filmov
tv
Using Vectorized Lookbacks with Two Numpy Arrays
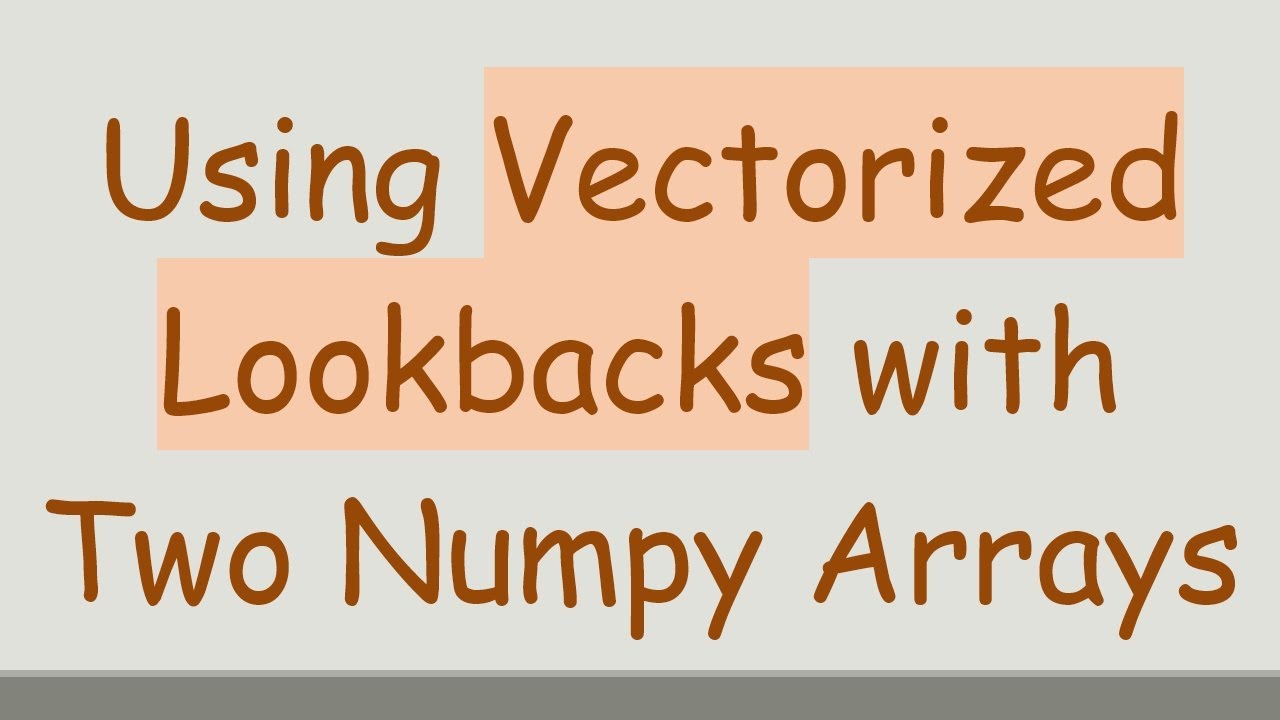
Показать описание
Learn how to efficiently implement vectorized lookbacks with two Numpy arrays to handle complex data processing tasks with ease.
---
Visit these links for original content and any more details, such as alternate solutions, comments, revision history etc. For example, the original title of the Question was: Vecotorized Lookback with Two Numpy Arrays
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Mastering Vectorized Lookbacks with Two Numpy Arrays
When working with large datasets in Python, especially in data analysis or machine learning, efficiency becomes paramount. One common challenge is implementing a lookback function that checks previous values based on specific criteria. In this article, we will focus on achieving this through vectorization using the NumPy library.
The Problem Statement
Suppose you have two NumPy arrays: first_arr and second_arr. The task is to loop through each element in first_arr and determine if any of the previous four values contain three or more values from second_arr. If this condition is met, the current value in first_arr should be added to a result array.
Understanding the Requirements
Lookback Limit: You need to check the previous four values (if available). If there are fewer than four values, check as many as are present.
Condition for Inclusion: A value in first_arr is included in the result if it meets the specified condition of containing at least three elements from second_arr.
Efficiency: The size of the actual arrays can be considerably larger, necessitating a vectorized solution rather than a standard loop.
Example of the Problem
To illustrate the problem, consider the following examples:
Example One
[[See Video to Reveal this Text or Code Snippet]]
Example Two
[[See Video to Reveal this Text or Code Snippet]]
The Solution: A Vectorized Approach
To tackle this problem efficiently, we can utilize NumPy's powerful array operations. Below is a vectorized function that fulfills the requirements:
[[See Video to Reveal this Text or Code Snippet]]
Breaking Down the Function
Initialization:
Creating a Sliding Window:
Counting Matches:
The sum operation counts how many True values are present in each window and checks if they meet the condition.
Returning Results:
Finally, values from first_arr that meet the criteria are returned in a new NumPy array.
Practical Examples of the Solution
Let’s see how our function performs with the provided examples:
Running Example One
[[See Video to Reveal this Text or Code Snippet]]
Running Example Two
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Vectorizing operations in Python, especially using the NumPy library, can greatly enhance the efficiency of data handling tasks. The sliding_window_contains function we outlined is a powerful way to perform lookback checks between two arrays, ensuring you can scale your data analysis without sacrificing performance.
By implementing this approach, you can manage larger datasets more effectively while maintaining clarity and simplicity in your code. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, comments, revision history etc. For example, the original title of the Question was: Vecotorized Lookback with Two Numpy Arrays
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Mastering Vectorized Lookbacks with Two Numpy Arrays
When working with large datasets in Python, especially in data analysis or machine learning, efficiency becomes paramount. One common challenge is implementing a lookback function that checks previous values based on specific criteria. In this article, we will focus on achieving this through vectorization using the NumPy library.
The Problem Statement
Suppose you have two NumPy arrays: first_arr and second_arr. The task is to loop through each element in first_arr and determine if any of the previous four values contain three or more values from second_arr. If this condition is met, the current value in first_arr should be added to a result array.
Understanding the Requirements
Lookback Limit: You need to check the previous four values (if available). If there are fewer than four values, check as many as are present.
Condition for Inclusion: A value in first_arr is included in the result if it meets the specified condition of containing at least three elements from second_arr.
Efficiency: The size of the actual arrays can be considerably larger, necessitating a vectorized solution rather than a standard loop.
Example of the Problem
To illustrate the problem, consider the following examples:
Example One
[[See Video to Reveal this Text or Code Snippet]]
Example Two
[[See Video to Reveal this Text or Code Snippet]]
The Solution: A Vectorized Approach
To tackle this problem efficiently, we can utilize NumPy's powerful array operations. Below is a vectorized function that fulfills the requirements:
[[See Video to Reveal this Text or Code Snippet]]
Breaking Down the Function
Initialization:
Creating a Sliding Window:
Counting Matches:
The sum operation counts how many True values are present in each window and checks if they meet the condition.
Returning Results:
Finally, values from first_arr that meet the criteria are returned in a new NumPy array.
Practical Examples of the Solution
Let’s see how our function performs with the provided examples:
Running Example One
[[See Video to Reveal this Text or Code Snippet]]
Running Example Two
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Vectorizing operations in Python, especially using the NumPy library, can greatly enhance the efficiency of data handling tasks. The sliding_window_contains function we outlined is a powerful way to perform lookback checks between two arrays, ensuring you can scale your data analysis without sacrificing performance.
By implementing this approach, you can manage larger datasets more effectively while maintaining clarity and simplicity in your code. Happy coding!