filmov
tv
How to Properly Wait for Data in JavaScript: Ensuring global.lastVideo Is Defined
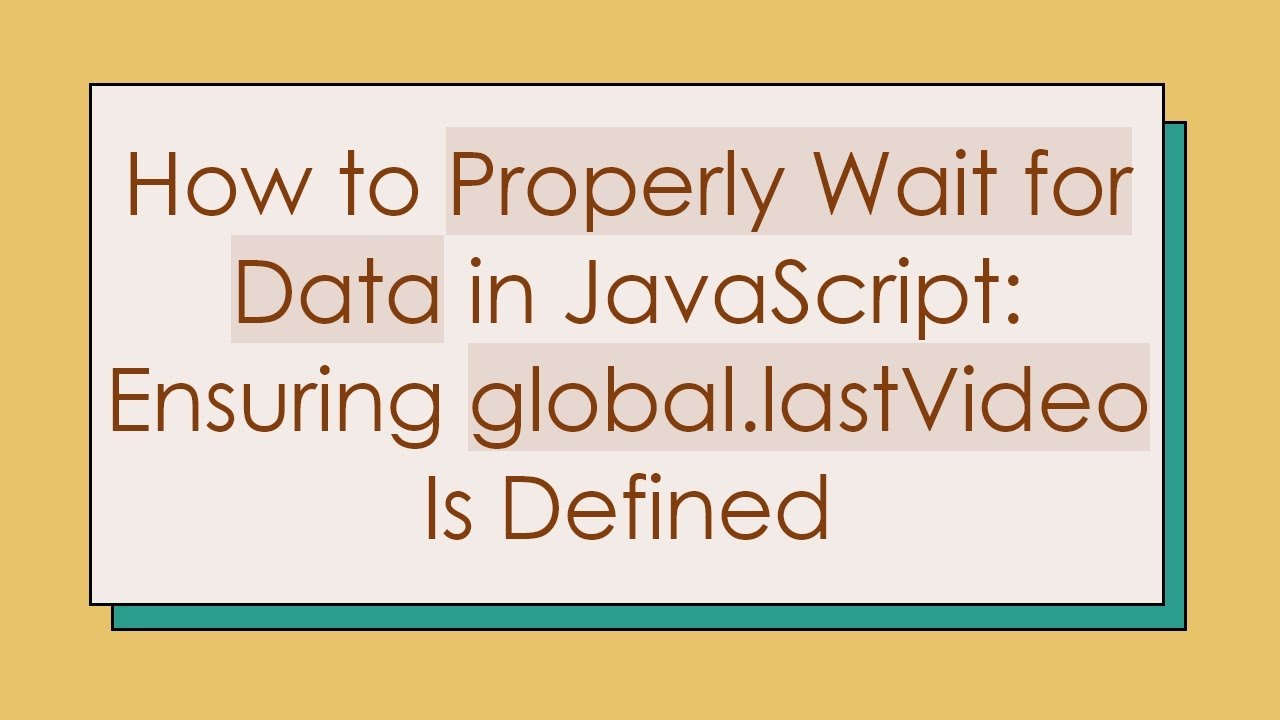
Показать описание
Discover how to handle asynchronous data fetching in JavaScript, ensuring that your functions wait for data before proceeding.
---
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
The Problem: Undefined Values from Asynchronous Operations
You might find yourself in a scenario where you're trying to fetch the latest video from a YouTube channel but end up with an undefined value. Here's a simplified version of the function you might be using:
[[See Video to Reveal this Text or Code Snippet]]
What's Going Wrong?
The Solution: Using Promises and Async/Await
To effectively handle asynchronous data fetching and ensure your code waits for the data to arrive, you can leverage JavaScript promises or the more modern async/await syntax. Let's break down both approaches.
Option 1: Using Promises
You can modify the getLastVideo function to return the promise directly, ensuring that any logic dependent on the result of the fetch occurs only once the data is available.
Here's the updated code:
[[See Video to Reveal this Text or Code Snippet]]
Explanation:
By returning the promise from parseURL, you allow the caller of getLastVideo to handle the data only after it has been retrieved.
The then() method ensures that you have access to the data once it resolves.
Option 2: Using Async/Await
If you prefer more readable and synchronous-like code, you can use async/await. Here's how you can rewrite your function:
[[See Video to Reveal this Text or Code Snippet]]
Explanation:
The async keyword allows you to use await inside the function, which makes the code wait for the promise from parseURL to resolve.
This approach is generally considered cleaner and more intuitive than chaining .then() calls.
Conclusion
Next time you face asynchronous operations in JavaScript, remember to implement one of these techniques to ensure your data is ready before you proceed!
---
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
The Problem: Undefined Values from Asynchronous Operations
You might find yourself in a scenario where you're trying to fetch the latest video from a YouTube channel but end up with an undefined value. Here's a simplified version of the function you might be using:
[[See Video to Reveal this Text or Code Snippet]]
What's Going Wrong?
The Solution: Using Promises and Async/Await
To effectively handle asynchronous data fetching and ensure your code waits for the data to arrive, you can leverage JavaScript promises or the more modern async/await syntax. Let's break down both approaches.
Option 1: Using Promises
You can modify the getLastVideo function to return the promise directly, ensuring that any logic dependent on the result of the fetch occurs only once the data is available.
Here's the updated code:
[[See Video to Reveal this Text or Code Snippet]]
Explanation:
By returning the promise from parseURL, you allow the caller of getLastVideo to handle the data only after it has been retrieved.
The then() method ensures that you have access to the data once it resolves.
Option 2: Using Async/Await
If you prefer more readable and synchronous-like code, you can use async/await. Here's how you can rewrite your function:
[[See Video to Reveal this Text or Code Snippet]]
Explanation:
The async keyword allows you to use await inside the function, which makes the code wait for the promise from parseURL to resolve.
This approach is generally considered cleaner and more intuitive than chaining .then() calls.
Conclusion
Next time you face asynchronous operations in JavaScript, remember to implement one of these techniques to ensure your data is ready before you proceed!