filmov
tv
Boost Performance with .NET Multithreading for File Copying in WinForms
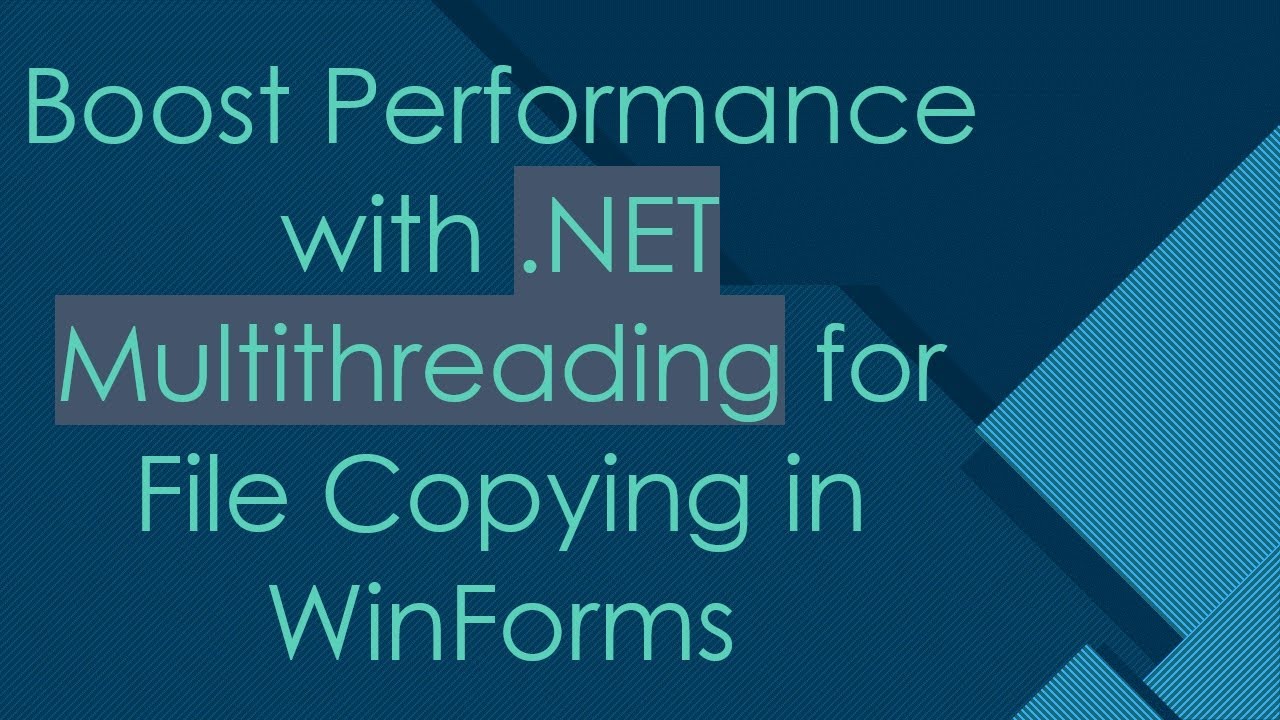
Показать описание
Summary: Learn how to employ multithreading in a .NET WinForms application to enhance file copying without freezing the UI, employing advanced techniques.
---
When building a WinForms application in .NET, one common task that can significantly benefit from multithreading is file copying. By employing multithreading, not only can you ensure a smooth user experience, but you can also significantly reduce the time taken for file operations. This post will guide you on how to implement multithreading for file copying in a WinForms application without locking the UI, aimed at intermediate to advanced .NET developers.
Understanding .NET Multithreading
To start, it’s important to understand the basics of multithreading in .NET. The primary goal is to allow multiple operations to run concurrently. This is particularly beneficial in a WinForms app where a long-running operation like file copying can otherwise cause the UI to become unresponsive. By using threads, you can perform these operations in the background, keeping the UI fluid and responsive.
