filmov
tv
How to Push an Item to Nested Object in JavaScript State Management
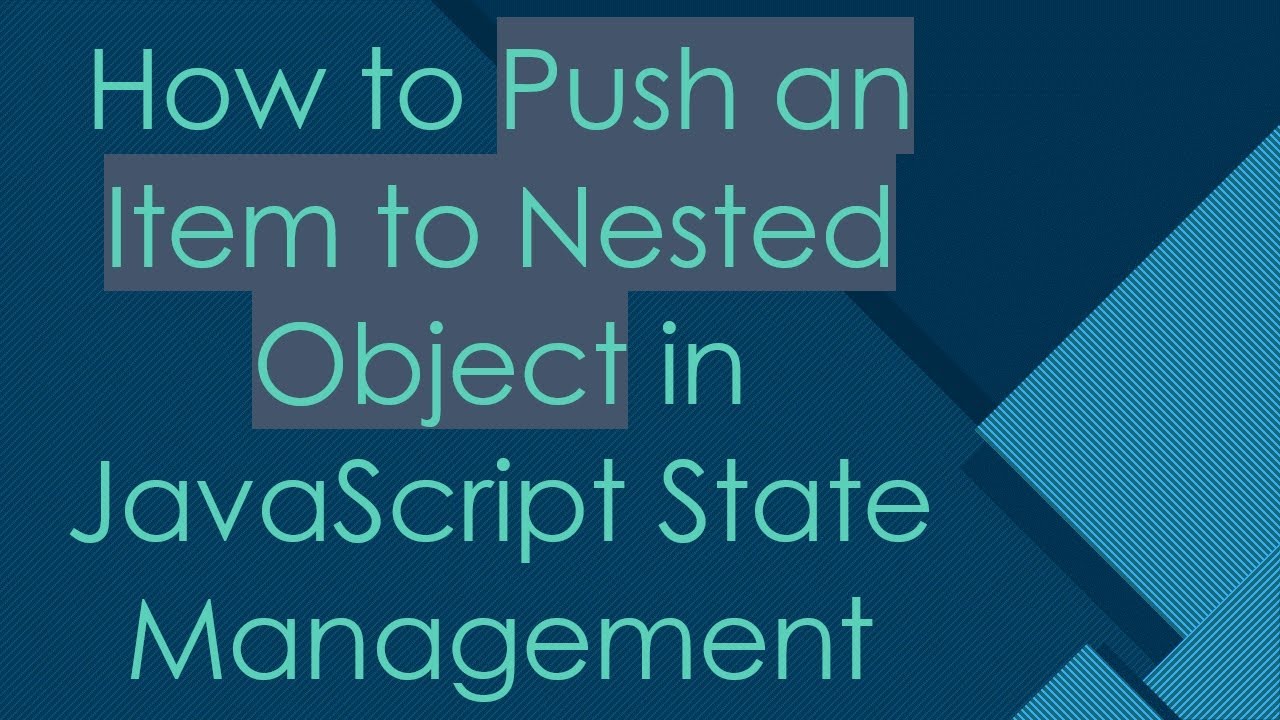
Показать описание
Learn how to effectively manage state in JavaScript by adding items to nested objects without direct modifications. Examples provided for React and TypeScript.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How i push an item to nested object?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Push an Item to Nested Object in JavaScript State Management
Managing state in JavaScript applications, particularly those built with React, often involves working with nested objects. This can be tricky when it comes time to modify these objects—particularly if you want to add new items or update existing values. In this post, we will tackle a common problem: how to push a value into a nested object without directly modifying the original state.
Understanding the Problem
You may find yourself in a situation where you have an object structure defined with TypeScript, and you want to add new items to a nested array within that object. This can be especially challenging when you need to maintain immutability, which is a critical aspect of state management in React.
Example Structure
Consider the following structure that defines initialValues:
[[See Video to Reveal this Text or Code Snippet]]
You may want to access the role array and push a new object into it. For example, you might want to add a role with init as "test" and lang as "TR".
Challenges Faced
If you try to use the map or filter methods directly, you might end up modifying the original array instead of returning a new version that includes your changes. This is why understanding the immutable approach to state updates is critical.
Step-By-Step Solution
Let's break down how to effectively add a new role to the role array in our state without mutating the existing data:
Using State Updates Wisely
Update Function: Use the setInitialValues function to receive the previous state (prevValues).
Map Through Values: Map through each item to find the correct object you want to update based on a rule (like matching addPrivs value).
Clone the Object: When you find the correct item, return a new object that combines the old attributes with the new role being added.
Here’s the code demonstrating this approach:
[[See Video to Reveal this Text or Code Snippet]]
Code Breakdown
setInitialValues: This function updates your state. It uses a callback to work with the previous state.
map: Iterates over each item in the prevValues array.
Condition Check: Here, we check if the addPrivs matches a certain value (in this case, "something"). If it does, we create a new object.
Object Spread Syntax (...): This is crucial for ensuring immutability. It allows you to copy the existing properties and add a new role with additional items.
Conclusion
By following the above approach, you can efficiently push new items to nested objects in your React state while adhering to the principles of immutability. This not only helps maintain clean code but also ensures predictable state management in your applications. If you're working with state in React and TypeScript, understanding these concepts will greatly improve the robustness of your code.
Make sure to practice these techniques, and you'll become proficient in managing complex state structures in no time!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How i push an item to nested object?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Push an Item to Nested Object in JavaScript State Management
Managing state in JavaScript applications, particularly those built with React, often involves working with nested objects. This can be tricky when it comes time to modify these objects—particularly if you want to add new items or update existing values. In this post, we will tackle a common problem: how to push a value into a nested object without directly modifying the original state.
Understanding the Problem
You may find yourself in a situation where you have an object structure defined with TypeScript, and you want to add new items to a nested array within that object. This can be especially challenging when you need to maintain immutability, which is a critical aspect of state management in React.
Example Structure
Consider the following structure that defines initialValues:
[[See Video to Reveal this Text or Code Snippet]]
You may want to access the role array and push a new object into it. For example, you might want to add a role with init as "test" and lang as "TR".
Challenges Faced
If you try to use the map or filter methods directly, you might end up modifying the original array instead of returning a new version that includes your changes. This is why understanding the immutable approach to state updates is critical.
Step-By-Step Solution
Let's break down how to effectively add a new role to the role array in our state without mutating the existing data:
Using State Updates Wisely
Update Function: Use the setInitialValues function to receive the previous state (prevValues).
Map Through Values: Map through each item to find the correct object you want to update based on a rule (like matching addPrivs value).
Clone the Object: When you find the correct item, return a new object that combines the old attributes with the new role being added.
Here’s the code demonstrating this approach:
[[See Video to Reveal this Text or Code Snippet]]
Code Breakdown
setInitialValues: This function updates your state. It uses a callback to work with the previous state.
map: Iterates over each item in the prevValues array.
Condition Check: Here, we check if the addPrivs matches a certain value (in this case, "something"). If it does, we create a new object.
Object Spread Syntax (...): This is crucial for ensuring immutability. It allows you to copy the existing properties and add a new role with additional items.
Conclusion
By following the above approach, you can efficiently push new items to nested objects in your React state while adhering to the principles of immutability. This not only helps maintain clean code but also ensures predictable state management in your applications. If you're working with state in React and TypeScript, understanding these concepts will greatly improve the robustness of your code.
Make sure to practice these techniques, and you'll become proficient in managing complex state structures in no time!