filmov
tv
Mastering List Transformations: How to Convert List to Integers in Python
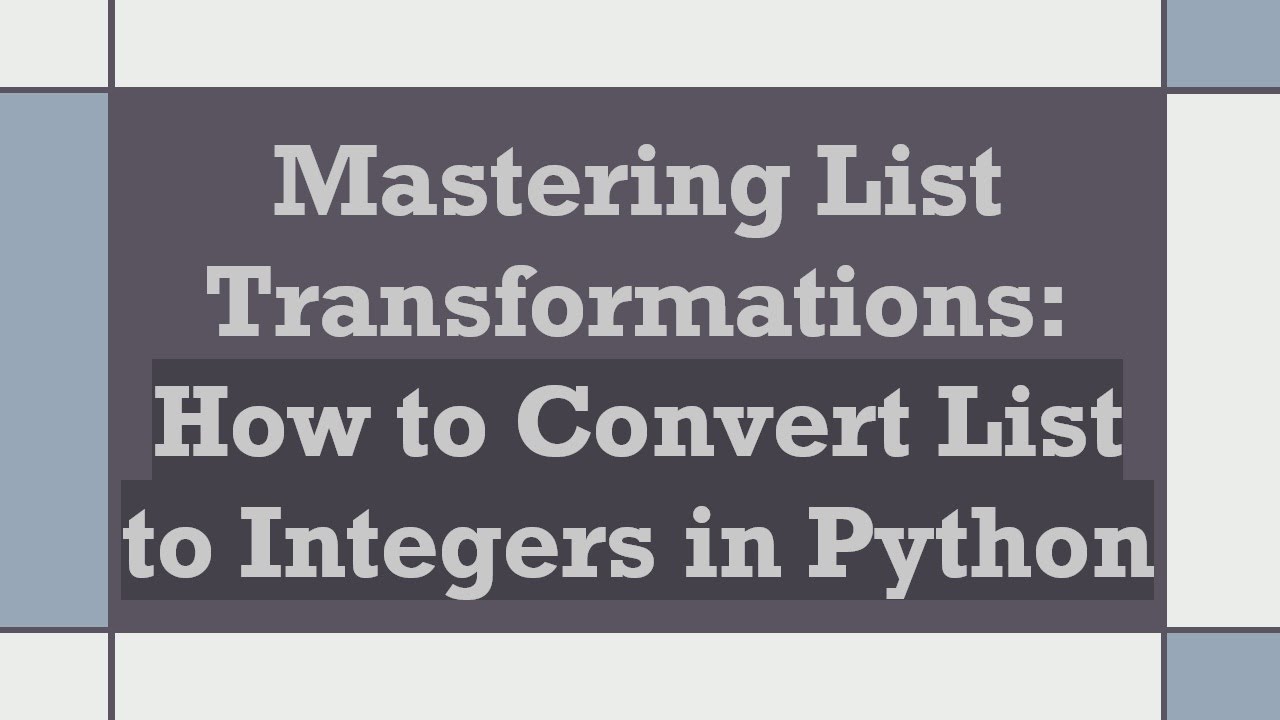
Показать описание
Summary: Learn how to efficiently convert lists to integers in Python with this comprehensive guide, perfect for both beginners and seasoned programmers.
---
Mastering List Transformations: How to Convert List to Integers in Python
In Python programming, lists are an incredibly versatile and widely used data structure. However, there are often scenarios where you'll need to convert a list of items into integers. Understanding the various ways to perform this transformation can be an essential skill in your Python toolbox. Whether you need to prepare data for computation, simplify data manipulation, or ensure consistency in data types, this guide will cover everything you need.
Converting a List of String Numbers to Integers
The most straightforward case is where you have a list of numbers in string format and you want to convert them to actual integers. Here's how you can do it:
[[See Video to Reveal this Text or Code Snippet]]
In this example, the map() function is used to apply the int function to each element of the string_numbers list. The list() function then converts the map object back into a list.
Turning Mixed Data Types into Integers
If your list contains mixed data types, such as strings and numbers, you'll need to filter and convert only the parts that can be turned into integers. You can handle this with a combination of list comprehensions and conditionals:
[[See Video to Reveal this Text or Code Snippet]]
Here, we use a list comprehension to check if each item is either a string that represents a digit or an integer. If these conditions are met, the item is converted to an integer.
Converting a List of Float Numbers to Integers
If your list contains floating-point numbers, converting them directly to integers will truncate their decimal values. This can be achieved using:
[[See Video to Reveal this Text or Code Snippet]]
This technique uses a list comprehension to iterate through the float_numbers list, applying the int function to each element, which truncates the decimal part.
How to Handle Advanced Structures
In some cases, you might be dealing with more complex structures like nested lists or lists of tuples. You would then need a more sophisticated approach:
[[See Video to Reveal this Text or Code Snippet]]
For lists of tuples:
[[See Video to Reveal this Text or Code Snippet]]
In both examples, nested list comprehensions are used to iterate and convert each element at the respective level of nesting.
Conclusion
Whether you're dealing with simple lists, mixed data types, floating-point numbers, or more complex structures, knowing how to turn a list to integers in Python can be a real game-changer. These techniques offer a robust foundation for your data manipulation needs. Happy coding!
---
Mastering List Transformations: How to Convert List to Integers in Python
In Python programming, lists are an incredibly versatile and widely used data structure. However, there are often scenarios where you'll need to convert a list of items into integers. Understanding the various ways to perform this transformation can be an essential skill in your Python toolbox. Whether you need to prepare data for computation, simplify data manipulation, or ensure consistency in data types, this guide will cover everything you need.
Converting a List of String Numbers to Integers
The most straightforward case is where you have a list of numbers in string format and you want to convert them to actual integers. Here's how you can do it:
[[See Video to Reveal this Text or Code Snippet]]
In this example, the map() function is used to apply the int function to each element of the string_numbers list. The list() function then converts the map object back into a list.
Turning Mixed Data Types into Integers
If your list contains mixed data types, such as strings and numbers, you'll need to filter and convert only the parts that can be turned into integers. You can handle this with a combination of list comprehensions and conditionals:
[[See Video to Reveal this Text or Code Snippet]]
Here, we use a list comprehension to check if each item is either a string that represents a digit or an integer. If these conditions are met, the item is converted to an integer.
Converting a List of Float Numbers to Integers
If your list contains floating-point numbers, converting them directly to integers will truncate their decimal values. This can be achieved using:
[[See Video to Reveal this Text or Code Snippet]]
This technique uses a list comprehension to iterate through the float_numbers list, applying the int function to each element, which truncates the decimal part.
How to Handle Advanced Structures
In some cases, you might be dealing with more complex structures like nested lists or lists of tuples. You would then need a more sophisticated approach:
[[See Video to Reveal this Text or Code Snippet]]
For lists of tuples:
[[See Video to Reveal this Text or Code Snippet]]
In both examples, nested list comprehensions are used to iterate and convert each element at the respective level of nesting.
Conclusion
Whether you're dealing with simple lists, mixed data types, floating-point numbers, or more complex structures, knowing how to turn a list to integers in Python can be a real game-changer. These techniques offer a robust foundation for your data manipulation needs. Happy coding!