filmov
tv
Java Multithreaded Part 5 - Cyclic Barrier
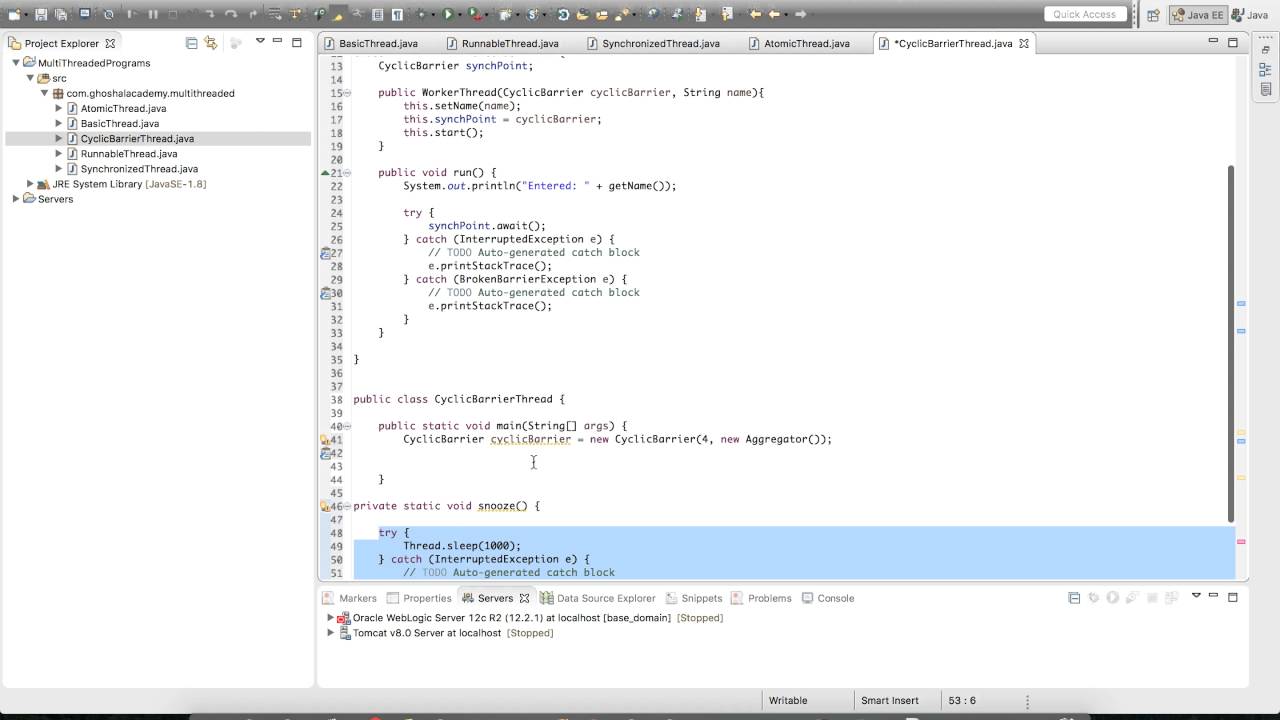
Показать описание
How to spawn multiple threads and then wait at an execution point for all the threads using CyclicBarrier
Java Multithreaded Part 5 - Cyclic Barrier
Advanced Java: Multi-threading Part 5 -- Thread Pools
Sleep Method in Java Multithreading | Multithreading Part - 5
Threading (Part 5-A): Synchronization-free multithreaded program
Core Java with OCJP/SCJP: Multi Threading Part-5 || yield() || join()
Multithreading in Java [Part 5] : Executor Service (Real Life Example)
Core Java-Multi-Threading-Synchronized Limitations-Part 5
Core Java-Multi-Threading-How to Define a Thread? || Part 5
String Implementation Problem 1 | Java Fundamentals | Bangla | Part 5
Core Java With OCJP/SCJP: Multithreading Enhancement Part- 5|| java thread local
Core Java-Multi-Threading-Thread Group-Part 5
Core Java-Multi-Threading-Synchronization Scenario-Part 5
Multi Threading Part-5 | Multi thread Multi thread task | By Extending Thread class
#52 Threads in Java | In Tamil | Java Tutorial Series | EMC
Core Java-Multi-Threading-Inter Thread Communication-Part 5
29. Multithreading and Concurrency in Java: Part1 | Threads, Process and their Memory Model in depth
Multithreading in Java Explained in 10 Minutes
How can you start a Thread? - Cracking the Java Coding Interview
Java Multithreading: Synchronization, Locks, Executors, Deadlock, CountdownLatch & CompletableFu...
yield( ) || yield method in java || Thread class methods in java part5
Java Multithreading Tutorial for Beginners #6: Thread Status and Join
Part 05 - Concurrency - Scheduling Tasks (Multithreading in Java) Java Certification 1Z0-819
Multithreading in C# NET Part 5 | C#.NET Tutorial | Mr. Bangar Raju
Java ExecutorService - Part 1 - Introduction
Комментарии