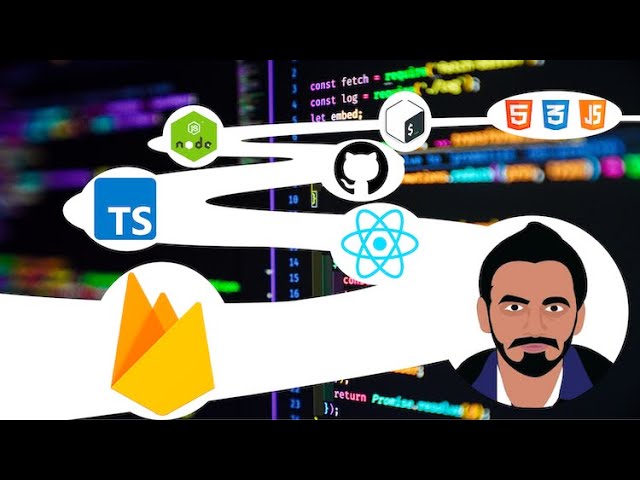
Показать описание
Hey you and welcome.
In this video we will learn about arrays in JavaScript.
So let's dive right into it.
I'm going to open up my co-worker in VS code with a new JavaScript file.
Close the terminal and we're ready to go.
I'll start by instantiating an array.
In order to do so, I'll start with a const name it r equals to square brackets and semicolon.
That's how you instantiate an empty array.
Square brackets mean array.
If I was to replace that with braces, that's object.
So array versus object there.
And inside this array I can provide a list of items and those items can be any data type that you want
and I mean anything.
So I could provide undefined if I'd like a null, a string value, a number, a boolean, I could even
provide an object.
I could provide another array inside an array, I could provide a date object or any other class that
we create.
So array can be of any, any kind.
And of course in JavaScript you could mix them up.
Ideally, you wouldn't really do that.
You would want to keep them as one type, but you are allowed to mix those up.
So I'm going to take all this away and inside the array I'm going to provide names of animals so we
can see how arrays work in JavaScript.
So let's start working with that.
I'll say pig.
Oh, not good.
Okay, let's take that away.
Chicken and horse.
Great.
And let's rename this so we know this is a list of animals.
Great.
Let's start by console logging our animals array.
See what it looks like.
There we go.
Square brackets on each side, string values, comma separated.
That's how it would look in the console.
I can fetch another property of the array, which is the length property animals dot length, which
will tell us how many items are there in the array at the moment, which are three, even though the
item length is three, the animal index for each animal is minus one.
So index for the array always starts with zero.
That can cause a little bit of confusion.
So let me show you what I mean by that.
I'll do a console log animals and using square brackets after animals I can target each item specifically
if I know the number of the item.
So ideally, if you provide the first number, number one, you would expect pig to show up.
However chicken shows up.
The reason why is because pig is at index zero.
Then one is chicken and horse is two.
So if I do that right now, zero is pig, one is chicken and two is horse.
So usually when you want to get the last item in the array, you can just replace the number two with
animals dot length minus one.
So it's always minus one to get the last item in the array.
Perfect.
I can also add another item, so let me just copy paste this and right in the middle I will just add
another item into animals array using animals dot length and this time I will not do minus one because
I do want to target the next the next index that is available to me in this case number four.
And if I do equals to fish.
There we go.
Last item now is fish.
So that's how we can make modifications to our array perfect.
Not only that, arrays are used so commonly in JavaScript that we also have a lot of methods that go
with the array.
So let me show you what I mean by that.
I'll take away some of this code.
Let's start by joining our animals array.
So there is a join function join method, and if I call that join method, you have to provide a string
as an argument.
So I'll start in a string and notice that as soon as I provide an empty string, it joins all my animals.
Pig, chicken, horse without any spaces, without any commas, just joins them together.
So now I can even provide some kind of delimiter to say separate them by comma and you can see commas
appear.
If I put comma space, make it a little bit better, or I can say space pipe, space, you get the idea.
It's just going to convert array into a string.
