filmov
tv
Given a byte, swap the two nibbles in it
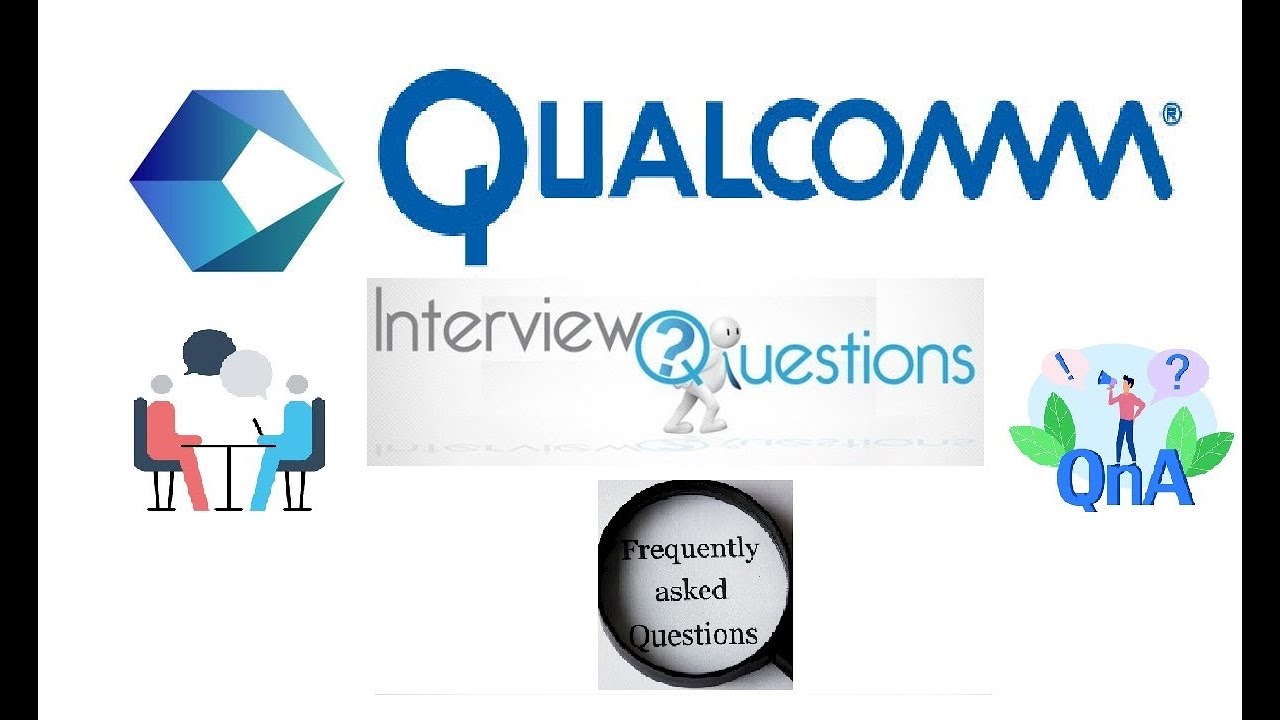
Показать описание
In this video, will discuss different approaches to swap the two nibbles in a byte. Will also discuss optimized approach, their time and space complexity.
A nibble is a four-bit aggregation, or half an octet. There are two nibbles in a byte. For example, 100 is be represented as 01100100 in a byte (or 8 bits). The two nibbles are (0110) and (0100). If we swap the two nibbles, we get 01000110 which is 70 in decimal.
#QualcommTechnicalInterview
#Swap2Nibbles
#CScode
#Programming
#BitManipulation
#SDEngineer
#CnC++
#Placements
A nibble is a four-bit aggregation, or half an octet. There are two nibbles in a byte. For example, 100 is be represented as 01100100 in a byte (or 8 bits). The two nibbles are (0110) and (0100). If we swap the two nibbles, we get 01000110 which is 70 in decimal.
#QualcommTechnicalInterview
#Swap2Nibbles
#CScode
#Programming
#BitManipulation
#SDEngineer
#CnC++
#Placements
Given a byte, swap the two nibbles in it
POTD- 31/05/2024 | Swap two nibbles in a byte | Problem of the Day | GeeksforGeeks Practice
4 Big Endian vs Little Endian Byte Ordering
Program to Swap two nibbles in a byte | C Programming Language
BYTESWAP - ВОТ И ВСЁ! Сайта Байтсвап больше нет. Надежду Нейман нужно привлекать....
Swapping in Java | How to swap nibbles in a byte | Java Programs | interview questions and answers
22 Swapping Nibbles in a Byte: using c
Swap Lower & Upper Byte in Mitsubishi PLC
Modbus Byte Swapping
A C Programming question on swapping nibbles in a byte
Java Quick Byte: Swap Two Values in Seconds! 💻🔄 #viral #shorts
Swap two nibbles in a byte | GFG POTD | GeeksForGeeks
Swap two nibbles in a byte | GFG POTD | Bit Manipulation | CPP | AlgoBazaar
Byteswap разоблачение. Metago = Byteswap.
Byteswap-huijaus – Näin tunnistat huijaussivun!
C bitwise operators 🔣
Swapping two bits of a byte using c program
GFG-POTD | Swap two nibbles in a byte using Java | Bit Magic | Data Structures | 31 May 2024
💡 The Innovation Core of #ByteSwap📌
Мошенники byteswap отзывы и проверка, кинут?
🚀 Don't miss this opportunity, act now and become a ByteSwap VIP! ✨
💡 ByteSwap Hackathons! 💡
C++ : Byte swap during copy
Swap two nibbles in a byte gfg problem
Комментарии