filmov
tv
Design Add and Search Words Data Structure | Made Easy | GOOGLE | Leetcode 211
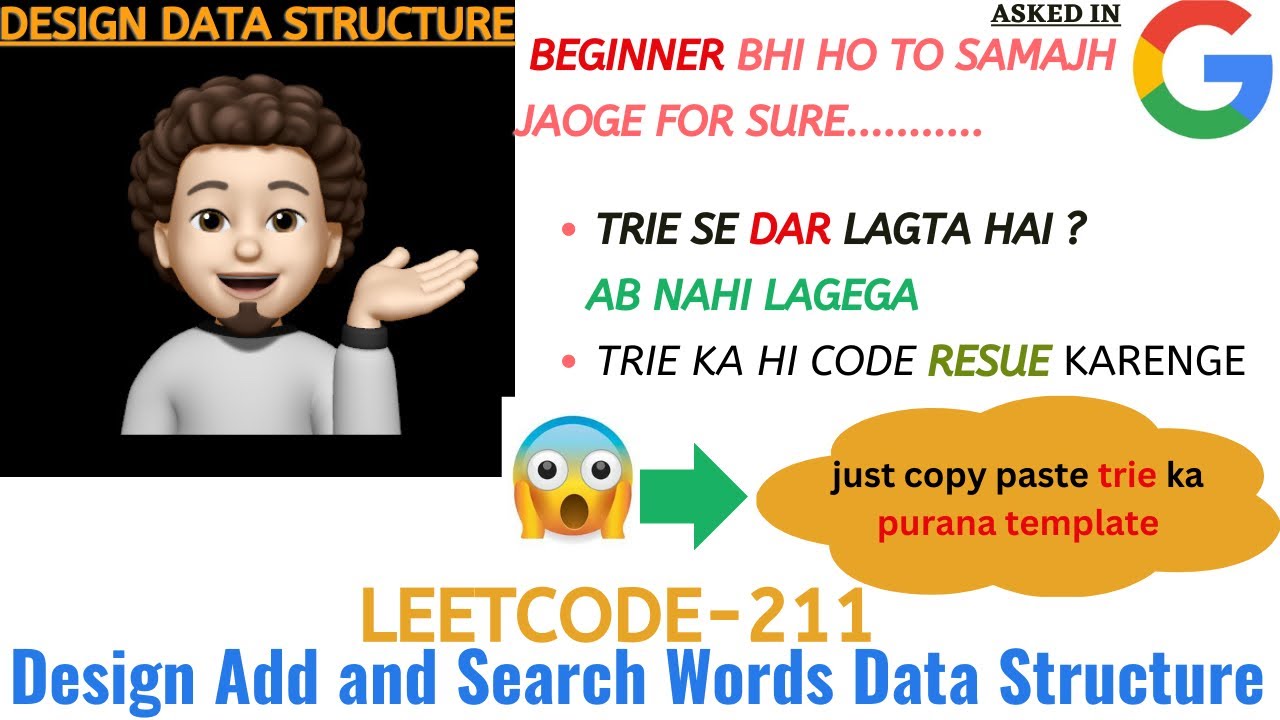
Показать описание
This is the 5th Video of our Design Data Structure Playlist.
In this video we will try to solve a very very good problem "Design Browser History" (Leetcode - 211).
This is actually based on Trie Data Structure.
Share your learnings on LinkedIn, Twitter (X), Instagram, Facebook(Meta) with #codestorywithmik & feel free to tag me.
We will do live coding after explanation and see if we are able to pass all the test cases.
Problem Name : Design Add and Search Words Data Structure
Company Tags : GOOGLE
0:00 - Read the problem
0:44 - Best thing about Complex DS
1:58 - Understand the Problem
4:00 - Why TRIE ? + Template
5:10 - Example Dry Run + Explanation
15:44 - Story To Code Live
╔═╦╗╔╦╗╔═╦═╦╦╦╦╗╔═╗
║╚╣║║║╚╣╚╣╔╣╔╣║╚╣═╣
╠╗║╚╝║║╠╗║╚╣║║║║║═╣
╚═╩══╩═╩═╩═╩╝╚╩═╩═╝
#coding #helpajobseeker #easyrecipes #leetcode #leetcodequestionandanswers #leetcodesolution #leetcodedailychallenge #leetcodequestions #leetcodechallenge #hindi #india #hindiexplanation #hindiexplained #easyexplaination #interview #interviewtips
#interviewpreparation #interview_ds_algo #hinglish
In this video we will try to solve a very very good problem "Design Browser History" (Leetcode - 211).
This is actually based on Trie Data Structure.
Share your learnings on LinkedIn, Twitter (X), Instagram, Facebook(Meta) with #codestorywithmik & feel free to tag me.
We will do live coding after explanation and see if we are able to pass all the test cases.
Problem Name : Design Add and Search Words Data Structure
Company Tags : GOOGLE
0:00 - Read the problem
0:44 - Best thing about Complex DS
1:58 - Understand the Problem
4:00 - Why TRIE ? + Template
5:10 - Example Dry Run + Explanation
15:44 - Story To Code Live
╔═╦╗╔╦╗╔═╦═╦╦╦╦╗╔═╗
║╚╣║║║╚╣╚╣╔╣╔╣║╚╣═╣
╠╗║╚╝║║╠╗║╚╣║║║║║═╣
╚═╩══╩═╩═╩═╩╝╚╩═╩═╝
#coding #helpajobseeker #easyrecipes #leetcode #leetcodequestionandanswers #leetcodesolution #leetcodedailychallenge #leetcodequestions #leetcodechallenge #hindi #india #hindiexplanation #hindiexplained #easyexplaination #interview #interviewtips
#interviewpreparation #interview_ds_algo #hinglish
Design Add and Search Words Data Structure - Leetcode 211 - Python
211. Design Add and Search Words Data Structure - Day 19/31 Leetcode March Challenge
Design Add and Search Word - TRIE | LeetCode 211 | Medium
Add and search word | Data structure design | Trie + Backtracking
Design Add and Search Words Data Structure | LeetCode 211 | Coders Camp
Design Add and Search Words Data Structure | LeetCode 211 | Java
Design Add and Search Words Data Structure-211 TRIE & TREE || Google, apple, Amazon, meta, micro...
Leetcode - Add and Search Word - Data structure design (Python)
Day 40 / 90 days kuch to karna hye series #coding #leetcode #datastructure #tries #problemsolving
Design Add and Search Words Data Structure | Made Easy | GOOGLE | Leetcode 211
Design Add and Search Words Data Structure || Trie Data Structure
Design Add and Search Words Data Structure #leetcode #blind75
Design Add and Search Words Data Structure | Leeetcode 211 | Live coding session 🔥🔥🔥 | Easy Tries...
Design Add and Search Words Data Structure - Leetcode - 211
Design Add and Search Words Data Structure - Leetcode 211 - Python
Design Add and Search Words Data Structure - Trie
Design Add And Search Word Data Structure - LeetCode 211 - Google Interview
211. Design Add and Search Words Data Structure - Day 28/31 Leetcode January Challenge
LeetCode 211 - Design Add and Search Words Data Structure [Daily Challenge]
Google Coding Interview Question | Leetcode 211 | Design Add and Search Words Data Structure
211. Design Add and Search Words Data Structure | JavaScript | LeetCode Daily Challenge
Design Add and Search Words Data Structure | LEETCODE 211 | Solution & Explanation
LeetCode 211 | Design Add and Search Words Data Structure | Java
Design Add and Search Words Data Structure Neetcode150 Series (62 of 150) Leetcode - Second Run
Комментарии