So and usually used for rendering it out in someplace or maybe putting it in the database.
So that's the join method.
Next, let's take a look at how we can add items to the end of the array.
We did do that just now where we can just say animals dot length and provide another animal fish and
then console log animals, animals dot length minus one.
Give me the last item so you can see how this works just fine.
That's fine.
However, there is a method to make it easier.
Instead of you having to provide animals dot length like this so I can replace this to say animals dot
push.
And push an item here and there we go.
It says fish.
And with this method, what was good is that I can provide a comma separated list of items that I want
to add.
In this video we will learn about arrays in JavaScript.
So let's dive right into it.
I'm going to open up my co-worker in VS code with a new JavaScript file.
Close the terminal and we're ready to go.
I'll start by instantiating an array.
In order to do so, I'll start with a const name it r equals to square brackets and semicolon.
That's how you instantiate an empty array.
Square brackets mean array.
If I was to replace that with braces, that's object.
So array versus object there.
And inside this array I can provide a list of items and those items can be any data type that you want
and I mean anything.
So I could provide undefined if I'd like a null, a string value, a number, a boolean, I could even
provide an object.
I could provide another array inside an array, I could provide a date object or any other class that
we create.
So array can be of any, any kind.
And of course in JavaScript you could mix them up.
Ideally, you wouldn't really do that.
You would want to keep them as one type, but you are allowed to mix those up.
So I'm going to take all this away and inside the array I'm going to provide names of animals so we
can see how arrays work in JavaScript.
So let's start working with that.
I'll say pig.
Oh, not good.
Okay, let's take that away.
Chicken and horse.
Great.
And let's rename this so we know this is a list of animals.
Great.
Let's start by console logging our animals array.
See what it looks like.
There we go.
Square brackets on each side, string values, comma separated.
That's how it would look in the console.
I can fetch another property of the array, which is the length property animals dot length, which
will tell us how many items are there in the array at the moment, which are three, even though the
item length is three, the animal index for each animal is minus one.
So index for the array always starts with zero.
That can cause a little bit of confusion.
So let me show you what I mean by that.
I'll do a console log animals and using square brackets after animals I can target each item specifically
if I know the number of the item.
So ideally, if you provide the first number, number one, you would expect pig to show up.
However chicken shows up.
The reason why is because pig is at index zero.
Then one is chicken and horse is two.
So if I do that right now, zero is pig, one is chicken and two is horse.
So usually when you want to get the last item in the array, you can just replace the number two with
animals dot length minus one.
So it's always minus one to get the last item in the array.
Perfect.
I can also add another item, so let me just copy paste this and right in the middle I will just add
another item into animals array using animals dot length and this time I will not do minus one because
I do want to target the next the next index that is available to me in this case number four.
And if I do equals to fish.
There we go.
Last item now is fish.
So that's how we can make modifications to our array perfect.
Not only that, arrays are used so commonly in JavaScript that we also have a lot of methods that go
with the array.
So let me show you what I mean by that.
I'll take away some of this code.
Let's start by joining our animals array.
So there is a join function join method, and if I call that join method, you have to provide a string
as an argument.
So I'll start in a string and notice that as soon as I provide an empty string, it joins all my animals.
Pig, chicken, horse without any spaces, without any commas, just joins them together.
So now I can even provide some kind of delimiter to say separate them by comma and you can see commas
appear.
If I put comma space, make it a little bit better, or I can say space pipe, space, you get the idea.
It's just going to convert array into a string.
So and usually used for rendering it out in someplace or maybe putting it in the database.
So that's the join method.
Next, let's take a look at how we can add items to the end of the array.
We did do that just now where we can just say animals dot length and provide another animal fish and
then console log animals, animals dot length minus one.
Give me the last item so you can see how this works just fine.
That's fine.
However, there is a method to make it easier.
Instead of you having to provide animals dot length like this so I can replace this to say animals dot
push.
And push an item here and there we go.
It says fish.
And with this method, what was good is that I can provide a comma separated list of items that I want
to add.