filmov
tv
C++ Programming SS21 - Week 0
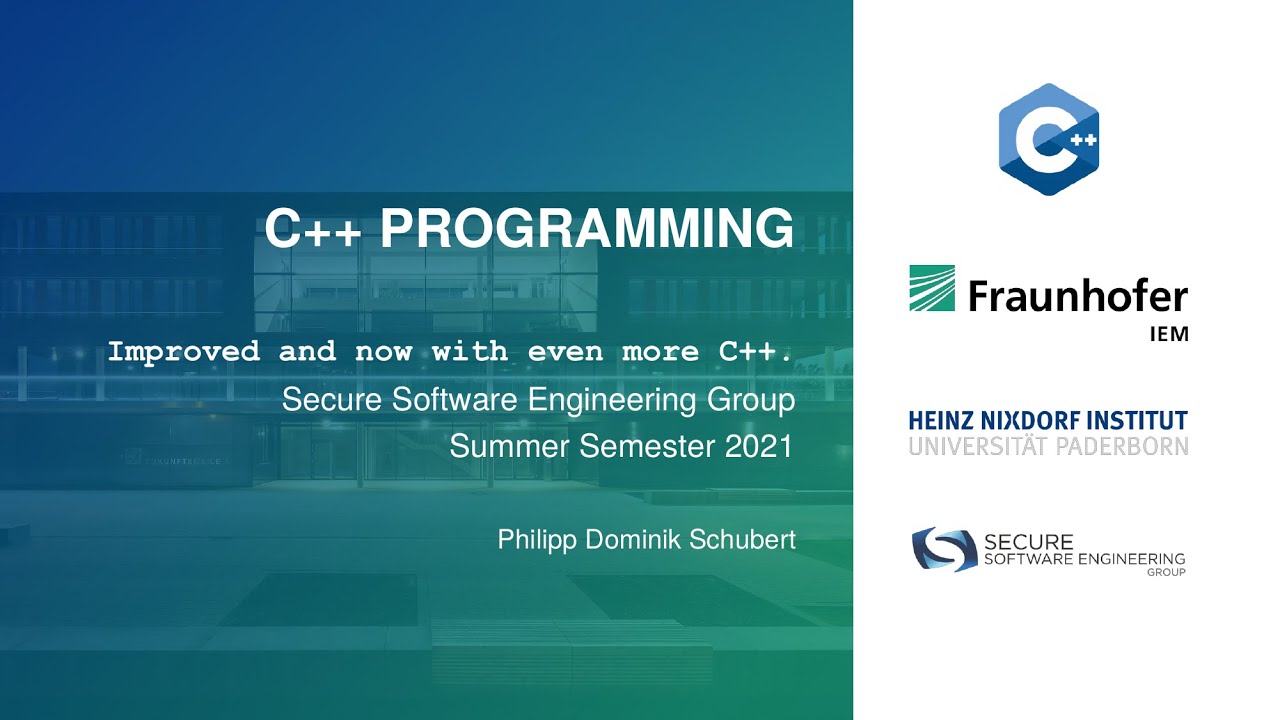
Показать описание
This is a recording of the "C++ Programming" course (summer term 2021) taught by Philipp Schubert (/Philipp Dominik Schubert) at Paderborn University.
The lecture covers the following topics:
========
-Organizational matter
-Course outline: now with even more C++
-History of C++
-C++ compilers
-A "Hello, World!" program
-Setting up a development environment
-Basic terms & concepts
========
-More on data types
-Expressions
-const & constexpr
-Statements
-Control flow
========
-Functions
-std::string
-std::vector
-Containers
-Pointer and reference types
========
-Unions
-Enumerations
-Structures
-Classes
-Organizing C++ projects
-More on C/C++ compiler toolchains
-Namespaces
========
-Operator overloading
-Memory
-Dynamic memory allocation
-Copy constructor / assign (a real-world example)
-Move constructor / assign (a real-world example)
-Notes on dynamic memory allocation
========
-Error handling
-Return codes
-Assertions
-Exceptions
-Function pointers
-std::function
========
-C/C++ preprocessor
-Software product lines
-Templates
-Variadic function arguments
-Functors
-Lambda functions
========
-Template metaprogramming
-Variadic template arguments
-Smart pointers
========
-Static memory
-Object oriented programming I
========
-Object oriented programming II
-Type conversion / type casting
-File IO
========
-Libraries
-Iterators
========
-High performance computing
-High performance computing in C++
-Example: matrix multiplication
-Important compiler flags
-How to help the compiler
-What the compiler can do for you
========
-Cyber attacks
-Program analysis
-Why do we need program analysis?
-Static program analysis
-Designing code analysis (DECA)
-Software Engineering Group: jobs and theses
========
-Introduction to the final project
-Miscellaneous and advanced topics
-What next
The lecture covers the following topics:
========
-Organizational matter
-Course outline: now with even more C++
-History of C++
-C++ compilers
-A "Hello, World!" program
-Setting up a development environment
-Basic terms & concepts
========
-More on data types
-Expressions
-const & constexpr
-Statements
-Control flow
========
-Functions
-std::string
-std::vector
-Containers
-Pointer and reference types
========
-Unions
-Enumerations
-Structures
-Classes
-Organizing C++ projects
-More on C/C++ compiler toolchains
-Namespaces
========
-Operator overloading
-Memory
-Dynamic memory allocation
-Copy constructor / assign (a real-world example)
-Move constructor / assign (a real-world example)
-Notes on dynamic memory allocation
========
-Error handling
-Return codes
-Assertions
-Exceptions
-Function pointers
-std::function
========
-C/C++ preprocessor
-Software product lines
-Templates
-Variadic function arguments
-Functors
-Lambda functions
========
-Template metaprogramming
-Variadic template arguments
-Smart pointers
========
-Static memory
-Object oriented programming I
========
-Object oriented programming II
-Type conversion / type casting
-File IO
========
-Libraries
-Iterators
========
-High performance computing
-High performance computing in C++
-Example: matrix multiplication
-Important compiler flags
-How to help the compiler
-What the compiler can do for you
========
-Cyber attacks
-Program analysis
-Why do we need program analysis?
-Static program analysis
-Designing code analysis (DECA)
-Software Engineering Group: jobs and theses
========
-Introduction to the final project
-Miscellaneous and advanced topics
-What next
Комментарии