filmov
tv
Longest Word in Dictionary (LeetCode 720. Algorithm Explained)
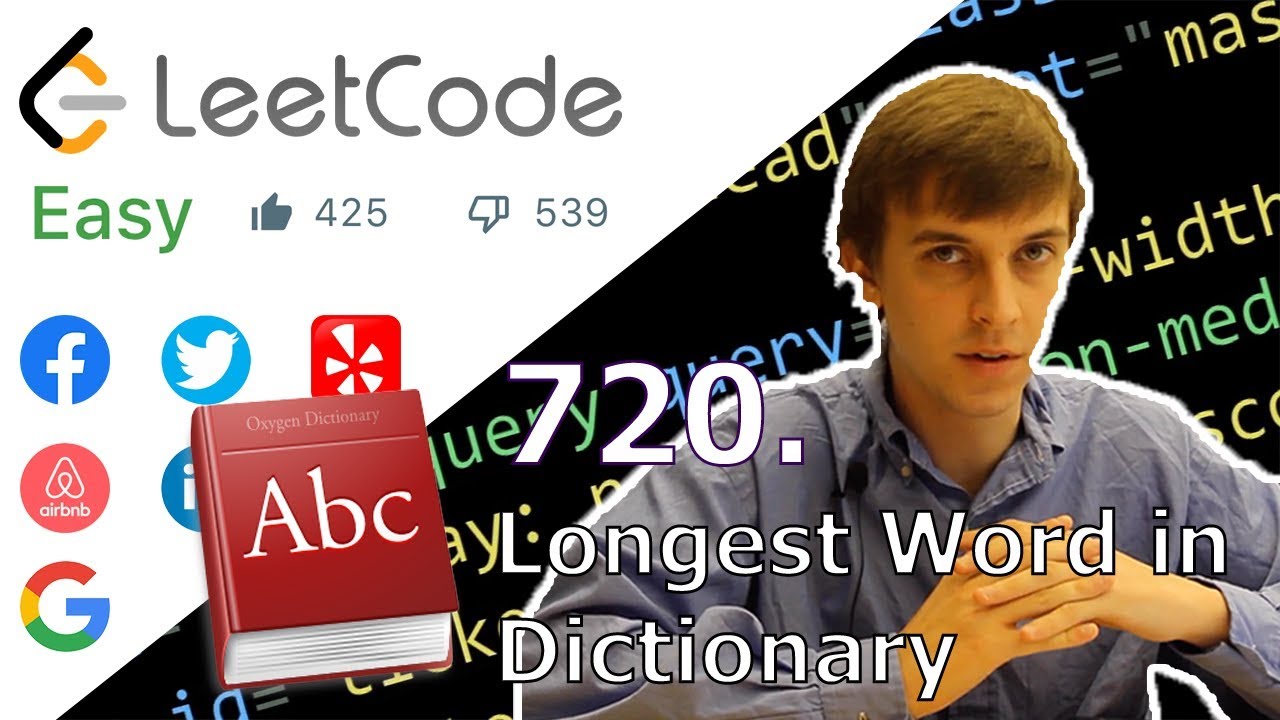
Показать описание
Preparing For Your Coding Interviews? Use These Resources
————————————————————
Other Social Media
----------------------------------------------
Show Support
------------------------------------------------------------------------------
#coding #programming #softwareengineering
Longest Word in Dictionary (LeetCode 720. Algorithm Explained)
Leetcode 720 - Trie | Longest Word Dictionary | Advanced Algorithm
Leetcode 720 Longest Word in Dictionary
Leetcode 720. Longest Word in Dictionary (Trie)
Longest Word in Dictionary through Deleting | Live Coding with Explanation | Leetcode - 524
Longest Word in Dictionary | Leetcode 720
Leetcode - Longest Word in Dictionary through Deleting (Python)
Leetcode 524 Longest Word in Dictionary through Deleting - 2 Pointers Approach
Longest Word in Dictionary through Deleting | LeetCode 524 | Stirng
Leetcode 524: Longest Word in Dictionary through Deleting
Longest Word in Dictionary JAVA SCRIPT(LeetCode 720. Algorithm Explained)|JavaScript
#LeetCode 720 | Longest Word in Dictionary #Java
LeetCode in Golang - Longest Word in Dictionary through Deleting
LONGEST WORD IN DICTIONARY THROUGH DELETING | LEETCODE EXPLORE | DAY 22
524. Longest Word in Dictionary through Deleting (LeetCode)
524 Longest Word in Dictionary through Deleting | Leetcode daily challenge | FAANG interviews
LeetCode 524. Longest Word in Dictionary through Deleting (Python)
LeetCode by Algorithms - 524 Longest Word in Dictionary through Deleting
Longest Word in Dictionary through Deleting | LeetCode 524 | Coders Camp
524. Longest Word in Dictionary through Deleting (Leetcode Medium)
524. Longest Word in Dictionary through Deleting - Day 22/28 Leetcode February Challenge
91. Leetcode 720 Longest Word in Dictionary
Longest Word in Dictionary through Deleting | February LeetCode Challenge
leetcode 524 Longest Word in Dictionary through Deleting
Комментарии