filmov
tv
Troubleshooting the Object is not callable Error in Python's Windpower Class
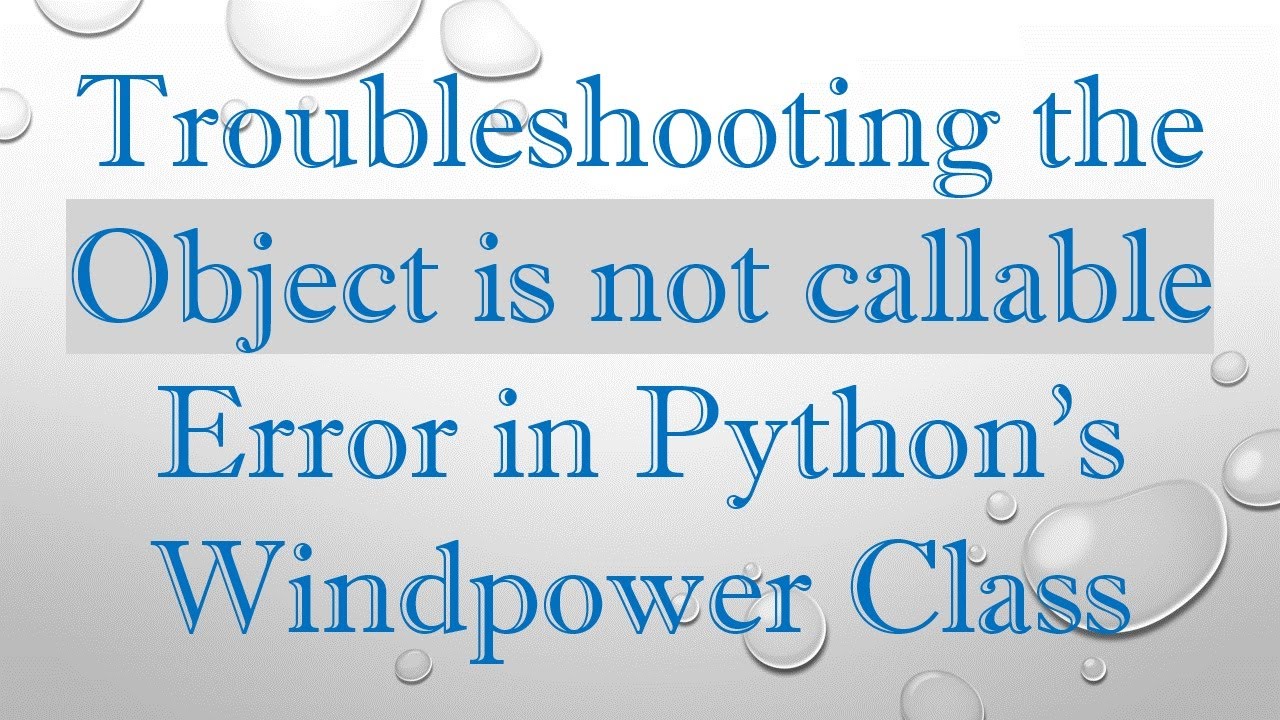
Показать описание
Learn why you encounter the "Object is not callable" error when using the Windpower class in your Python code, and how to fix it.
---
Disclaimer/Disclosure - Portions of this content were created using Generative AI tools, which may result in inaccuracies or misleading information in the video. Please keep this in mind before making any decisions or taking any actions based on the content. If you have any concerns, don't hesitate to leave a comment. Thanks.
---
Troubleshooting the Object is not callable Error in Python's Windpower Class
If you've encountered the "Object is not callable" error while working with the Windpower class in Python, you're not alone. This is a common error that can stump even seasoned developers. Let's dive into why this happens and how you can resolve it.
Understanding the Error
In Python, this error often occurs when you try to call an object that isn't a function or method. For instance, if you define an object and mistakenly attempt to 'call' it like a function, Python will raise a TypeError and state that the "Object is not callable."
The Windpower Class
Consider a scenario where you're working with a class named Windpower:
[[See Video to Reveal this Text or Code Snippet]]
If you later try to execute plant() instead of accessing its methods or attributes, Python will raise the error:
[[See Video to Reveal this Text or Code Snippet]]
Why This Happens
The error occurs because plant is an instance of the Windpower class, not a function. Python expects callable objects (functions or methods) to have a __call__ method defined internally. Classes, by default, do not have this method, so trying to call an instance of the class like a function will raise a TypeError.
How to Fix It
To fix this error, review your code and ensure that you're not mistakenly trying to call an instance of a class. Instead, you should be calling methods of the instance. Here’s how to properly use the Windpower instance:
[[See Video to Reveal this Text or Code Snippet]]
If your intention is to make the class instance callable, you should implement the __call__ method in your class:
[[See Video to Reveal this Text or Code Snippet]]
By following these tips, you can effectively troubleshoot and resolve the "Object is not callable" error in your code involving the Windpower class.
Conclusion
The "Object is not callable" error is a common but fixable issue. By understanding why it happens and how to avoid it, you can write more robust Python code. Remember to double-check if you're trying to call an instance instead of its methods, and consider implementing the __call__ method if you need class instances to be callable.
Happy coding!
---
Disclaimer/Disclosure - Portions of this content were created using Generative AI tools, which may result in inaccuracies or misleading information in the video. Please keep this in mind before making any decisions or taking any actions based on the content. If you have any concerns, don't hesitate to leave a comment. Thanks.
---
Troubleshooting the Object is not callable Error in Python's Windpower Class
If you've encountered the "Object is not callable" error while working with the Windpower class in Python, you're not alone. This is a common error that can stump even seasoned developers. Let's dive into why this happens and how you can resolve it.
Understanding the Error
In Python, this error often occurs when you try to call an object that isn't a function or method. For instance, if you define an object and mistakenly attempt to 'call' it like a function, Python will raise a TypeError and state that the "Object is not callable."
The Windpower Class
Consider a scenario where you're working with a class named Windpower:
[[See Video to Reveal this Text or Code Snippet]]
If you later try to execute plant() instead of accessing its methods or attributes, Python will raise the error:
[[See Video to Reveal this Text or Code Snippet]]
Why This Happens
The error occurs because plant is an instance of the Windpower class, not a function. Python expects callable objects (functions or methods) to have a __call__ method defined internally. Classes, by default, do not have this method, so trying to call an instance of the class like a function will raise a TypeError.
How to Fix It
To fix this error, review your code and ensure that you're not mistakenly trying to call an instance of a class. Instead, you should be calling methods of the instance. Here’s how to properly use the Windpower instance:
[[See Video to Reveal this Text or Code Snippet]]
If your intention is to make the class instance callable, you should implement the __call__ method in your class:
[[See Video to Reveal this Text or Code Snippet]]
By following these tips, you can effectively troubleshoot and resolve the "Object is not callable" error in your code involving the Windpower class.
Conclusion
The "Object is not callable" error is a common but fixable issue. By understanding why it happens and how to avoid it, you can write more robust Python code. Remember to double-check if you're trying to call an instance instead of its methods, and consider implementing the __call__ method if you need class instances to be callable.
Happy coding!