filmov
tv
C++: How to Deduce Template Types from a Singleton Instance
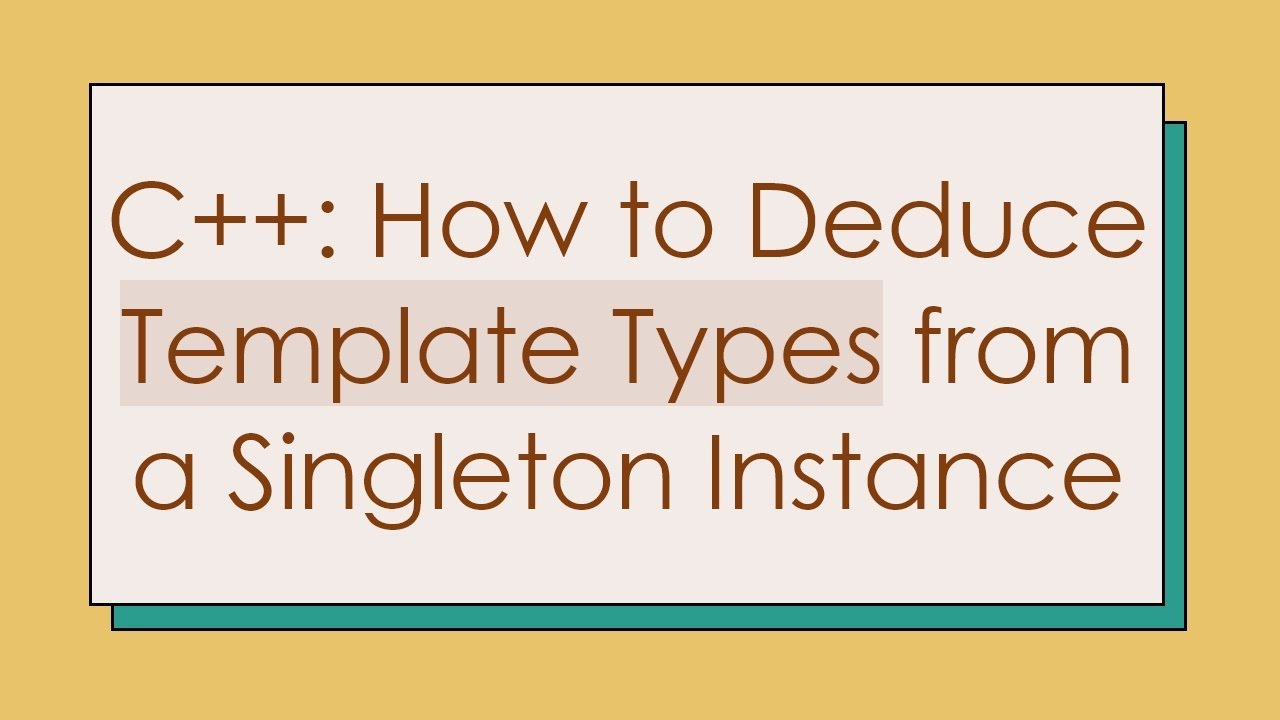
Показать описание
Summary: Learn how to deduce template types from a singleton instance in C++ without making your classes templated, ensuring simpler and more maintainable code.
---
C++: How to Deduce Template Types from a Singleton Instance
Working with templates in C++ can often present unique challenges, especially when it comes to deducing template types from singleton instances without making the entire class a template. This guide aims to shed light on how you can accomplish this with streamlined and efficient code.
The Context and the Challenge
In C++ programming, templates are a powerful feature that allow you to write generic and reusable code. However, deducing the correct template type when dealing with singleton instances can be tricky, especially if you want to avoid the overhead of making your entire class templated.
Singleton design patterns ensure that a class has only one instance and provide a global point of access to it. Integrating templates with singletons, while maintaining flexibility and avoiding templated classes, requires a careful approach.
The Solution
One effective method to deduce template types from a singleton instance without templating your classes is through type traits and type inference. Here's a step-by-step example of how you can achieve this.
Step-by-Step Example
Define a Singleton Class: Start by defining a singleton class.
[[See Video to Reveal this Text or Code Snippet]]
Utility Structure to Deduce Type: Use a utility structure to deduce the template type.
[[See Video to Reveal this Text or Code Snippet]]
Function to Deduce Type: Create a function that uses decltype and the utility structure to infer the type.
[[See Video to Reveal this Text or Code Snippet]]
Main Function to Test: Write a main function to test the template type deduction.
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
By leveraging type traits and type inference, you can successfully deduce template types from a singleton instance without modifying the singleton class itself to be templated. This approach keeps your codebase simpler and more maintainable, without sacrificing the power of C++ templates.
Experiment with this pattern in your projects to see its benefits in various scenarios, whether it's reducing redundancy or simplifying type management in complex codebases.
We hope this article has provided clear insights into deducing template types from singleton instances. As you grow in your C++ journey, mastering these advanced techniques can significantly enhance your ability to write clean and efficient code.
---
C++: How to Deduce Template Types from a Singleton Instance
Working with templates in C++ can often present unique challenges, especially when it comes to deducing template types from singleton instances without making the entire class a template. This guide aims to shed light on how you can accomplish this with streamlined and efficient code.
The Context and the Challenge
In C++ programming, templates are a powerful feature that allow you to write generic and reusable code. However, deducing the correct template type when dealing with singleton instances can be tricky, especially if you want to avoid the overhead of making your entire class templated.
Singleton design patterns ensure that a class has only one instance and provide a global point of access to it. Integrating templates with singletons, while maintaining flexibility and avoiding templated classes, requires a careful approach.
The Solution
One effective method to deduce template types from a singleton instance without templating your classes is through type traits and type inference. Here's a step-by-step example of how you can achieve this.
Step-by-Step Example
Define a Singleton Class: Start by defining a singleton class.
[[See Video to Reveal this Text or Code Snippet]]
Utility Structure to Deduce Type: Use a utility structure to deduce the template type.
[[See Video to Reveal this Text or Code Snippet]]
Function to Deduce Type: Create a function that uses decltype and the utility structure to infer the type.
[[See Video to Reveal this Text or Code Snippet]]
Main Function to Test: Write a main function to test the template type deduction.
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
By leveraging type traits and type inference, you can successfully deduce template types from a singleton instance without modifying the singleton class itself to be templated. This approach keeps your codebase simpler and more maintainable, without sacrificing the power of C++ templates.
Experiment with this pattern in your projects to see its benefits in various scenarios, whether it's reducing redundancy or simplifying type management in complex codebases.
We hope this article has provided clear insights into deducing template types from singleton instances. As you grow in your C++ journey, mastering these advanced techniques can significantly enhance your ability to write clean and efficient code.