Implementing Multithreading for File Copying
In a WinForms app, the BackgroundWorker is a common choice for implementing multithreading. It provides a straightforward way to run an operation on a separate, dedicated thread.
Here’s a high-level approach to implement file copying using BackgroundWorker:
Add a BackgroundWorker component to your form from the toolbox.
Handle the DoWork event, where you'll put the logic for file copying. This event runs on a separate thread.
[[See Video to Reveal this Text or Code Snippet]]
Start the BackgroundWorker when you trigger the file copy operation, such as a button click.
[[See Video to Reveal this Text or Code Snippet]]
Optionally handle the ProgressChanged and RunWorkerCompleted events for updating the UI during and after the file operation. These events ensure that UI updates are marshaled back to the main thread safely.
[[See Video to Reveal this Text or Code Snippet]]
Advanced Techniques
For more complex operations or finer control over threading, you might explore the Task Parallel Library (TPL), which is a more modern and powerful alternative to BackgroundWorker.
Here's a basic example using Task:
[[See Video to Reveal this Text or Code Snippet]]
This approach leverages asynchronous programming, making it more suitable for scenarios needing integration with other asynchronous workflows.
Conclusion
Implementing multithreading for file copying can greatly enhance the performance and responsiveness of a .NET WinForms application. By using techniques like BackgroundWorker or the Task Parallel Library, you can keep your application responsive while performing intensive tasks in the background. As always, consider the complexity of the operations and choose the strategy that best suits your application's requirements.
Whether you’re developing a simple tool or a complex application, understanding and utilizing multithreading effectively can give your projects an essential performance boost.
---
When building a WinForms application in .NET, one common task that can significantly benefit from multithreading is file copying. By employing multithreading, not only can you ensure a smooth user experience, but you can also significantly reduce the time taken for file operations. This post will guide you on how to implement multithreading for file copying in a WinForms application without locking the UI, aimed at intermediate to advanced .NET developers.
Understanding .NET Multithreading
To start, it’s important to understand the basics of multithreading in .NET. The primary goal is to allow multiple operations to run concurrently. This is particularly beneficial in a WinForms app where a long-running operation like file copying can otherwise cause the UI to become unresponsive. By using threads, you can perform these operations in the background, keeping the UI fluid and responsive.
Implementing Multithreading for File Copying
In a WinForms app, the BackgroundWorker is a common choice for implementing multithreading. It provides a straightforward way to run an operation on a separate, dedicated thread.
Here’s a high-level approach to implement file copying using BackgroundWorker:
Add a BackgroundWorker component to your form from the toolbox.
Handle the DoWork event, where you'll put the logic for file copying. This event runs on a separate thread.
[[See Video to Reveal this Text or Code Snippet]]
Start the BackgroundWorker when you trigger the file copy operation, such as a button click.
[[See Video to Reveal this Text or Code Snippet]]
Optionally handle the ProgressChanged and RunWorkerCompleted events for updating the UI during and after the file operation. These events ensure that UI updates are marshaled back to the main thread safely.
[[See Video to Reveal this Text or Code Snippet]]
Advanced Techniques
For more complex operations or finer control over threading, you might explore the Task Parallel Library (TPL), which is a more modern and powerful alternative to BackgroundWorker.
Here's a basic example using Task:
[[See Video to Reveal this Text or Code Snippet]]
This approach leverages asynchronous programming, making it more suitable for scenarios needing integration with other asynchronous workflows.
Conclusion
Implementing multithreading for file copying can greatly enhance the performance and responsiveness of a .NET WinForms application. By using techniques like BackgroundWorker or the Task Parallel Library, you can keep your application responsive while performing intensive tasks in the background. As always, consider the complexity of the operations and choose the strategy that best suits your application's requirements.
Whether you’re developing a simple tool or a complex application, understanding and utilizing multithreading effectively can give your projects an essential performance boost.