filmov
tv
JavaScript Algorithms - 12 - Recursive Fibonacci Sequence
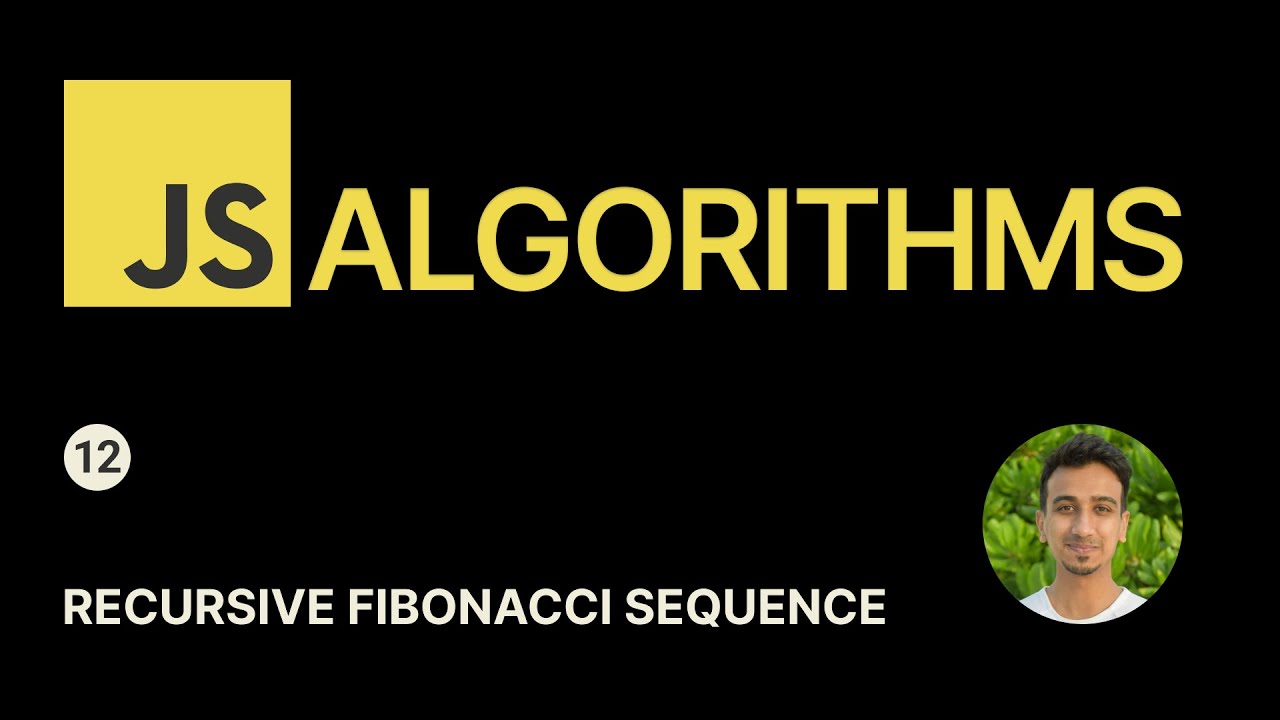
Показать описание
📱 Follow Codevolution
Recursive Fibonacci Sequence
JavaScript Algorithms
Algorithms with JavaScript
JavaScript Algorithms - 12 - Recursive Fibonacci Sequence
Top 10 Javascript Algorithms to Prepare for Coding Interviews
JavaScript Algorithms - 13 - Recursive Factorial of a Number
Learn Javascript with me #12 | JavaScript Algorithms and Data Structures Free Code Camp course
ALL IN ONE: Data Structures & Algorithms In JavaScript Complete Course 2024 By HuXn
Learn Form Validation by Building a Calorie Counter| STEP 12|JavaScript Algorithms & Data Struct...
Javascript Freecodecamp Algorithm #12: Sum All Odd Fibonacci Numbers
JS Checkpoint 12 - Do you know this Javascript method?
Learn the Date Object by Building a Date Formatter| Step 12 |JavaScript Algorithms & Data Struct...
Should I learn DSA with JavaScript
JavaScript Algorithms - 1 - Introduction
Basic Javascript | Subtract One Number from Another with JavaScript | freeCodeCamp | 12 of 113
JavaScript Algorithms - 4 - Big-O Notation
Debugging - JavaScript Algorithms and Data Structures
Learn JavaScript - Full Course for Beginners
Lesson 12 | Basic JavaScript | Subtract One Number from Another with JavaScript
JavaScript Algorithms - 11 - Recursion
It’s literally perfect 🫠 #coding #java #programmer #computer #python
Day-12: JavaScript Algorithms and Data Structures
Leetcode Interviews
Coding - Expectation vs Reality | Programming - Expectation vs Reality | Codeiyapa #Shorts
Easiest Programming language to start with to earn money
New Local Scopes Syntax in Laravel 12.x #laravel
freeCodeCamp - Pt29 JavaScript Algorithms and Data Structures | Sept 12 24 Study Session 1
Комментарии