filmov
tv
Understanding and Fixing the TypeError in Python: Concatenating Strings with Tuples
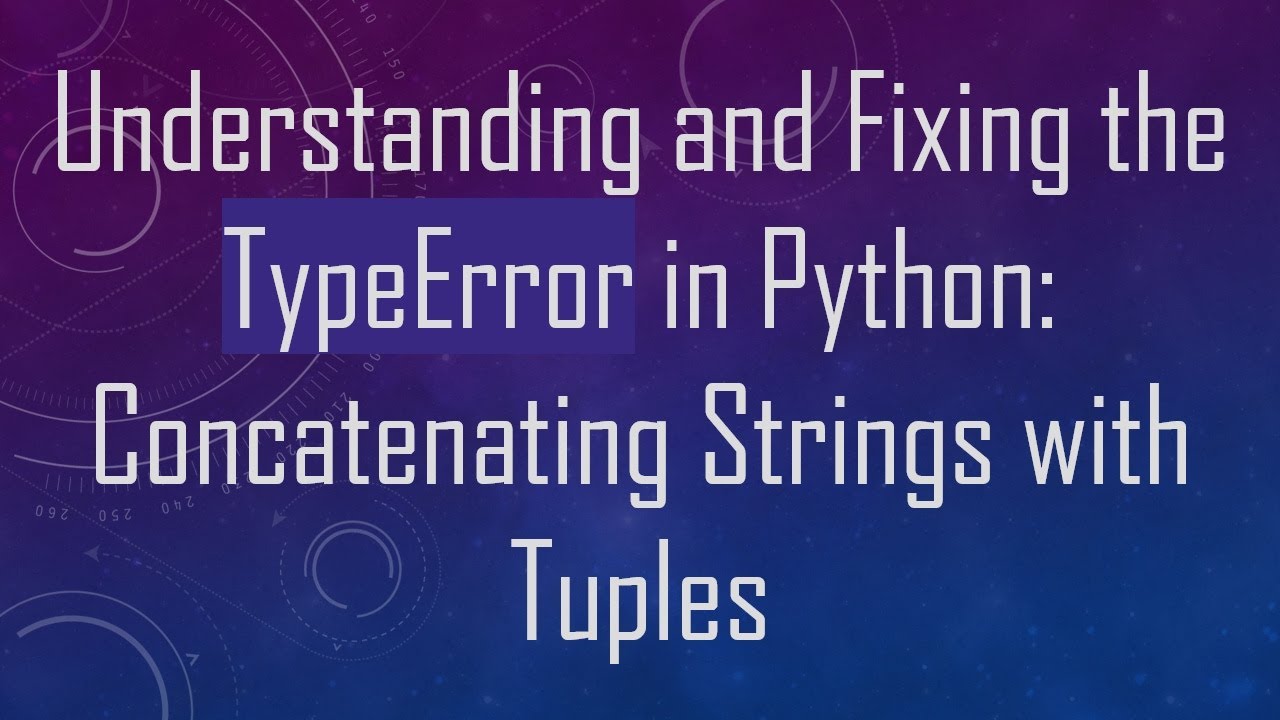
Показать описание
Discover how to resolve the `TypeError` in Python when looping through a dictionary. Learn step-by-step solutions to avoid common mistakes in string concatenation.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Type error: can only concatenate str (not "tuple") to str when looping through dictionary
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding and Fixing the TypeError in Python: Concatenating Strings with Tuples
When working with Python dictionaries, you might encounter a common error that begs an understanding of how to properly handle data types. One such error is the dreaded TypeError: can only concatenate str (not "tuple") to str, which typically arises when attempting to loop through a dictionary’s key-value pairs incorrectly. This guide will guide you through the issue and provide a solution that not only fixes it but also explains the reasoning behind it.
The Problem: The TypeError Explained
This error typically occurs when trying to concatenate a string with a tuple. Consider the following example of a Python dictionary named webuser:
[[See Video to Reveal this Text or Code Snippet]]
If you try to loop through this dictionary using the following code:
[[See Video to Reveal this Text or Code Snippet]]
The Solution: Correcting the Loop
To resolve this issue, you need to unpack the tuple returned by items() so that each value is accessible as a separate variable. You can do this by modifying your loop as follows:
[[See Video to Reveal this Text or Code Snippet]]
Breakdown of the Solution
Unpacking the Tuple: By using two variables, key and value, you can unpack the tuple directly in the loop. The first element of the tuple will be assigned to key, and the second will go to value.
Concatenating Strings: Both key and value are strings, allowing you to safely concatenate them using the + operator without encountering a TypeError.
Key Takeaways
Use .items() Method: Always remember that when you call the .items() method on a dictionary, it returns tuples of key-value pairs. Make sure to unpack them correctly.
Debugging Tip: When debugging, print the variables within the loop to better understand how data is being processed.
The Importance of Understanding Data Types
Having a firm understanding of data types in Python can significantly reduce the number of runtime errors. In this case, knowing the difference between a string and a tuple is crucial to writing error-free code.
By being aware of how dictionary iteration works in combination with data types, you can prevent similar issues in your own projects in the future.
Conclusion
Understanding the mechanics behind Python dictionaries and how to iterate through them effectively is essential for any programmer. The TypeError issue we've discussed is a prime example of why unpacking tuples is necessary. With this knowledge, you should be well on your way to writing clear and effective Python code without pesky errors lurking behind your loops.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Type error: can only concatenate str (not "tuple") to str when looping through dictionary
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding and Fixing the TypeError in Python: Concatenating Strings with Tuples
When working with Python dictionaries, you might encounter a common error that begs an understanding of how to properly handle data types. One such error is the dreaded TypeError: can only concatenate str (not "tuple") to str, which typically arises when attempting to loop through a dictionary’s key-value pairs incorrectly. This guide will guide you through the issue and provide a solution that not only fixes it but also explains the reasoning behind it.
The Problem: The TypeError Explained
This error typically occurs when trying to concatenate a string with a tuple. Consider the following example of a Python dictionary named webuser:
[[See Video to Reveal this Text or Code Snippet]]
If you try to loop through this dictionary using the following code:
[[See Video to Reveal this Text or Code Snippet]]
The Solution: Correcting the Loop
To resolve this issue, you need to unpack the tuple returned by items() so that each value is accessible as a separate variable. You can do this by modifying your loop as follows:
[[See Video to Reveal this Text or Code Snippet]]
Breakdown of the Solution
Unpacking the Tuple: By using two variables, key and value, you can unpack the tuple directly in the loop. The first element of the tuple will be assigned to key, and the second will go to value.
Concatenating Strings: Both key and value are strings, allowing you to safely concatenate them using the + operator without encountering a TypeError.
Key Takeaways
Use .items() Method: Always remember that when you call the .items() method on a dictionary, it returns tuples of key-value pairs. Make sure to unpack them correctly.
Debugging Tip: When debugging, print the variables within the loop to better understand how data is being processed.
The Importance of Understanding Data Types
Having a firm understanding of data types in Python can significantly reduce the number of runtime errors. In this case, knowing the difference between a string and a tuple is crucial to writing error-free code.
By being aware of how dictionary iteration works in combination with data types, you can prevent similar issues in your own projects in the future.
Conclusion
Understanding the mechanics behind Python dictionaries and how to iterate through them effectively is essential for any programmer. The TypeError issue we've discussed is a prime example of why unpacking tuples is necessary. With this knowledge, you should be well on your way to writing clear and effective Python code without pesky errors lurking behind your loops.