filmov
tv
Understanding JavaScript: Pass by Reference or Pass by Value
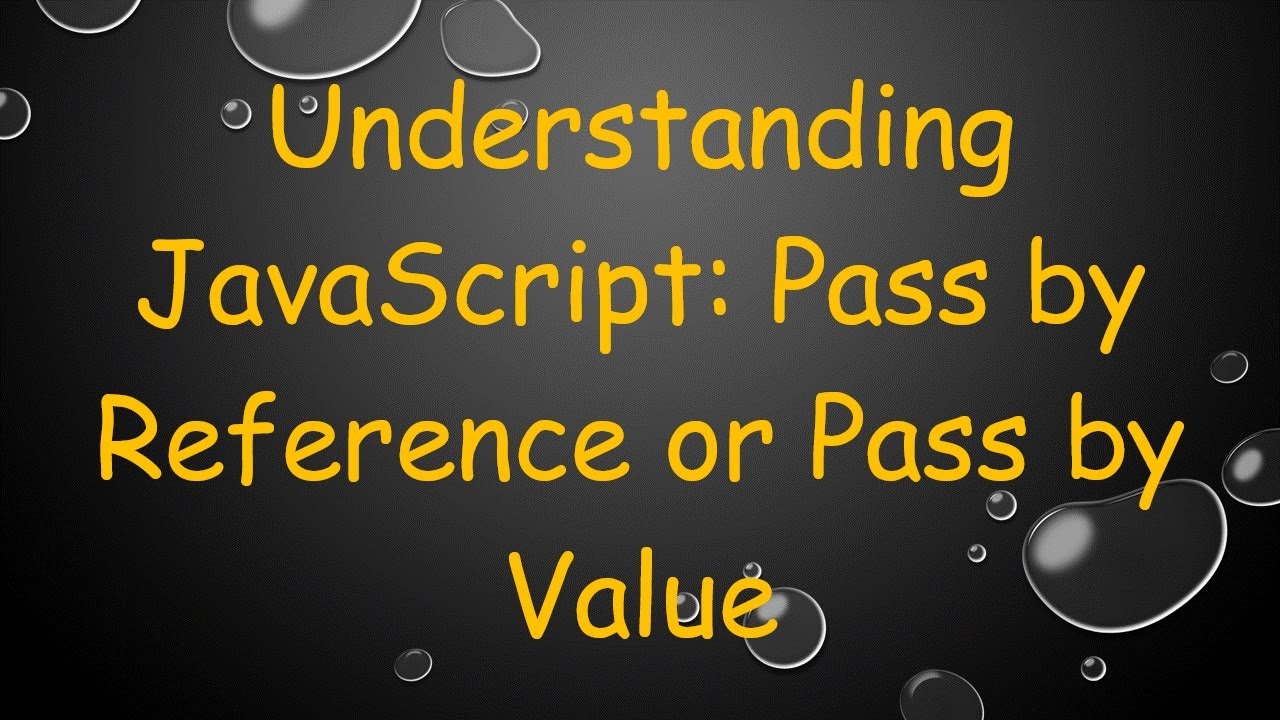
Показать описание
Explore whether JavaScript is a pass by reference or pass by value language. Understand how JavaScript handles variable assignments and function arguments.
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Understanding JavaScript: Pass by Reference or Pass by Value
When diving into JavaScript, one commonly asked question is whether the language utilizes "pass by reference" or "pass by value" semantics. This concept is essential, especially when dealing with variable assignments and function calls. Grasping this will better equip you to debug and write more predictable, error-free code.
Pass by Value
In JavaScript, primitive data types such as numbers, strings, boolean values, undefined, null, and symbols are passed by value. This means that when you assign a primitive value to a variable or pass it as a function argument, a copy of that value is created.
Here’s a simple example:
[[See Video to Reveal this Text or Code Snippet]]
In this example, modifying b does not affect a because b is just a copy of the value of a.
Pass by Reference
In contrast, complex data types like objects and arrays are passed by reference. This means that when you assign an object or an array to a variable, or pass it as an argument to a function, what gets copied is a reference to that object, not the actual value.
Consider the following code:
[[See Video to Reveal this Text or Code Snippet]]
Here, modifying obj2 also modifies obj1 since both obj1 and obj2 reference the same object in memory.
Function Arguments
Understanding how JavaScript handles function arguments is crucial. For primitive types, function arguments are passed by value:
[[See Video to Reveal this Text or Code Snippet]]
For objects and arrays, function arguments are passed by reference:
[[See Video to Reveal this Text or Code Snippet]]
In this example, the function modifyObject modifies the original object because the function received a reference to myObj.
Conclusion
To summarize, JavaScript is a language that primarily passes primitive data types by value and complex data structures (objects and arrays) by reference. Understanding this distinction is pivotal for avoiding unintended mutations and writing more predictable code. By being mindful of these nuances, developers can leverage JavaScript more effectively to build robust applications.
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Understanding JavaScript: Pass by Reference or Pass by Value
When diving into JavaScript, one commonly asked question is whether the language utilizes "pass by reference" or "pass by value" semantics. This concept is essential, especially when dealing with variable assignments and function calls. Grasping this will better equip you to debug and write more predictable, error-free code.
Pass by Value
In JavaScript, primitive data types such as numbers, strings, boolean values, undefined, null, and symbols are passed by value. This means that when you assign a primitive value to a variable or pass it as a function argument, a copy of that value is created.
Here’s a simple example:
[[See Video to Reveal this Text or Code Snippet]]
In this example, modifying b does not affect a because b is just a copy of the value of a.
Pass by Reference
In contrast, complex data types like objects and arrays are passed by reference. This means that when you assign an object or an array to a variable, or pass it as an argument to a function, what gets copied is a reference to that object, not the actual value.
Consider the following code:
[[See Video to Reveal this Text or Code Snippet]]
Here, modifying obj2 also modifies obj1 since both obj1 and obj2 reference the same object in memory.
Function Arguments
Understanding how JavaScript handles function arguments is crucial. For primitive types, function arguments are passed by value:
[[See Video to Reveal this Text or Code Snippet]]
For objects and arrays, function arguments are passed by reference:
[[See Video to Reveal this Text or Code Snippet]]
In this example, the function modifyObject modifies the original object because the function received a reference to myObj.
Conclusion
To summarize, JavaScript is a language that primarily passes primitive data types by value and complex data structures (objects and arrays) by reference. Understanding this distinction is pivotal for avoiding unintended mutations and writing more predictable code. By being mindful of these nuances, developers can leverage JavaScript more effectively to build robust applications.