filmov
tv
How to Filter a JSON Response and Extract Required Data in React Native
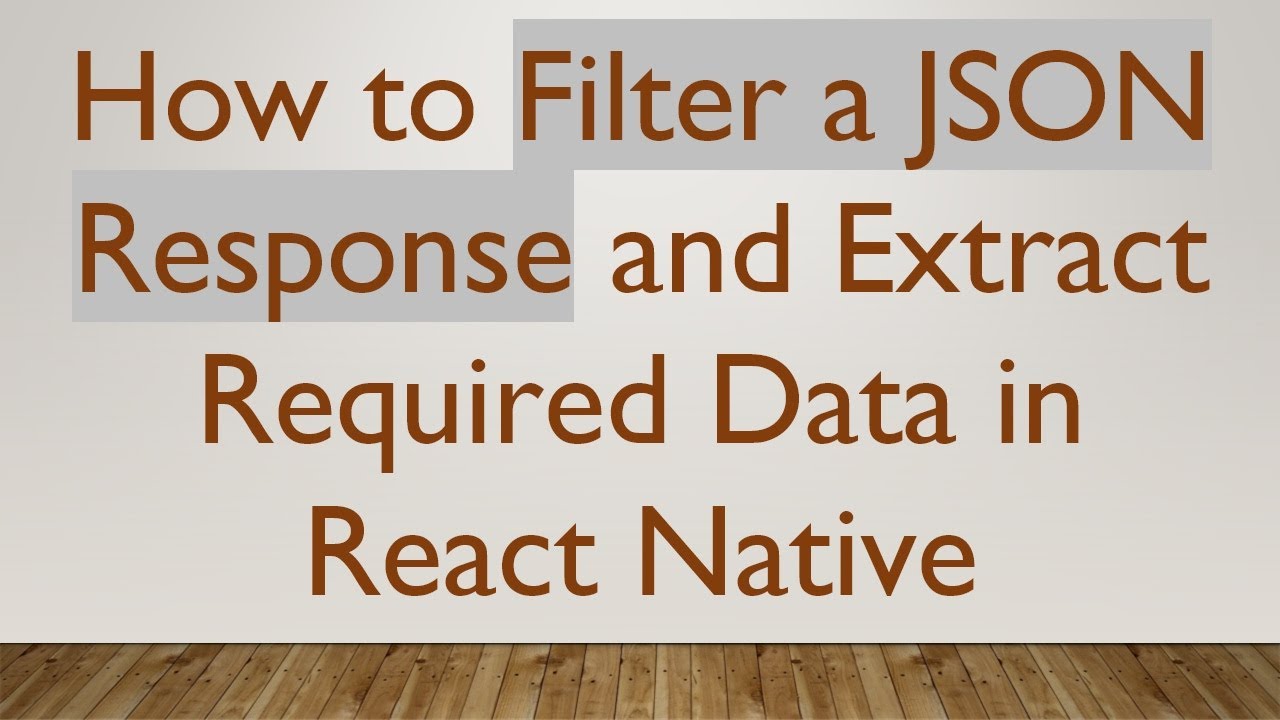
Показать описание
Learn how to efficiently filter a JSON response in React Native to retrieve specific data based on conditions. This guide will walk you through the process step-by-step.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to filter a JSON response and get needed data from it in react native?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Filter a JSON Response and Extract Required Data in React Native
When developing applications with React Native, you often work with JSON data obtained from APIs. A common requirement in these scenarios is to filter this data and extract only the necessary information. In this guide, we'll tackle a specific problem: how to filter a JSON response to get the tripDate when the voidType in tripLineItemDetails is zero.
Understanding the JSON Structure
Let’s first examine the JSON response we are working with:
[[See Video to Reveal this Text or Code Snippet]]
tripDate: Represents the date of the trip.
totalFare: The cost associated with the trip.
tripLineItemDetails: An array where each object can have a voidType.
Our goal here is to return an array containing only those entries where at least one voidType in the tripLineItemDetails equals zero.
Solution: Filtering the Data
Step 1: Set Up the Filter Logic
We can easily achieve this using JavaScript array methods. We'll use the filter method along with the find method to check for the condition of voidType being zero. Here’s the complete code snippet:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Code
response: An array of objects containing trips.
filter(): This method creates a new array with elements that pass the test implemented by the provided function.
find(): It checks each tripLineItemDetails array to see if there exists any object where voidType is 0.
Step 2: Expected Result
The resulting filtered data will contain only those entries that have at least one voidType of zero:
[[See Video to Reveal this Text or Code Snippet]]
Ensuring No Extra Data
If you wish to avoid getting any voidType: 1 along with the results, you can utilize more complex logic like nesting loops or further checking the conditions while filtering. Here’s a simple check to prevent including any items with voidType of 1:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
We’ve successfully learned how to filter a JSON response in React Native to extract specific data based on certain conditions. By using JavaScript’s array methods like filter and find, we can efficiently parse through JSON objects and obtain the required information. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to filter a JSON response and get needed data from it in react native?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Filter a JSON Response and Extract Required Data in React Native
When developing applications with React Native, you often work with JSON data obtained from APIs. A common requirement in these scenarios is to filter this data and extract only the necessary information. In this guide, we'll tackle a specific problem: how to filter a JSON response to get the tripDate when the voidType in tripLineItemDetails is zero.
Understanding the JSON Structure
Let’s first examine the JSON response we are working with:
[[See Video to Reveal this Text or Code Snippet]]
tripDate: Represents the date of the trip.
totalFare: The cost associated with the trip.
tripLineItemDetails: An array where each object can have a voidType.
Our goal here is to return an array containing only those entries where at least one voidType in the tripLineItemDetails equals zero.
Solution: Filtering the Data
Step 1: Set Up the Filter Logic
We can easily achieve this using JavaScript array methods. We'll use the filter method along with the find method to check for the condition of voidType being zero. Here’s the complete code snippet:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Code
response: An array of objects containing trips.
filter(): This method creates a new array with elements that pass the test implemented by the provided function.
find(): It checks each tripLineItemDetails array to see if there exists any object where voidType is 0.
Step 2: Expected Result
The resulting filtered data will contain only those entries that have at least one voidType of zero:
[[See Video to Reveal this Text or Code Snippet]]
Ensuring No Extra Data
If you wish to avoid getting any voidType: 1 along with the results, you can utilize more complex logic like nesting loops or further checking the conditions while filtering. Here’s a simple check to prevent including any items with voidType of 1:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
We’ve successfully learned how to filter a JSON response in React Native to extract specific data based on certain conditions. By using JavaScript’s array methods like filter and find, we can efficiently parse through JSON objects and obtain the required information. Happy coding!