filmov
tv
Javascript in 1 shot in Hindi | part 1
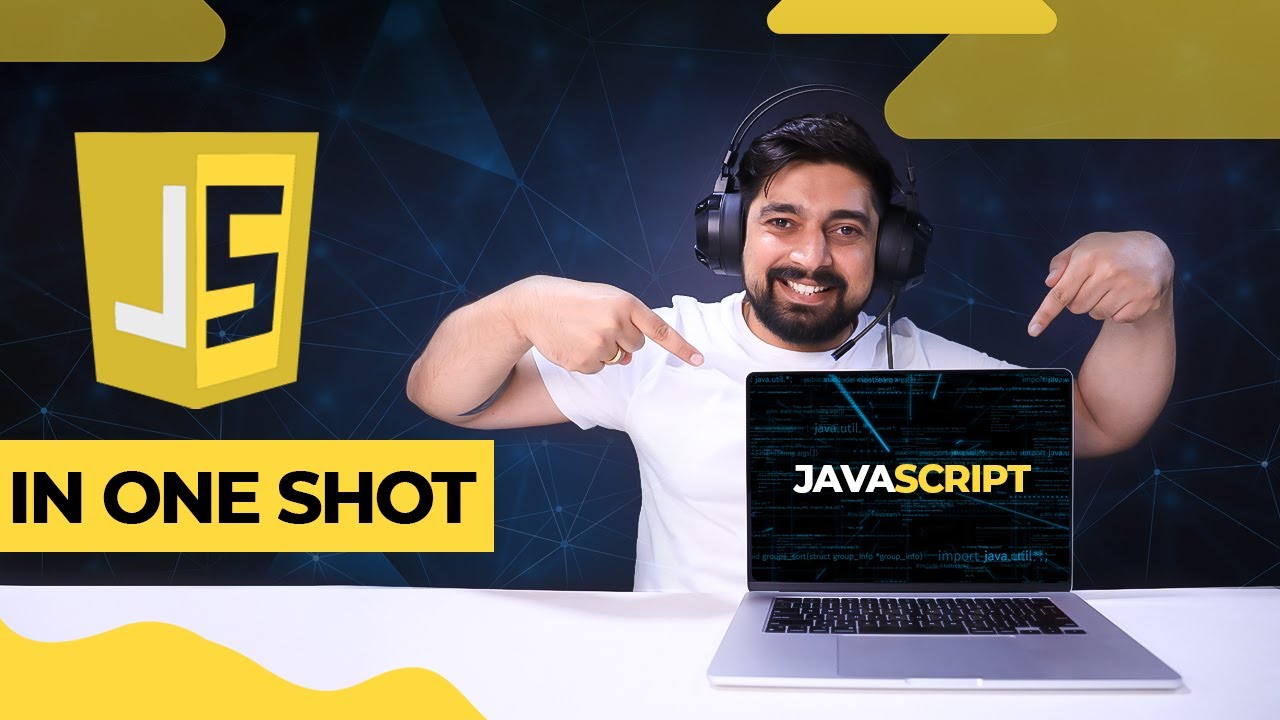
Показать описание
00:00:00 - Javascript for beginners
00:05:04 - Setting up environment
00:16:53 - Save and work on GitHub
00:27:14 - Let const var
00:43:54 - Datatypes and ECMA standards
01:01:55 - Datatyope conversion confusion
01:14:46 - Why string to number
01:29:46 - comparison of datatypes
01:38:38 - datatypes summary
01:56:40 - stack and heap memory
02:06:34 - String in javascript
02:29:17 - Number and maths
02:52:34 - date and time
03:10:47 - Array in javascript
03:29:42 - Array part 2
03:45:23 - Objects in depth
04:03:31 - Objects part 2
04:21:13 - Objects destructuring and JSON API
04:34:46 - Functions and parameters
04:53:59 - functions with objects
05:05:14 - Global and local scope
05:14:51 - Scope level and mini hoisting
05:29:47 - this and arrow function
05:48:16 - Immediately invoked function
05:55:33 - How does javascript works behind the scene
06:21:45 - Control flow in javascript
07:14:34 - for loop break and continue
07:39:05 - while do while loop
07:49:24 - High order array loops
08:23:35 - filter map and reduce
Sara code yaha milta h
Discord pe yaha paaye jaate h:
Instagram pe yaha paaye jaate h:
00:05:04 - Setting up environment
00:16:53 - Save and work on GitHub
00:27:14 - Let const var
00:43:54 - Datatypes and ECMA standards
01:01:55 - Datatyope conversion confusion
01:14:46 - Why string to number
01:29:46 - comparison of datatypes
01:38:38 - datatypes summary
01:56:40 - stack and heap memory
02:06:34 - String in javascript
02:29:17 - Number and maths
02:52:34 - date and time
03:10:47 - Array in javascript
03:29:42 - Array part 2
03:45:23 - Objects in depth
04:03:31 - Objects part 2
04:21:13 - Objects destructuring and JSON API
04:34:46 - Functions and parameters
04:53:59 - functions with objects
05:05:14 - Global and local scope
05:14:51 - Scope level and mini hoisting
05:29:47 - this and arrow function
05:48:16 - Immediately invoked function
05:55:33 - How does javascript works behind the scene
06:21:45 - Control flow in javascript
07:14:34 - for loop break and continue
07:39:05 - while do while loop
07:49:24 - High order array loops
08:23:35 - filter map and reduce
Sara code yaha milta h
Discord pe yaha paaye jaate h:
Instagram pe yaha paaye jaate h:
Комментарии